If you’ve misplaced the password for your Django project’s Admin or Superuser, there’s no need to establish a new superuser account. Provided you recall the Superuser’s username or remain logged into the admin interface, you can effortlessly update the Django admin password using the strategies I’m about to outline.
Resetting the Django Superuser Password Using the ‘changepassword’ Command
When it comes to efficiently resetting the password of a Django admin or superuser, the ‘changepassword’ command associated with python manage.py proves to be an invaluable tool. This article offers a detailed step-by-step guide on how to navigate this process, ensuring a secure and swift password reset.
Prerequisites
Identification of Superuser Username: While it’s crucial to be aware of your superuser’s username, there’s flexibility if you only maintain a single superuser for your Django project. If you’ve forgotten both the password and the username but have only one superuser, Django will still allow you to reset the password.
Instructions for Resetting Django Admin Password via the ‘changepassword’ Command
Step 1: Accessing the Command Line Interface and Initiating the ‘changepassword’ Command
- Launch the terminal interface on your computer;
- Navigate to your Django project directory. This can be identified by the presence of the ‘manage.py’ file within;
- Ensure that your virtual environment specific to the project is activated. This ensures all dependencies and libraries are available for use;
- Enter the command: python manage.py changepassword [username]
Note: Replace [username] with the superuser’s username you aim to reset. If you’re unsure or have forgotten the username but have only one superuser, simply omit the username.
For instance:
$ python manage.py changepassword john_doe
Changing password for user ‘john_doe’
Password:
Password (again):
Password changed successfully for user ‘john_doe’
A security feature ensures that as you input the password, it remains invisible in the terminal, preventing over-the-shoulder snooping.
If there are multiple superusers and you attempt the command without specifying a username, Django will default to applying the operation for the superuser that is presently logged in.
Step 2: Setting and Confirming the New Password
- Django will prompt the user to enter a fresh password;
- To ensure consistency and that the user recalls the password, Django will request the password to be entered a second time for confirmation;
- Upon successful matching of both password inputs, Django will display a confirmation message: ‘Password changed successfully for user ‘[username]’.
Make it a priority to select a robust password, combining letters, numbers, and symbols. Regularly updating passwords is a good practice to ensure the security of your Django projects.
Resetting the Superuser Password in Django Using the set_password() Function
The set_password() function offers a secure and straightforward approach to reset the superuser’s password in Django. Whether you’re working within the Django interactive shell or directly in your Python code, follow the detailed steps below to reset the superuser password efficiently.
Pre-requisites:
Ensure you have the Django superuser’s username at hand. Without this, the process can’t proceed.
Step 1: Launching the Django Interactive Shell:
To start the Django interactive shell, execute the following command in the terminal of your Django project:
$ python manage.py shell
Upon successfully launching the shell, each console line will begin with >>>, signifying the shell’s active status.
Step 2: Retrieving the Desired Superuser:
To reset a superuser’s password, first locate the user:
Import the necessary function to gain access to the user model:
>>> from django.contrib.auth import get_user_model
Extract a list of all superusers:
>>> list(get_user_model().objects.filter(is_superuser=True).values_list(‘username’, flat=True))
Here, get_user_model() allows access to the list of registered users. Filtering by is_superuser=True narrows down the list to only superusers. To target a specific superuser, further refine the filter with the superuser’s username.
Select the target user:
user = get_user_model().objects.get(username=u)
Upon executing this, the desired superuser is stored in the variable named user.
Step 3: Utilizing the set_password() Function:
To set a new password for the chosen superuser:
user.set_password(password)
Here, password represents the new password desired for the superuser.
Step 4: Storing the Updated Password:
After resetting the password, ensure the changes reflect in the database by saving the user object:
user.save()
Automating the Process in Python Code:
For those who prefer not to use the interactive shell, encapsulate the entire procedure within a Python function. Below is an illustrative example:
from django.contrib.auth import get_user_model
def reset_password(u, password):
“””
Resets the password of a Django superuser.
Parameters:
– u (str): Username of the superuser.
– password (str): New desired password.
Returns:
– str: Status message indicating success or an error.
“””
try:
user = get_user_model().objects.get(username=u)
user.set_password(password)
user.save()
return “Password has been changed successfully”
except Exception as e:
return f”Error: {e}”
This function, reset_password, requires the superuser’s username and the new password as inputs. If the specified user is found, their password gets reset and saved to the database. If the user is absent or an error occurs, an appropriate message gets returned.
Resetting Django Superuser Password through the Admin Panel
The Django admin panel offers an in-built feature that allows superusers to reset their passwords. However, there are specific prerequisites to use this method:
Prerequisites:
- Knowledge of the Old Password: This method is unique because it necessitates the user to remember their previous superuser password. It’s implemented for added security;
- Access to Admin Panel: The superuser must be logged into the admin panel. Remember, regular users do not possess the authority to modify the passwords of other users.
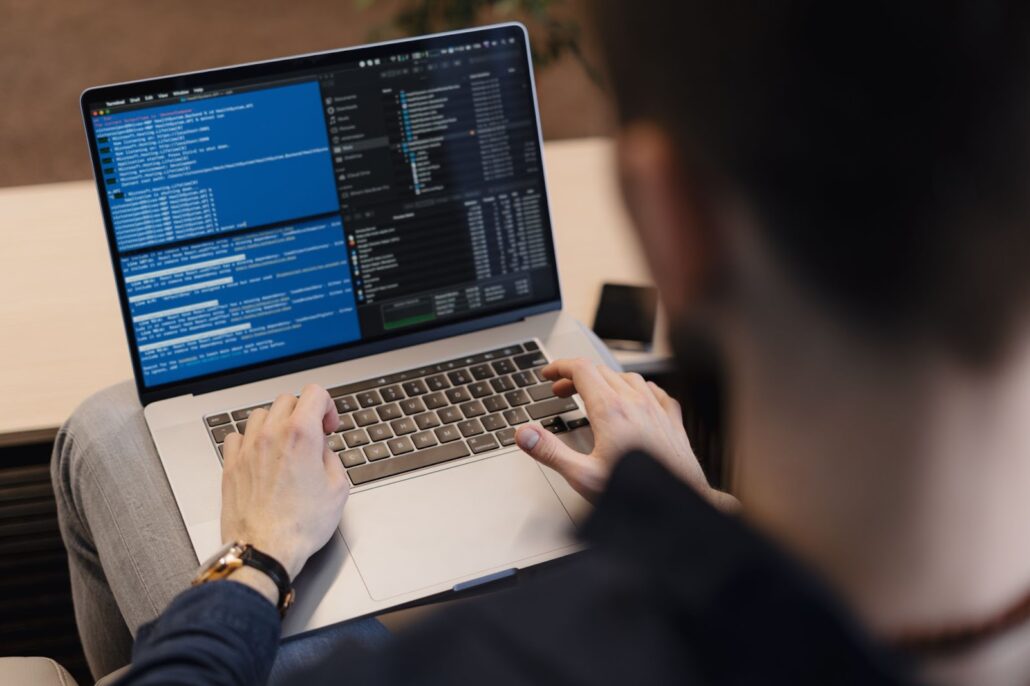
Detailed Step-by-Step Guide:
1. Activate the Server and Access the Admin Panel:
- First, ensure that the Django project server is up and running;
- Use a browser to navigate to http://127.0.0.1:8000/admin;
- If already logged in: The dashboard will appear;
- If not logged in: The system will redirect to http://127.0.0.1:8000/admin/login/?next=/admin/, prompting for login credentials. If the latter occurs, resetting the password via this method won’t be feasible.
2. Locate and Click the “CHANGE PASSWORD” Option:
- In the Django admin interface, look at the top right corner;
- Click on the “CHANGE PASSWORD” link to proceed.
3. Input the Old Password:
- A key distinction of this approach is its requirement for the old password. This provision bolsters security;
- If the old password is misplaced or forgotten, this method is rendered ineffective.
4. Input and Verify the New Password:
There will be two input fields:
- The first field is for entering the new desired password;
- The second field requires re-entry of the new password to confirm accuracy;
- It’s imperative to follow the password guidelines provided below the input fields to ensure the creation of a robust and secure password.
5. Finalize the Change:
- Locate and click the “CHANGE PASSWORD” button, situated at the lower right corner of the form;
- If executed correctly, the system will navigate to a confirmation page with the message: ‘Password change successful’.
By following the above comprehensive guide, superusers can successfully modify their Django passwords using the admin panel.
Conclusion
In the rapidly evolving world of web development, keeping security at the forefront is paramount. There may be occasions where administrators or developers find themselves locked out of the Django admin panel due to a forgotten password or other unforeseen circumstances. Thankfully, Django, as a robust and versatile framework, offers multiple avenues to address this issue. We’ve explored three distinct methods to reset the admin/superuser password—directly from the command line, via the shell, and through database manipulations. Each has its own advantages and use-cases. Whether you’re a beginner just starting out with Django or a seasoned developer, these methods can serve as invaluable tools in your toolkit. Always remember to ensure that any changes made are done so securely, and consider regularly updating passwords as part of a comprehensive security strategy.