It seems you’ve encountered a simple yet easily overlooked step. The crucial action is registering a model in the Django admin. This single step instantly makes it visible within the Django interface.
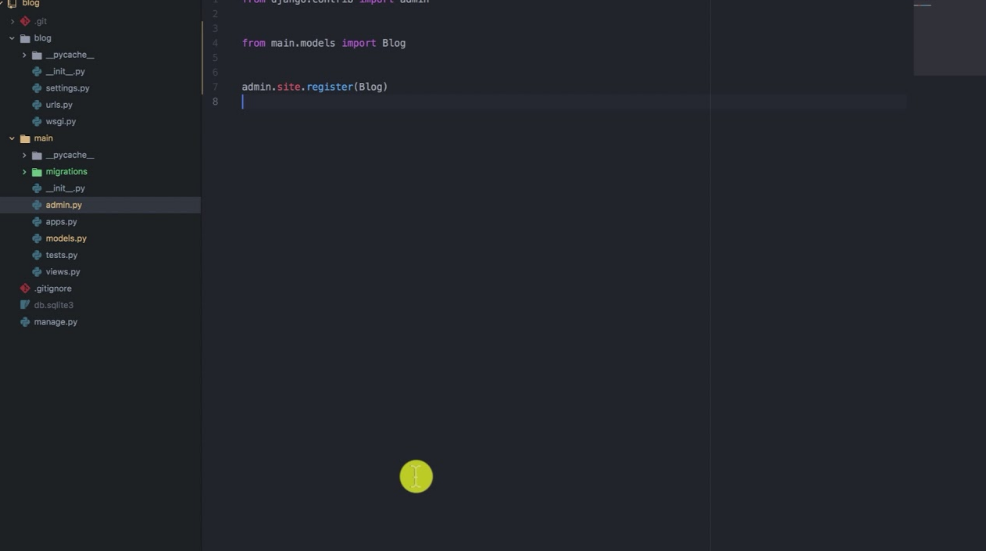
But there’s no need for disappointment, as you’ve stumbled upon the perfect online resource that comprehensively covers the process of registering a model in the Django admin.
By the end of this guide, you’ll have gained enough experience to smoothly register your models in the Django administration system.
What is Django Admin?
So, let’s get started.
As an illustration, imagine you’re building a blog application on Django with models located in the models.py file.
models.py:
python
Copy code
from django.db import models
from django.contrib.auth import get_user_model
User = get_user_model()
class Author(models.Model):
User = models.OneToOneField(User, on_delete=models.CASCADE)
profile_picture = models.ImageField()
def __str__(self):
return self.user.username
class Category(models.Model):
title = models.CharField(max_length=20)
def __str__(self):
return self.title
class Post(models.Model):
title = models.CharField(max_length=50)
overview = models.TextField()
timestamp = models.DateTimeField(auto_now_add=True)
comment_count = models.IntegerField(default=0)
author = models.ForeignKey(Author, on_delete=models.CASCADE)
thumbnail = models.ImageField()
categories = models.ManyToManyField(Category)
def __str__(self):
return self.title
Initially, the complexity of this code might seem overwhelming, but allow me to provide a brief explanation of its functionality before delving into the specifics of model registration in the Django interface.
How to Register All Models In Django Admin
If you aim to streamline the process of registering all models in Django, eliminating the need to re-register them across different Django applications within your project, follow these concise instructions.
Start by creating an “admin.py” file within your project directory and structure it as follows:
Next, navigate to the “settings.py” file in the same directory and expand the list of installed applications by adding the newly created folder to it.
settings.py:
python
Copy code
INSTALLED_APPS = [
‘django.contrib.admin’,
‘django.contrib.auth’,
‘django.contrib.contenttypes’,
‘django.contrib.sessions’,
‘django.contrib.messages’,
‘django.contrib.staticfiles’,
‘post’,
‘blog’ # new (project folder name)
]
Now, insert the following code snippet into the “admin.py” file within your project folder:
admin.py (project folder):
python
Copy code
from django.contrib import admin
from django.apps import apps
all_models = apps.get_models()
for model in all_models:
try:
admin.site.register(model)
except admin.sites.AlreadyRegistered:
pass
Start the server and take a look at your admin panel. The appearance of the admin interface will match the image that follows.
Conclusions
In conclusion, your journey through registering models in the Django admin is complete. Remarkably, you’ve acquired skills in automating the registration as a whole. I hope this guide has been clear and enables you to independently execute the described steps.