Python 3 stands as a gateway to the world of programming, known for its simplicity, readability, and versatility. If you’re looking to venture into the realm of coding or expand your programming horizons, this illustrated guide is tailored to meet your needs.
We’ll explore whether Python 3 is the right choice for beginners, delve into the process of learning it from scratch, recommend the best beginner-friendly books, and shed light on what you can expect to learn in the process.
Is Python 3 for Beginners?
Python 3’s user-friendly syntax and clear readability make it an excellent choice for beginners. Its focus on reducing the complexity of code while maintaining its power has made it a popular language for those taking their first steps into the world of programming. Whether you’re a student, professional, or hobbyist, Python 3 provides a welcoming entry point.
How to Learn Python 3 from Scratch
Learning Python 3 from scratch is an exciting journey that requires a structured approach. Start with the basics: understanding variables, data types, and control structures. Progress to functions, modules, and object-oriented programming. Our illustrated guide ensures you grasp each concept visually, solidifying your understanding.
Recommended Learning Path
- Basic Concepts: Explore variables, data types, and operators to establish a solid foundation;
- Control Structures: Master conditional statements and loops to control program flow;
- Functions and Modules: Dive into function creation and module usage for efficient code organization;
- Object-Oriented Programming (OOP): Understand classes, objects, inheritance, and polymorphism.
Which Book Is Best for Python Beginners?
Selecting the right book can significantly accelerate your learning journey. Consider titles like “Python Crash Course” by Eric Matthes, which provides hands-on projects, or “Automate the Boring Stuff with Python” by Al Sweigart, which focuses on practical applications.
These books offer clear explanations and exercises, ideal for beginners seeking a comprehensive guide.
What Do You Learn in Python 3?
Python 3 encompasses a wide array of topics that equip you with practical programming skills.
Core Concepts
- Syntax Simplicity: Python’s clean syntax facilitates easy code comprehension;
- Data Handling: Learn to manipulate strings, lists, dictionaries, and other data structures;
- File Handling: Master reading from and writing to files for data persistence;
- Web Development: Explore frameworks like Flask and Django for web application creation;
- Data Science: Utilize libraries like NumPy, Pandas, and Matplotlib for data analysis and visualization;
- Automation: Use Python to automate tasks and streamline workflows.
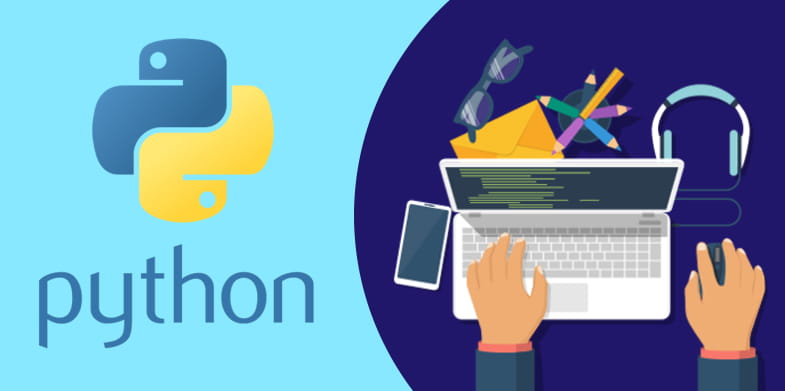
Taking Your First Steps in Python 3: Embracing Simplicity in Syntax
Python 3’s syntax is designed to be human-readable and intuitive, making it a fantastic choice for beginners. Unlike some programming languages that require intricate symbols and punctuation, Python uses indentation to indicate code blocks.
This elegant approach not only makes your code visually appealing but also helps you focus on the logic without getting lost in syntax complexities.
Writing Your First Python Program
Let’s dive right in with a classic “Hello, World!” program. In Python 3, printing a message to the console is as straightforward as:
“`python
print(“Hello, World!”)
“`
That’s it! You’ve just executed your first Python code. This simplicity sets the stage for grasping more intricate concepts.
The Building Blocks: Data Types and Variables
Python 3 provides various built-in data types, including integers, floating-point numbers, strings, lists, dictionaries, and more. Each data type serves a specific purpose, allowing you to manipulate and store different kinds of information.
Integers and Floating-Point Numbers
“`python
age = 25 # An integer variable
temperature = 98.6 # A floating-point variable
“`
Strings
“`python
name = “Alice” # A string variable
message = “Hello, ” + name
“`
Lists and Dictionaries
“`python
fruits = [“apple”, “banana”, “orange”] # A list
person = {“name”: “Bob”, “age”: 30} # A dictionary
“`
Variables: Your Data Containers
Variables allow you to store and manage data in your programs. They act as containers that can hold different types of information, which you can manipulate and modify as needed. Think of variables as labeled boxes that hold specific values.
Controlling Your Code: Conditional Statements and Loops
Conditional statements, like if-else and elif, enable your code to make decisions based on certain conditions. For instance, let’s create a simple program that determines if a person is eligible to vote:
“`python
age = 18
if age >= 18:
print(“You are eligible to vote!”)
else:
print(“You are not eligible to vote.”)
“`
Looping for Efficiency
Loops allow you to execute a block of code repeatedly, making tasks like processing lists or performing calculations efficient. The two main types of loops in Python are the “for” loop and the “while” loop.
Using the “for” Loop
“`python
fruits = [“apple”, “banana”, “orange”]
for fruit in fruits:
print(“I like”, fruit)
“`
Using the “while” Loop
“`python
count = 0
while count < 5:
print(“Count:”, count)
count += 1
“`
Beyond Basics: Functions and Modularization
Creating Functions for Reusability
Functions are blocks of code that perform specific tasks. They promote code reuse and organization, enabling you to break down complex problems into smaller, manageable pieces.
Let’s define a function that calculates the square of a number:
“`python
def square(number):
return number ** 2
result = square(5)
print(“Square:”, result)
“`
Modularizing Your Code
As your programs grow in complexity, keeping everything in a single file becomes unwieldy. Python 3 allows you to create modular code by splitting your program into multiple files (modules) that can be imported and used in other programs. This modular approach enhances code readability and maintainability.
Applying Your Python 3 Skills
The best way to solidify your Python 3 knowledge is through hands-on projects. Create a simple text-based game, build a web application, analyze a dataset, or automate everyday tasks. The possibilities are endless!
Conclusion
Congratulations on your exciting journey into the world of Python 3! Through this illustrated guide, you’ve learned the fundamentals of Python’s elegant syntax, grasped essential concepts like data types, variables, conditional statements, and loops, and even delved into creating functions and modularizing your code.
Remember, programming is a skill that flourishes with practice, so keep coding, exploring, and embracing the versatility of Python 3. As you embark on your projects, you’ll witness your newfound expertise turning ideas into reality through the art of coding.