Images play a pivotal role in creating captivating impressions, and Django, the high-level Python web framework, offers a robust tool for convenient image management – the ImageField.
What is ImageField?
ImageField is a field type provided by the Django web framework, a sophisticated Python framework used for web application development. Specifically designed for handling and managing image files within a Django model, which represents a database table. In simpler terms, it’s a way to store images as part of your application’s data and use them on web pages.
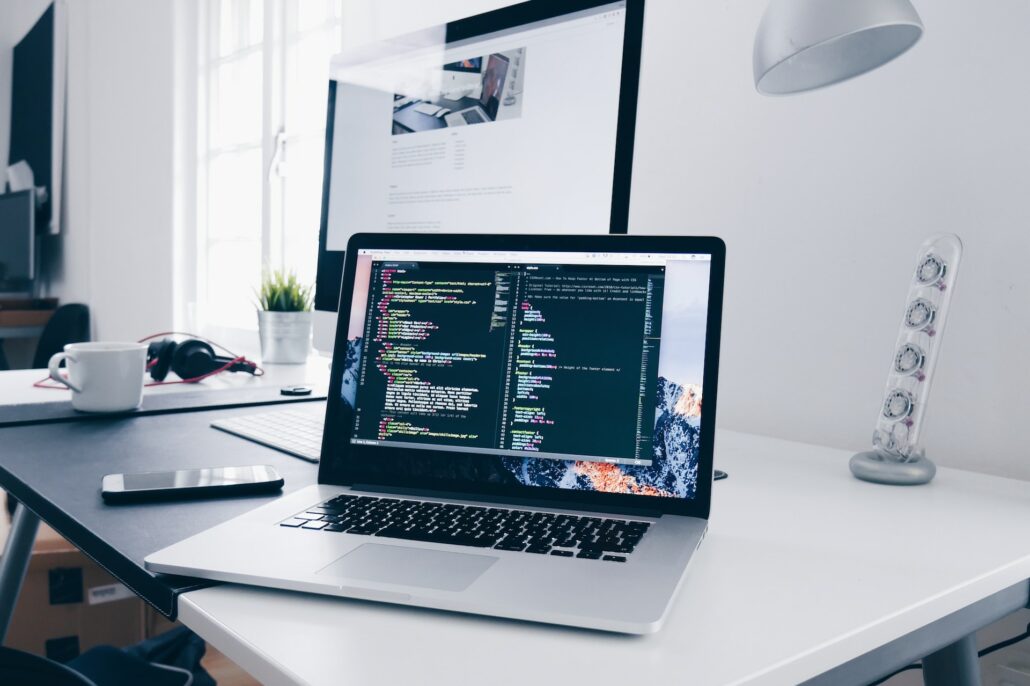
When creating a model (database table), you can define various fields to store different types of data such as text, numbers, dates, and images. This is where the ImageField function comes to the rescue, allowing you to add a field for image file storage to the model. When users upload pictures through your site, Django’s ImageField takes care of the file uploading process, storing the picture in a designated location, and managing associated metadata.
Upload Images in Django
Uploading a picture using ImageField in Django involves several steps, but it’s quite straightforward. Here’s a step-by-step guide on how to do it:
- Configure Your Project: Ensure your project is created and running. If you’re new to Django, you can create a new project using the following command: django-admin startproject projectname;
- Create a Model with an ImageField: Open the models.py file of the application where you want to add pictures uploading functionality. Define a model with an ImageField. For example, let’s create a model named ImageModel in an app named myapp:
python
from django.db import models
class ImageModel(models.Model):
image = models.ImageField(upload_to='images/')
In this example, the upload_to parameter specifies the directory in your media storage where uploaded pictures will be stored.
Configure Media Settings: Open your project's settings.py file and ensure you've configured media settings. Add the following lines:
python
MEDIA_URL = '/media/'
MEDIA_ROOT = os.path.join(BASE_DIR, 'media')
This tells Django where to store and serve your media files, including pictures.
Create and Apply Migrations: Run the following commands to create migrations and apply them to update the database schema:
bash
python manage.py makemigrations myapp
python manage.py migrate
Create a Form: You need a form that allows users to upload pictures. Create a new file named forms.py in your app:
python
from django import forms
from .models import ImageModel
class ImageForm(forms.ModelForm):
class Meta:
model = ImageModel
fields = ['image']
Create a View: In your app’s views.py file, create a view to handle pictures uploading. This view will display the form and process the uploaded picture:
python
from django.shortcuts import render
from .forms import ImageForm
def upload_image(request):
if request.method == 'POST':
form = ImageForm(request.POST, request.FILES)
if form.is_valid():
form.save()
else:
form = ImageForm()
return render(request, 'upload_image.html', {'form': form})
Create a Template: Create an HTML template named upload_image.html to display the image upload form:
html
<form method="post" enctype="multipart/form-data">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Upload Image</button>
</form>
Configure URLs: In your app’s urls.py file, create a URL route to link to the view:
python
from django.urls import path
from .views import upload_image
urlpatterns = [
path('upload/', upload_image, name='upload_image'),
]
Start the Server: Launch the development server:
bash
python manage.py runserver
Navigate to http://127.0.0.1:8000/upload/ in your browser to see the image upload form.
That’s it! You’ve successfully set up image uploading using ImageField in Django. Now users can upload images that will be stored in the specified media directory, and their metadata will be saved in the database.
How to Upload Images in the Django REST Framework?
Uploading images in Django REST framework involves creating a serializer, views, and URL configuration.
Here’s a step-by-step guide:
Configure Your Django Project: Ensure you have a project and Django REST framework installed:
bash
pip install djangorestframework
Create a Model: Create a model with an ImageField:
python
from django.db import models
class ImageModel(models.Model):
image = models.ImageField(upload_to='images/')
Create a Serializer: In serializers.py, create a serializer for ImageModel:
python
from rest_framework import serializers
from .models import ImageModel
class ImageSerializer(serializers.ModelSerializer):
class Meta:
model = ImageModel
fields = ('image',)
Create a View: In views.py, create a view using the serializer:
python
from rest_framework.parsers import FileUploadParser
from rest_framework.response import Response
from rest_framework.views import APIView
from rest_framework import status
from .models import ImageModel
from .serializers import ImageSerializer
class ImageUploadView(APIView):
parser_classes = (FileUploadParser,)
def post(self, request):
serializer = ImageSerializer(data=request.data)
if serializer.is_valid():
serializer.save()
return Response(serializer.data, status=status.HTTP_201_CREATED)
else:
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
Configure URLs: In urls.py, configure a URL route:
python
from django.urls import path
from .views import ImageUploadView
urlpatterns = [
path('upload/', ImageUploadView.as_view(), name='image_upload'),
]
python
urlpatterns = [
path('upload/', ImageUploadView.as_view(), name='image-upload'),
]
URL Configuration:
Add the following code to your project’s main urls.py file to include a URL pattern for serving media files during development:
python
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
# ... other URL patterns ...
]
if settings.DEBUG:
urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
This configuration enables Django to serve media files while your application is in debug mode.
Using Image URLs in Templates
To display uploaded pictures in templates, use the url attribute of the ImageField in your model. Assuming you have a model called ImageModel with an image field, here’s how you can use it in a template:
html
<img src="{{ image_model_instance.image.url }}" alt="Uploaded Image">
Replace image_model_instance with an actual instance of your model containing the uploaded picture.
Starting the Development Server:
Start the development server:
bash
python manage.py runserver
Now, when you visit a page that contains the above image tag, the uploaded picture will be loaded and displayed on the page.
Remember that serving media files using the Django development server is not recommended for production environments. For efficient and secure multimedia file handling, consider setting up a dedicated web server (e.g., Nginx or Apache).
By following these steps, you’ll be able to upload and display pictures in your Django application.
Conclusions
Using Django’s ImageField significantly simplifies working with pictures in web applications. Its ability to handle picture uploads, automatic thumbnail generation, and optimized rendering make it an essential tool for enhancing the visual appeal of your projects.
Effective integration of ImageField and adherence to best practices will enable you to create web applications that not only operate smoothly but also engage users with compelling visual content.