Within the realm of programming, the seamless conversion of binary numbers to their decimal counterparts is a fundamental skill that opens doors to a multitude of applications. This guide is your passport to unraveling the mysteries of binary-to-decimal conversion in Python, all without relying on built-in functions.
Join us on this journey as we explore the intricacies of binary and decimal representations and equip you with the knowledge to perform this conversion effortlessly.
The Essence of Binary-to-Decimal Conversion
Understanding Binary Representation
Binary is the cornerstone of computing, representing data in the form of zeros and ones. Each binary digit, or bit, holds a significant value in powers of two, enabling the representation of a wide range of data.
The Challenge of Decimal Conversion
Converting binary numbers to their decimal equivalents might seem daunting, especially when excluding built-in functions that provide instant solutions. However, this process unveils the mathematical intricacies that form the basis of modern computing.
The Step-by-Step Conversion Process
Step 1: Decomposing the Binary Number
Begin by breaking down the binary number into its individual digits. Start from the rightmost digit and assign powers of two to each bit, progressing from 2^0, 2^1, 2^2, and so forth.
Step 2: Calculating the Decimal Equivalent
Multiply each binary digit by its corresponding power of two and sum up the results. This cumulative sum yields the decimal equivalent of the binary number.
```python
binary_number = "101010"
decimal_equivalent = 0
for index, bit in enumerate(binary_number[::-1]):
decimal_equivalent += int(bit) * (2 ** index)
print(f"The decimal equivalent of {binary_number} is {decimal_equivalent}")
```
Practical Applications of Binary-to-Decimal Conversion
Data Representation and Manipulation
Binary-to-decimal conversion forms the foundation for data representation and manipulation in computers. From image processing to cryptography, the ability to seamlessly switch between these number systems is indispensable.
Networking and Protocol Handling
In networking and protocol communication, binary data is a common currency. Converting binary data to decimal aids in interpreting packet payloads, facilitating efficient data exchange between systems.
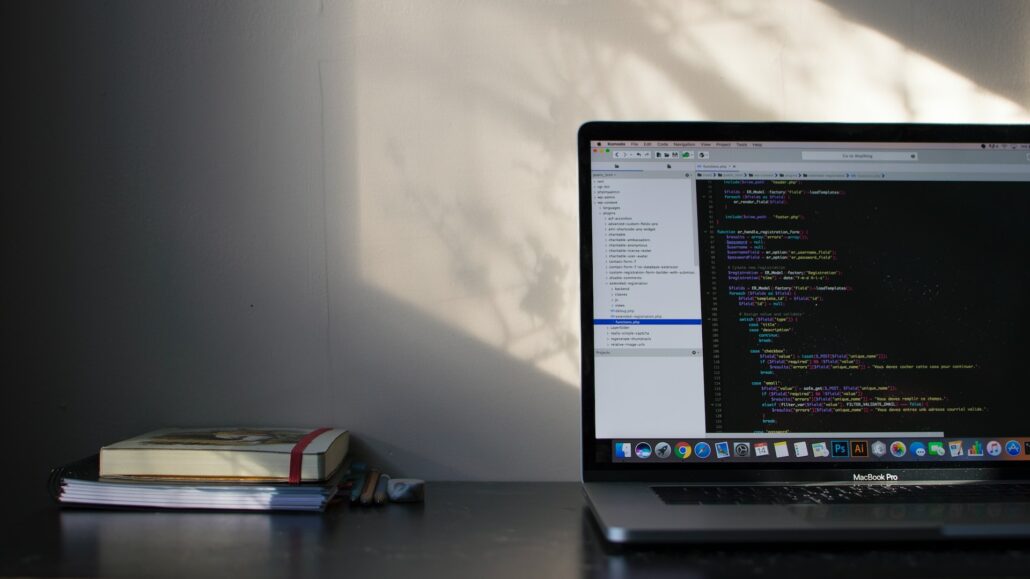
Conclusion
As we conclude this journey through binary-to-decimal conversion, you’ve emerged equipped with a skill that bridges the gap between fundamental number systems. By mastering this art without resorting to built-in functions, you’ve not only elevated your programming prowess but also gained insight into the core principles that underlie modern computing.
With this knowledge, you hold the key to deciphering binary mysteries and translating them into the decimal realm, allowing you to harness the power of numbers in novel and innovative ways.