In the realm of web development, crafting robust and efficient applications demands a keen understanding of every facet of the chosen framework. Django, a popular and powerful Python web framework, provides developers with a comprehensive toolkit to create dynamic and feature-rich websites and applications. However, as developers delve deeper into Django’s intricacies, they inevitably encounter the nuanced concepts of “blank” and “null” fields within its ORM (Object-Relational Mapping) system.
In this article, we embark on a journey to demystify the concepts of “blank” and “null” within the Django framework. We will delve into their definitions, explore their significance in the context of database design, and provide real-world examples that illustrate their impact on data integrity and application behavior. By the end of this exploration, you will not only have a clear grasp of the differences between “blank” and “null” but also possess the knowledge necessary to make informed decisions when designing and implementing your Django models. So, let’s unravel the intricacies of Django’s “blank” and “null” attributes and equip ourselves with the tools to create more efficient and resilient applications.
Understanding the Distinction: null=True vs. blank=True in Django Model Fields
Delving into the realm of Django’s model fields, the contrast between null=True and blank=True serves as a pivotal concept, steering the course of data validation at different layers of your application. This distinction embarks on a journey where null orchestrates the symphony of database-level validation, while blank takes the center stage during form field validation at the application level. This intriguing interplay of null and blank can profoundly influence your data integrity and user interactions.
Illustrating with a Practical Model
Imagine sculpting a model that encapsulates the essence of a Book. In this literary realm, each book is a masterpiece, enriched with a title that captures its essence, a date when its wisdom was unveiled, and a time when readers can immerse themselves in its pages.
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
pub_date = models.DateField()
pub_time = models.TimeField()
The Inherent Requirement: All Fields Required
In the realm of Django’s model fields, fields have an innate craving for data – they yearn to be graced by values. By default, they assume a stance that all data they encapsulate is essential, obligatory, and non-negotiable. The fabric of this model demands a commitment to completeness.
Unlocking the Realm of Optionality
Yet, in the dance between models and data, there arise moments when a field wishes to relinquish its demanding nature, to transform from a staunch mandatory participant to a graceful optional contributor. Enter the concept of optionality, where a field, once required, becomes a choice. In this enchanting waltz of form and function, we seek a way to create fields that stand open-handed, embracing both data and void.
Embracing the optional with blank=True
To invite optionality into the heart of a model’s field, we invoke the power of blank=True. A signal to the validators, this command bestows the field with the gift of emptiness. It allows the form to accept the graceful absence of data without protest, enabling users to submit blank responses.
pub_time = models.TimeField(blank=True)
However, the tale does not conclude here. A form’s heart might welcome the void, but the database’s foundation demands more than mere acquiescence.
Bridging the Chasm: null=True
As our model’s story journeys from form to database, the terrain shifts. The ground beneath the fields transforms into the realm of databases, a space that craves definition, a space that abhors ambiguity. null=True emerges as the bridge, the mediator that communicates with the database, whispering the secret language of nullability.
Adding null=True to a field signifies a truce between the form and the database, an agreement that states, “This field may stand empty in the halls of the database.” The model’s voice echoes through the corridors of SQL, ensuring that the database knows how to interpret a field’s absence.
pub_time = models.TimeField(blank=True, null=True)
The caveat to remember is the evolution of schema. Altering the null value of a model field necessitates the crafting of a new migration, a meticulous transformation that harmonizes the database’s schema with the model’s desires.
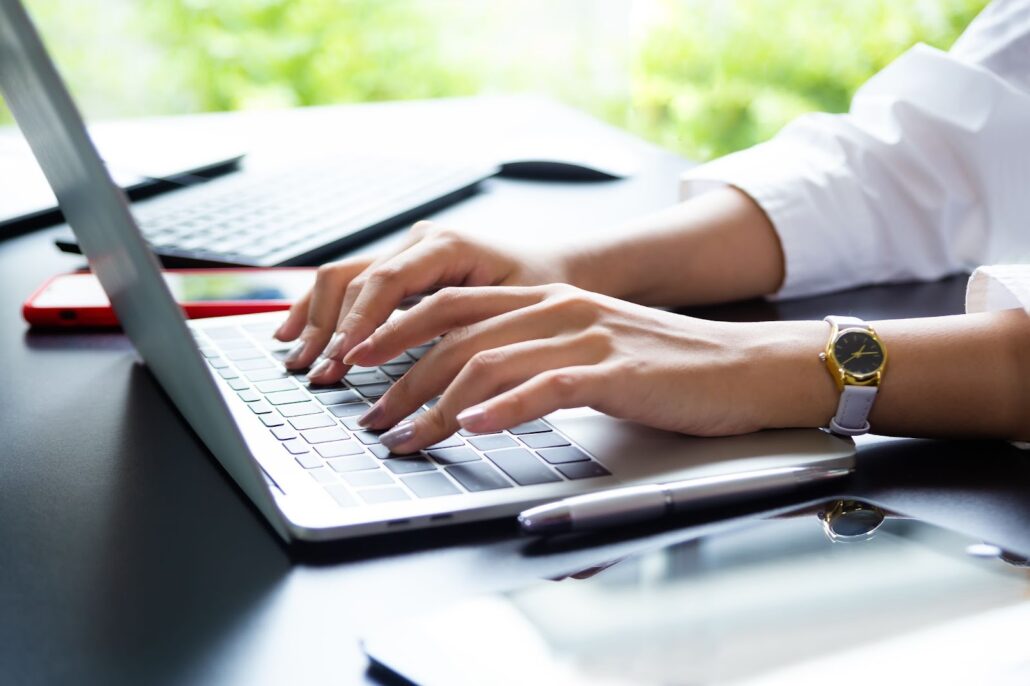
Whispers of Exception: CharField and TextField
Amidst the symphony of optionality, there exists a duet that plays by different rules – the harmonious partnership of CharField and TextField. These fields dance to a unique rhythm, where silence is not shrouded in nullness but instead adorned with an empty string, a token of presence in absence.
title = models.CharField(max_length=200, blank=True)
pub_date = models.DateField()
pub_time = models.TimeField(blank=True, null=True)
In this enchanting choreography, null=True finds itself on the sidelines, observing the silent eloquence of an empty string.
Guiding Principles and Insights
As you embark on your Django journey, let these guiding principles illuminate your path:
- Embrace Intentional Optionality: Blankness is not a sign of lack but a canvas for possibilities. Harness blank=True when you desire fields that gracefully accept the absence of data;
- Negotiate with Databases: The language of databases resonates with the notes of null. Employ null=True to mediate between your model’s aspirations and the database’s requirements;
- Respect the CharField and TextField Duet: For CharField and TextField, nullness takes a back seat. Instead, appreciate the elegance of an empty string, a quiet declaration of existence within the realms of absence;
- Evolution Requires Migration: When altering the null attribute, heed the call of migrations. Craft these artifacts with care, ensuring your database’s schema stays in tune with your model’s evolution;
- Conventions Beckon: While you can paint the canvas with null=True, consider Django’s conventions. Let the dance of optionality manifest through blankness and an empty string, preserving harmony within your code.
As your Django odyssey unfolds, remember that null=True and blank=True are more than technical annotations – they are the threads weaving through the fabric of your application’s narrative, shaping its interactions and echoing its essence.
Conclusion
In conclusion, the distinction between “blank” and “null” in Django holds paramount significance for database design and data integrity within your web applications. Understanding and correctly implementing these attributes can have a profound impact on the functionality, reliability, and maintainability of your Django projects.
As you embark on your Django development journey, remember that a well-informed decision regarding “blank” and “null” attributes can save you time, effort, and potential pitfalls down the road. By grasping the nuances of these attributes and tailoring their usage to your application’s needs, you’ll be better equipped to craft robust, reliable, and user-friendly web solutions using the power of Django.