In the realm of web development, Django stands as a powerful and versatile framework for building robust applications. While Django greatly simplifies the process of creating dynamic web pages, it also recognizes the significance of static files (SF) such as stylesheets, JavaScript scripts, and images to enhance user experience.
To ensure seamless deployment and an optimal user interface, Django provides a mechanism for collecting and efficiently managing these static files.
Understanding Static Files in Django
In the landscape of web development, grasping the concept of SF holds vital importance when working with frameworks like Django. Django, a potent and popular framework, empowers the creation of dynamic and interactive web applications.
However, beyond the framework-generated dynamic content, these assets are essential for elevating the aesthetics and functionality of web applications.
What Are Static Files?
These encompass a broad range of resources that remain unchanged throughout the lifespan of a web application. They include Cascading Style Sheets (CSS), JavaScript, images, fonts, and more.
In contrast to dynamic content, which is generated “on the fly” based on user interaction or data from databases, these elements do not alter their content or behavior during the application’s runtime. Instead, they are delivered directly to the user’s browser, providing visual and interactive components that complement the dynamic aspects of the application.
The Role in Web Development
SF play a pivotal role in web development, enhancing site usability and functionality. Here are some key functions:
- Enhancing User Interface (UI): CSS files style the layout and appearance of the site, making it visually appealing and user-friendly. JavaScript enables interactive features like form validation, animations, and dynamic content updates without needing to reload the entire page;
- Boosting Performance: Offloading certain functions to JavaScript and utilizing optimized images allows web developers to create faster and more responsive applications. Proper organization and minification reduce loading times and improve overall site performance;
- Maintaining Branding: Elements like logos, icons, and custom fonts contribute to maintaining a consistent brand style across different site pages.
Efficient cache management also reduces server load, leading to more resource-effective usage.
Django’s Approach to Working with Static Files
During development, Django’s built-in development server serves these files directly from the app directories, ensuring seamless testing. However, such an approach isn’t suitable for production environments due to potential performance and security issues.
To address this, Django offers the collectstatic management command. This command gathers required items from various apps and any specified additional locations, centralizing them into one directory.
This directory, defined by the STATIC_ROOT parameter in the project settings, can be served by a dedicated web server, such as Nginx or Apache, in production. This approach simplifies deployment, enhances security, and optimizes the performance of serving static assets.
Why Collect Static Files?
Collecting files in a Django web application is a crucial step in ensuring efficient deployment, improving user experience, and maintaining a consistent and professional appearance.
While dynamic content defines interactive and personalized aspects of a web application, these files play an equally important role in its overall success. Let’s explore the reasons why such collection is necessary:
- Deployment Optimization: During development, the Django development server serves files directly from relevant app directories. However, this approach becomes inefficient when transitioning to a production environment. Collecting allows you to gather all these scattered assets in one place, streamlining the deployment process. This centralized structure minimizes the risk of missing elements, reduces complexity, and ensures smooth and consistent application deployment;
- Performance Enhancement: The collected files can be efficiently managed and optimized for production use. Methods such as minification, compression, and bundling can be applied to CSS and JavaScript files, reducing their size. This optimization directly contributes to faster page loading, crucial for maintaining seamless and responsive user interactions. Additionally, to further enhance performance, caching mechanisms can be employed to reduce the need for repeated requests;
- Security Boost: Centralizing files in a dedicated directory allows for better access control and security configuration. In a production environment, exposing individual app directories containing such elements can pose security risks. By serving these files from a single controlled location, the attack surface is minimized, reducing potential vulnerabilities exploitable by malicious actors;
- Consistent Branding and User Experience: Often including assets like logos, icons, and fonts, these files contribute to creating a unified visual style and branding across the application. By collecting and organizing these assets, web developers can ensure that the application’s design remains consistent and professional, reinforcing the brand image and delivering a memorable user experience;
- Efficient Caching Strategies: Web browsers cache files, speeding up loading when users revisit the same pages. Centralization allows more efficient caching strategies. This ensures users receive updated content when necessary while using cached resources for improved site usability;
- Maintenance and Scalability: As your Django application evolves and grows, having a well-structured and centralized repository makes maintenance and scalability more manageable. Adding new features, updating styles, or altering JavaScript behavior becomes simpler as you have a clear understanding of where all assets are located.
Centralization and optimization of these assets enable developers to establish a consistent and appealing visual style, accelerate page loading, simplify maintenance, ultimately resulting in a more successful web application.
Step-by-Step Guide: Collecting Static Files in Django
In this step-by-step guide, we’ll walk through the process of collecting SF using Django’s built-in collectstatic command.
Step 1: Configure File Parameters
Open your project’s settings file (settings.py) and configure necessary parameters for working with SF.
python
Copy code
# settings.py
# Define the URL prefix for static files
STATIC_URL = ‘/static/’
# Specify the absolute path to the directory where static files will be collected
STATIC_ROOT = os.path.join(BASE_DIR, ‘staticfiles’)
Here, STATIC_URL sets the URL prefix for serving static files, and STATIC_ROOT specifies the absolute path to the directory where collected static files will be stored. You can modify the directory path if needed.
Step 2: Organize Your Application’s Files
Within each Django app, create a static directory to hold that app’s static files. For example:
arduino
Copy code
your_app/
static/
your_app/
css/
js/
images/
Place CSS, JavaScript, images, and other static assets in their respective directories within each app’s static directory.
Step 3: Run the collectstatic Command
Open your terminal or command prompt, navigate to your project’s root directory, and execute the collectstatic command using the following syntax:
bash
Copy code
python manage.py collectstatic
Django will scan all apps in your project and collect their SF, copying them to the directory specified in the STATIC_ROOT setting.
Step 4: Verify Collected Files
After running the collectstatic command, check the directory specified in STATIC_ROOT to ensure SF have been collected. The structure should resemble the static directories within your apps.
Step 5: Deploy Collected Files to Production Environment
In a production environment, configure your web server (e.g., Nginx, Apache) to serve collected SF from the directory specified in STATIC_ROOT. Set up the web server to handle SF efficiently and implement caching mechanisms for performance optimization.
Additional tips:
- Versioned Files: To prevent browser caching issues when making changes to SF, consider using versioning methods. This can be achieved by adding a version number or timestamp to the URLs of relevant files;
- Third-party Libraries: If your project utilizes third-party libraries or packages with their own files, ensure these files are also collected and served correctly. Some packages might require additional configuration;
- Automate the Process: In a Continuous Integration/Continuous Deployment (CI/CD) pipeline, automate the execution of the collectstatic command to ensure files are collected and deployed correctly.
Collecting files in Django is a fundamental step in preparing a web application for deployment. By configuring project parameters, organizing SF within apps, and utilizing the collectstatic command, you can centralize and optimize assets, ensuring smooth and efficient user experiences upon launching the application.
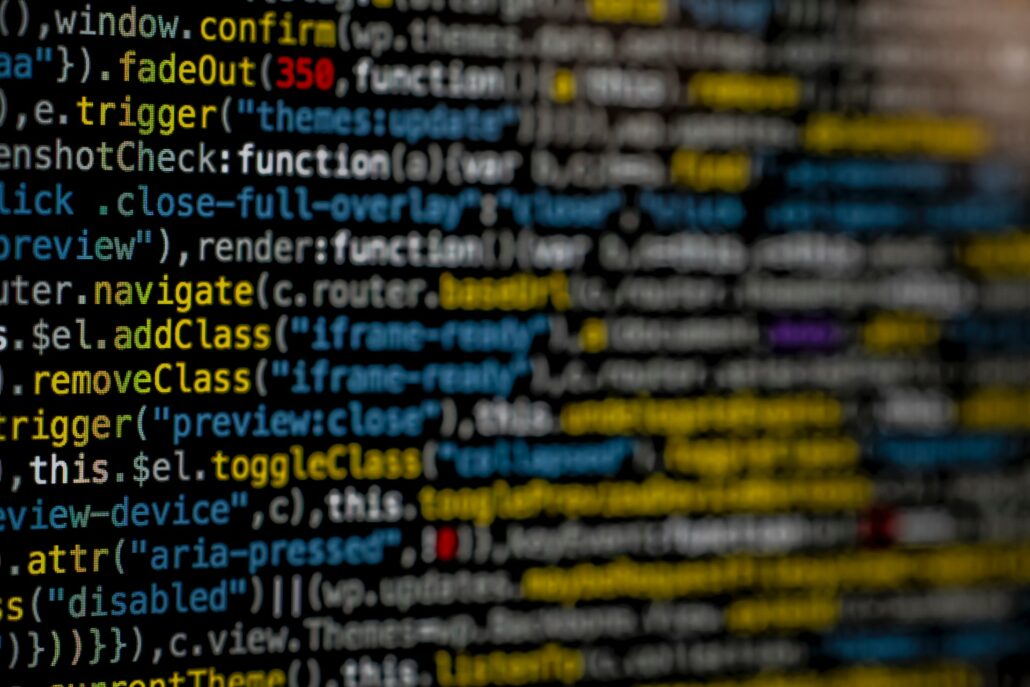
Conclusions
Collecting files in Django is a crucial deployment step, enhancing the performance and reliability of your web application. Utilizing the collectstatic command, you can easily gather assets from various sources into a single directory, streamlining deployment and enhancing user convenience.
Thus, as you embark on the path of Django development, don’t forget to master the art of collecting SF, so your web applications can shine in all their glory.