Encountering an issue like this: “TypeError: list indices must be integers or slices, not str”? Fear not, for it merely suggests that an incorrect data type (specifically, a string) has been utilized as an index for a list element, instead of the required integer. This error often arises when attempting to access a specific element within a list using a non-integer value, which can be quite perplexing for those new to programming.
- To shed light on this situation, let’s delve into the intricacies of why this error occurs and how to address it effectively. Python uses indices to locate items within a list, with each item assigned a unique numerical identifier. This identifier, akin to an ID, aids in referencing and accessing list items. The initial item in a list bears an index of 0, while the subsequent items are assigned ascending integers;
- However, when a non-integer, such as a string, is used as an index to retrieve a list item, Python interprets this as an inappropriate operation, leading to the emergence of the “TypeError: list indices must be integers or slices, not str” error message.
In essence, this error highlights the disparity between the data type used for indexing and the expected data type, which should invariably be an integer. Rest assured, this challenge is not insurmountable. By grasping the concepts and strategies elucidated in this context, one can swiftly rectify the “TypeError: list indices must be integers or slices, not str” error and forge ahead with programming tasks more confidently.
Understanding the Occurrence of TypeError: list indices must be integers or slices, not str
When Does the TypeError Occur?
Encountering an issue like this: “TypeError: list indices must be integers or slices, not str”? Fear not, for it merely suggests that an incorrect data type (specifically, a string) has been utilized as an index for a list element, instead of the required integer. This error often arises when attempting to access a specific element within a list using a non-integer value, which can be quite perplexing for those new to programming.
- To shed light on this situation, let’s delve into the intricacies of why this error occurs and how to address it effectively. Python uses indices to locate items within a list, with each item assigned a unique numerical identifier. This identifier, akin to an ID, aids in referencing and accessing list items. The initial item in a list bears an index of 0, while the subsequent items are assigned ascending integers;
- Each item within a Python list possesses an index – a numerical representation denoting its position in the list, akin to an ID. These indices are employed to select specific list items or sequences thereof. The initial list item holds an index of 0, followed by 1, 2, and so forth, culminating in the final item;
- However, when a non-integer, such as a string, is used as an index to retrieve a list item, Python interprets this as an inappropriate operation, leading to the emergence of the “TypeError: list indices must be integers or slices, not str” error message.
In essence, this error highlights the disparity between the data type used for indexing and the expected data type, which should invariably be an integer. Rest assured, this challenge is not insurmountable. By grasping the concepts and strategies elucidated in this context, one can swiftly rectify the “TypeError: list indices must be integers or slices, not str” error and forge ahead with programming tasks more confidently.
Indexes and Their Corresponding Values
- Index 0: ‘Steve’;
- Index 1: 18;
- Index 2: {‘age’: 18, ‘name’: ‘Steve’};
- Index 3: 500.
Notably, these indices are strictly integers, identified as <class ‘int’> when evaluated using the type() function. The error, “TypeError: list indices must be integers or slices, not str,” arises when a non-integer data type is used to access a list item. This post will address strategies to rectify this error.
Diving into the Resolution of TypeError: list indices must be integers or slices, not str
Identifying the Cause of the Error
The error message itself contains vital information to swiftly pinpoint the source of the issue and resolve it. A detailed example demonstrates this:
Error:
“`python
Traceback (most recent call last):
File “file.py”, line 5, in <module>
print(error_words[first_word])
TypeError: list indices must be integers or slices, not str
“`
In this example, Python divulges the faulty file (line ➊) and the specific line (line ➋) contributing to the error. Furthermore, the exact problematic line is displayed (line ➌).
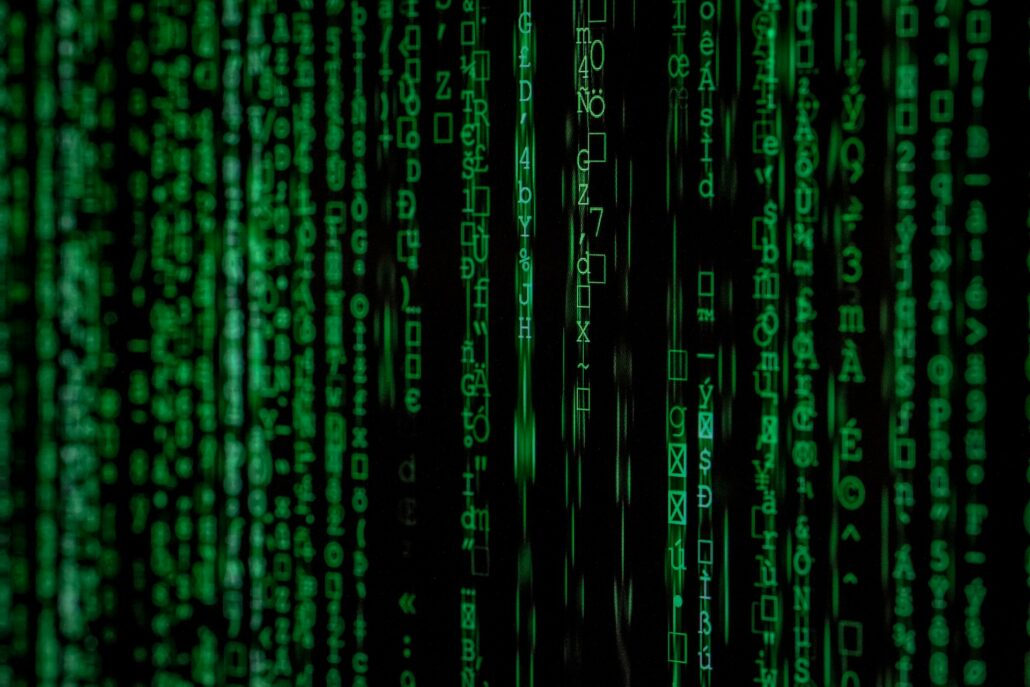
Leveraging this error information alongside the techniques shared in this post enables the efficient resolution of the “list indices must be integers or slices, not str” error.
Instances and Solutions for Fixing the Error
Scenario 1: Unconverted String Variables
Commonly, the error occurs when a string is employed instead of an integer to index a list item. This often involves utilizing a variable containing a string value as an index. An example illustrates this:
Code:
“`python
error_words = [‘list’, ‘indices’, ‘must’, ‘integers’, ‘slices’, ‘not’, ‘str’]
first_word_index = ‘0’
print(error_words[first_word_index])
“`
To rectify this issue, the string-holding index must be converted to an integer, achieved programmatically through the int() function or manually by eliminating the quotes from the string:
Solution Code:
“`python
error_words = [‘list’, ‘indices’, ‘must’, ‘integers’, ‘slices’, ‘not’, ‘str’]
first_word_index = ‘0’
print(error_words[int(first_word_index)])
“`
Scenario 2: Handling Lists of Dictionaries
Novices frequently encounter the error when dealing with lists of dictionaries. This mirrors the previous scenario, wherein a string is erroneously utilized as an index. This arises due to the mistaken treatment of lists as dictionaries.
Considering a list of dictionaries, the error emerges when trying to access a dictionary key using a string index. Correct practice mandates referencing the list item index prior to accessing the dictionary key. Failure to observe this step leads to the familiar “list indices must be integers or slices, not str” error.
Scenario 2, Case 1: Dictionary Referencing in a List
“`python
actors = [
{‘name’: ‘Tom Cruise’, ‘age’: 60},
{‘name’: ‘Arnold Schwarzenegger’, ‘age’: 75},
{‘name’: ‘Adam Sandler’, ‘age’: 56}
]
if actors[‘name’] == ‘Adam Sandler’:
print(‘He is a genius’)
“`
Solution:
“`python
if actors[2][‘name’] == ‘Adam Sandler’:
print(‘He is a genius’)
“`
Scenario 2, Case 2: Looping Over List of Dictionaries
“`python
for actor in actors:
name = actors[‘name’]
age = actors[‘age’]
print(f'{name} is {age} years old’)
“`
Solution:
“`python
for actor in actors:
name = actor[‘name’]
age = actor[‘age’]
print(f'{name} is {age} years old’)
“`
In Conclusion: Addressing the Issue of List Indices Being Integers or Slices, Not Str
Encountering an error like “TypeError: list indices must be integers or slices, not str” presents a common challenge in Python programming. This error message essentially alerts developers that a string value, instead of the expected integer or slice, has been employed as an index to access a list element. It’s crucial to understand that Python requires integer values to navigate and retrieve items from a list.
- This error is not limited to string indices; it’s a representative example of a broader class of issues arising from incorrect data types. The key to resolving such errors lies in comprehending the nature of the data being used as an index and the underlying structure of the data it’s intended to access;
- Thankfully, the solutions provided in the previous paragraphs can be extended to address similar errors caused by other data types. By converting incompatible data types to the correct format or using appropriate methods for the desired operation, you can circumvent these issues and ensure your code functions as intended.
When confronted with such errors, it’s essential to leverage the information provided by the error message itself. The error message specifies the file and line number where the error occurred, helping developers quickly identify and correct the problematic code. This pinpointed approach, combined with a solid understanding of Python’s data types and indexing principles, will empower you to confidently tackle these types of errors and improve your programming skills.