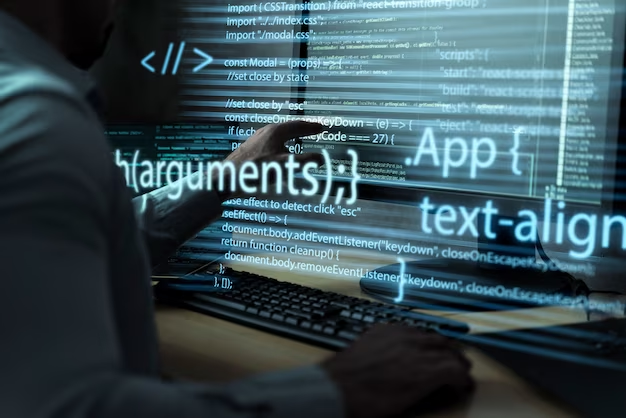
Django, the beloved Python web development framework, offers an array of built-in features that streamline the development process. From creating a superuser for your admin panel to customizing it extensively, Django simplifies many tasks. However, understanding the version of Django you’re working with is crucial to ensure compatibility and take advantage of the latest features. In this comprehensive guide, we delve into four effective methods to check Django version. Whether you’re a beginner or an experienced developer, knowing these methods can save you time and help you stay on top of your Django project.
Method 1: Using Django Scripts
Django’s versatile manage.py command-line utility provides more than just the ability to manage your project. You can also use it to check the installed Django version. Open your terminal or command prompt and run:
python manage.py version
Alternatively, you can use the Django-admin command:
django-admin –version
Both of these commands will display the version of Django you have installed globally or within your virtual environment.
Method 2: Checking Via Django Shell
The Django shell offers an interactive Python environment to run code. You can utilize it to check the Django version as well. Access the shell by running:
python manage.py shell
Once inside the shell, execute the following Python code:
import django print(django.get_version())
Alternatively, you can achieve this in a single line:
python -c “import django; print(django.get_version())”
Method 3: Utilizing Pip
Pip, Python’s package installer, provides two convenient methods to check the Django version. Use the following commands:
To get detailed information:
pip show django
To only display the version:
pip freeze | grep Django
Method 4: Harnessing Django’s Built-in Functions
Django offers built-in functions that allow you to check the version using the Django shell. Import the appropriate module and use the methods as shown below:
python manage.py shell
from django.utils import version print(version.get_version())
Comparative Table of Django Version Checking Methods
For your convenience, we have collected all these methods in one table, and we suggest you pay attention to it.
Method | Command/Code | Additional Information |
---|---|---|
Method 1: Django Scripts | python manage.py version | Uses the standard manage.py command to output the Django version. |
django-admin –version | An alternative command for version output, recommended by the official Django documentation. | |
Method 2: Django Shell | python manage.py shell | Enters the Django interactive shell, where you can import Django and check the version. |
import django<br>django.version | Imports Django and outputs the version using the version attribute. | |
Method 3: Using Pip | pip show django | Displays not only the Django version but also additional package information. |
pip freeze | grep Django | Shows the Django version as well as other dependencies in the project. | |
Method 4: Django Built-In Functions | version.get_version() | Utilizes the built-in get_version() method to output the Django version, imported from django.utils. |
version.get_complete_version() | An alternative method to output the version in a different format. |
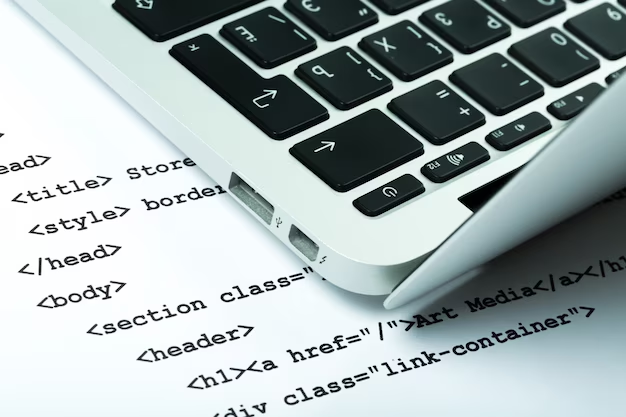
Conclusion
By now, you’re equipped with multiple methods to check the Django version. Whether you prefer scripts, the interactive shell, Pip, or Django’s built-in functions, these techniques ensure you’re using the correct version for your project. Stay up-to-date with the latest features and enhancements to make the most of your Django development journey. If you discover new methods or face challenges, share your insights and questions with the developer community. Happy Django coding!
FAQ
If you encounter issues with any of the methods mentioned in this guide, it’s advisable to seek help from the Django community. Online forums, developer communities, and resources like Stack Overflow can provide valuable insights and solutions.
While checking the Django version is crucial, also ensure that other packages and libraries used in your project are compatible with the Django version you’re using. Refer to the official documentation and package release notes for compatibility information.
It’s not recommended to use multiple Django versions within the same project, as it can lead to compatibility issues and make maintenance challenging. Stick to a single Django version for each project to ensure consistency and stability.
While staying updated with the latest Django version is advantageous, upgrading immediately might not be feasible for all projects. Evaluate the new features, changes, and potential compatibility concerns before upgrading.