Mastery of multiplication tables lays a strong foundation in mathematics. Python simplifies this skill through input(), range(), and loop functions. This tutorial explores diverse strategies to create multiplication tables effectively and with ease.
Method 1: Using For Loop
The for loop is a workhorse when it comes to iterating through sequences. In this method, we’ll employ a for loop to generate multiplication tables from 1 to 10.
number = int(input("Enter the number of which the user wants to print the multiplication table: ")) print("The Multiplication Table of:", number) for count in range(1, 11): print(number, 'x', count, '=', number * count)
Method 2: Using While Loop
The while loop offers another approach to crafting multiplication tables. This method is equally effective and involves a bit of variation.
number = int(input("Enter the number of which the user wants to print the multiplication table: ")) count = 1 print("The Multiplication Table of:", number) while count <= 10: print(number, 'x', count, '=', number * count) count += 1
Method 3: Combining Input and Range
Combining the input() function and range() function with a loop enables us to create dynamic multiplication tables.
num = int(input("Enter the number for multiplication table: ")) for i in range(1, 11): print(num, 'x', i, '=', num*i)
Method 4: Advanced Formatting
For a polished multiplication table, Python’s string formatting can be employed to align the output neatly.
number = int(input("Enter the number for multiplication table: ")) print(f"Multiplication Table of {number}:".center(30, '-')) for i in range(1, 11): print(f"{number} x {i} = {number*i}")
Comparing Methods: Which One to Choose?
Here’s a comparative table highlighting the key aspects of each method:
Method | Input Required | Loop Type | Output Format |
---|---|---|---|
Using For | Single number | for loop | Aligned |
Using While | Single number | while loop | Standard |
Input + Range | Single number | for loop | Standard |
Advanced | Single number | for loop | Aligned |
Conclusion
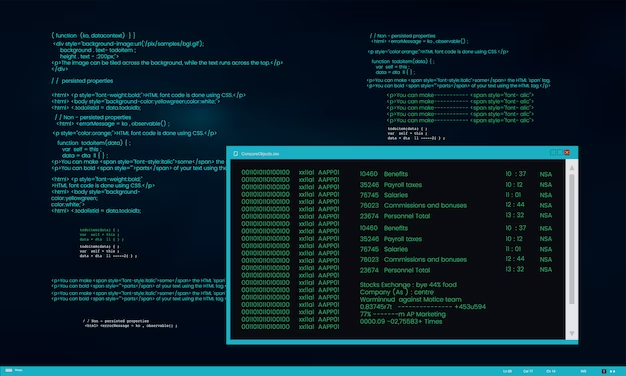
Python’s versatility shines through when it comes to generating multiplication tables. Whether you opt for the simplicity of the for loop, the flexibility of the while loop, or the finesse of advanced formatting, Python empowers you to create elegant and functional tables. Experiment with these methods to enhance your programming skills and mathematical prowess. Happy coding!
FAQ
The choice of method depends on your familiarity with loops and your formatting preferences. If you’re new to programming, the basic for loop or while loop methods are great starting points. If you want more polished output, consider using the advanced formatting approach.
Certainly! The techniques elucidated within this compendium possess remarkable adaptability, enabling the construction of multiplication tables for any positive integer you desire.
The examples in this guide demonstrate tables up to 10 for simplicity. However, you can easily extend the range in the loop to generate multiplication tables of larger numbers.
User input is already incorporated in the methods outlined here. By utilizing the input() function, you can dynamically decide which number’s multiplication table to create.