Within the realm of Python programming, the act of type casting, also commonly referred to simply as “casting,” embodies the intricate procedure of altering the manifestation of a given data type into another. This maneuver encompasses a spectrum of modifications: the translation of an integer into a string, the morphing of a float into an integer, or the transition from a string to a list, and so forth.
The utilization of the term “casting” in the programming domain mirrors its etymological roots in the sphere of metallurgy, where the casting technique entails the pouring of molten metal into a cast to create a distinct configuration. Analogously, when one undertakes the casting of a value from one data category to another within Python’s dominion, a metamorphosis transpires—a reforming of the value, analogous to forging it into a new mold, or more fittingly, type, to conform harmoniously to one’s objectives.
In conjunction with the practice of “casting,” an array of alternate designations exists to delineate the process of transmuting a data type from one manifestation to another in the programming realm. Such designations encompass type conversion, type coercion, and type transformation, collectively encapsulating the malleable artistry of data manipulation.
Mastering Type Casting in Python: Unveiling the Power of Built-In Conversion Functions
In the realm of Python programming, the ability to seamlessly transform one data type into another opens up a world of possibilities. Whether you’re refining data for calculations, ensuring compatibility between variables, or enhancing the user experience, type casting comes to your aid. Python generously offers a range of built-in functions tailored for this very purpose. In the following sections, we’ll delve into these conversion functions, uncovering their nuances and potential applications.
Elevating Data Transformations: Unveiling Python’s Conversion Arsenal
Python equips you with a toolkit of conversion functions that gracefully maneuver through the intricacies of data types. These functions possess the prowess to morph your data while maintaining its essence. Here are the stalwart functions that command this transformation symphony:
int() – Forge the Integer Path
- Purpose: Transmute a value into an integer, stripping away decimal points;
- Applications: Ideal for converting user inputs or extracted values into integers, crucial for numerical operations;
- Pro Tip: Handle potential exceptions using try-except blocks when converting non-numeric strings to integers.
float() – Embrace the Floating Point Metamorphosis
- Purpose: Bestow a floating-point identity upon your value, including fractional and decimal components;
- Applications: Vital for computations requiring precision, such as scientific simulations and financial models;
- Pro Tip: Be aware of floating-point precision issues when performing complex calculations.
str() – The Alchemical Conversion to Strings
- Purpose: Transfigure diverse data types, primarily integers and floats, into human-readable strings;
- Applications: Valuable for generating user-friendly outputs and formatting data for display;
- Pro Tip: Utilize string formatting techniques to enhance the readability and aesthetics of the resultant strings.
list() – The Shape-Shifting Enigma for Sets
- Purpose: Reshape sets into lists, ensuring ordered and mutable collections;
- Applications: Facilitates operations that require indexing, appending, or modifying elements within a collection;
- Pro Tip: Leverage list comprehensions for concise and efficient transformation of set elements.
tuple() – Weaving the Tuple Tapestry from Lists
- Purpose: Weave the threads of lists into an immutable tapestry of tuples;
- Applications: Suitable for scenarios where data integrity and immutability are paramount, such as dictionary keys;
- Pro Tip: Understand the performance trade-offs between lists and tuples when dealing with large datasets.
set() – The Conjurer of Distinct Elements
- Purpose: Summon a set into existence, purging duplicates from your data;
- Applications: Crucial for tasks where uniqueness matters, such as finding distinct items in a collection;
- Pro Tip: Remember that sets are unordered, so maintain awareness of their unsorted nature.
A Glimpse of the Symphony: Demonstrating Python’s Conversion Mastery
The nomenclature of each conversion function reveals its transformative destiny. Now, let’s exemplify the wizardry of these functions through a captivating snippet:
original_value = “42”
integer_value = int(original_value)
print(f”Original Value: {original_value}”)
print(f”Transformed to Integer: {integer_value}”)
This enchanting code snippet showcases the metamorphosis of a string, encapsulating the essence of the int() function. Embark on the journey of executing this code to witness the seamless transition from a string to an integer.
Diving Deeper: Guided Exploration and Alternatives
Embark on a quest for deeper understanding by delving into the following tutorials that unravel the intricacies of each conversion function and their alternatives:
- Harnessing the Power of int(): Unearth the nuances of integer conversion and discover alternative strategies to handle diverse scenarios;
- Navigating the Realm of float(): Plunge into the realm of floating-point conversions, while learning about mitigating precision challenges;
- Strings Unveiled: A Journey with str(): Embark on a voyage to comprehend string conversions, alongside advanced techniques for formatting;
- Mastering list(): From Sets to Lists: Master the art of reshaping sets into lists and explore alternative approaches for data transformation;
- Taming Data with tuple(): Navigate the landscape of tuple conversions, unravel their immutability, and explore scenarios where they shine;
- Set Transformation Demystified: Unravel the magic of set conversions, accompanied by insights into their applications and performance attributes.
In the grand tapestry of Python programming, type casting stands as a cornerstone of data manipulation and transformation. Armed with a compendium of conversion functions, you possess the tools to elegantly navigate the intricate terrain of data types. Whether you’re orchestrating mathematical symphonies or crafting user-centric experiences, the power of type casting is yours to wield. As you embark on your coding odyssey, let these insights be your guiding constellations in the celestial expanse of Python’s capabilities.
Illustrating Type Casting in Python: Converting Strings to Integers
Let’s delve into the intriguing realm of type casting in Python, where we will embark on an exploration of converting a string, represented by the str() data type, into an integer, hailing from the int() domain. This enlightening example, showcasing the versatility of type conversion, serves as a gateway to comprehending similar transformations across various functions.
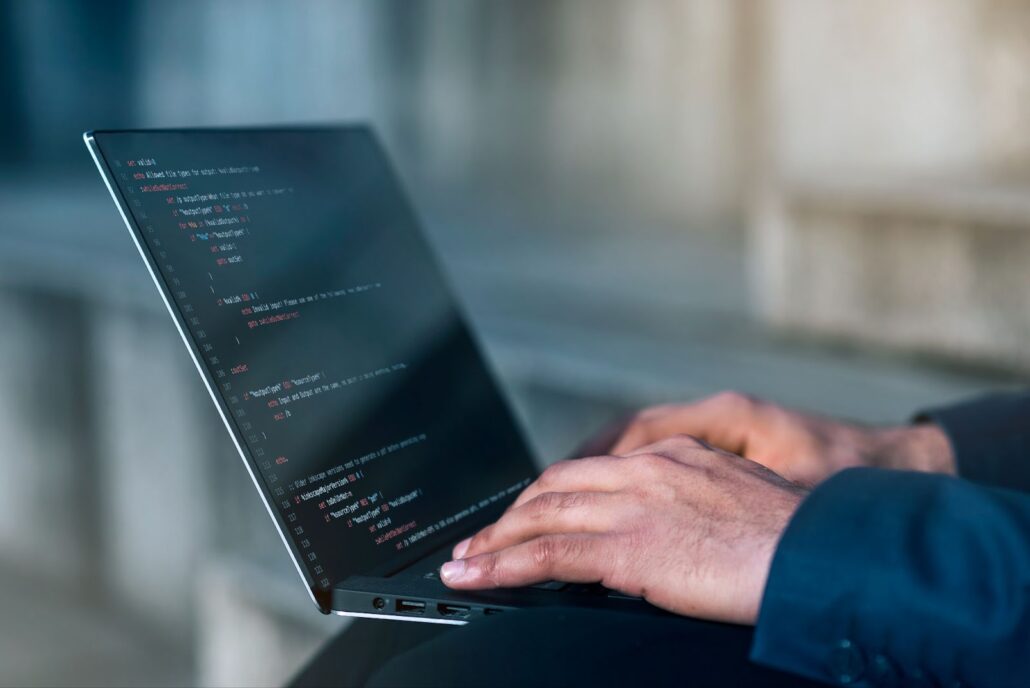
Setting the Stage:
Imagine we have a variable x, elegantly housing the string “10”. Despite the apparent numeric nature of 10, it is nestled within quotes, rendering x a member of the str() family. Our journey commences here, as we embark on a quest to morph this string into a bona fide integer.
The Enchanting Metamorphosis:
Behold, the incantation that breathes life into our endeavor:
x = “10”
y = int(x)
Intriguingly, as the int() function gracefully intercedes, the enchanting metamorphosis transpires. The essence of x is transmuted into its integer counterpart, a feat only possible through the art of type casting. Witness the embodiment of this alchemical conversion, as y gracefully cradles the newly minted integer.
The Grand Reveal:
A pivotal moment arrives as we unravel the transformation’s culmination:
print(y) # Output: 10
The console resounds with the triumphant proclamation of 10, the transformed integer. Gaze upon this revelation, a testament to the power of type casting, as we bridge the chasm between disparate data types.
Deconstructing the Magic:
Diving deeper, let’s dissect the mechanics that underpin this enchanting act of type casting:
- The ethereal entity ‘x’ houses the textual representation “10”, its numeric essence encased in quotes, cloaking it in the garb of a string;
- Enter the int() function, a maestro of metamorphosis, summoned to perform the conversion. It bestows upon ‘y’ the numeric identity that once eluded ‘x’;
- Finally, the curtains part, unveiling the transformed ‘y’ to the world through the incantation of ‘print’. The console reverberates with the digit “10”, a testament to the seamless synergy between human intention and machine execution.
Conclusion
In the symphony of Python’s data manipulation, casting emerges as a melodious refrain, harmonizing the diverse notes of different data types into a coherent composition. Through this exploration of casting in Python, we’ve ventured deep into the heart of a transformative process that transcends mere syntax. Casting, like a skilled artisan, molds and sculpts data, enabling it to seamlessly traverse between varying forms, breathing life into algorithms, applications, and computations.
So, let us venture forth, equipped with the arcane wisdom of casting, to weave spells of code that dazzle and astonish. As we delve deeper into the realms of Python’s capabilities, casting stands as a reliable companion, ready to lend its transformative touch to our endeavors. With every cast, we inch closer to mastery, sculpting our code with precision, and etching our mark upon the ever-evolving tapestry of programming excellence.