The perplexing Python TypeError, which asserts that “list indices must be integers or slices, not list,” emerges when attempting to employ a list as an index for another list, rather than utilizing an integer or a slice. A remedy for this situation necessitates furnishing an integer or slice as the index, rather than a list. Detecting and resolving the “list indices must be integers or slices, not list” error can be a formidable task, particularly for neophyte Python programmers. This article will elucidate various scenarios in which this error typically manifests.
Illustrative Scenario of the “list indices must be integers or slices, not list” Error
In line with the aforementioned issue, a case in point is provided to facilitate comprehension. Consider the subsequent example:
“`python
list1 = [1, 2, 3]
index_list = [0, 1, 2]
print(list1[index_list])
“`
In the above example, the list `index_list` is employed as an index for `list1`. Regrettably, `index_list` is neither an integer nor a slice, precipitating the emergence of the “list indices must be integers or slices, not list” error upon code execution. To ameliorate this, a solution mandates the provisioning of an integer or slice as the index. For instance:
“`python
list1 = [1, 2, 3]
index_list = [0, 1, 2]
print(list1[index_list[0]]) # Output: 1
“`
This rectified illustration utilizes `index_list[0]`—which evaluates to an integer—as the index for `list1`, successfully printing the initial element, denoted as 1.
Pervasive Scenarios Resulting in the “list indices must be integers or slices, not list” Error
While the foregoing example provided clarity, real-world scenarios aren’t always as straightforward. This section elucidates a predominant circumstance leading to the “list indices must be integers or slices, not list” error: situations encompassing nested lists and loops. Observe the following paradigm:
“`python
a = [[1, 2, 3], [4, 5, 6]]
b = [[7, 8, 9], [10, 11, 12]]
list_a = [a, b]
for i in list_a:
for j in i:
print(list_a[j]) # <— Error occurs here
“`
Upon executing the aforementioned code, the error “list indices must be integers or slices, not list” materializes, pinpointing the exact line harboring `print(list_a[j])`. This predicament arises due to the expression’s outcome being a list. Remedying this entails understanding how to resolve the “Typeerror: list indices must be integers or slices, not list,” which is elaborated in the ensuing segment.
Strategies to Mitigate the “list indices must be integers or slices, not list” Error
Effecting a resolution for the “Typeerror: list indices must be integers or slices, not list” mandates the utilization of integers or slices as indices, in lieu of lists. When confronted with this error, it signifies the existence of a mistake within the code that must be identified and rectified. Consider the following prospective solution:
“`python
a = [[1, 2, 3], [4, 5, 6]]
b = [[7, 8, 9], [10, 11, 12]]
list_a = [a, b]
for i in list_a:
for j in i:
print(j)
“`
Depending on the desired outcome, this approach could provide a viable solution. Alternatively, if the anticipated result remains elusive, adopting an alternative data structure, such as a dictionary, might yield better results. It is essential to differentiate this error from the “list indices must be integers or slices, not str” error, as the former involves supplying a list as an index, as opposed to a string.
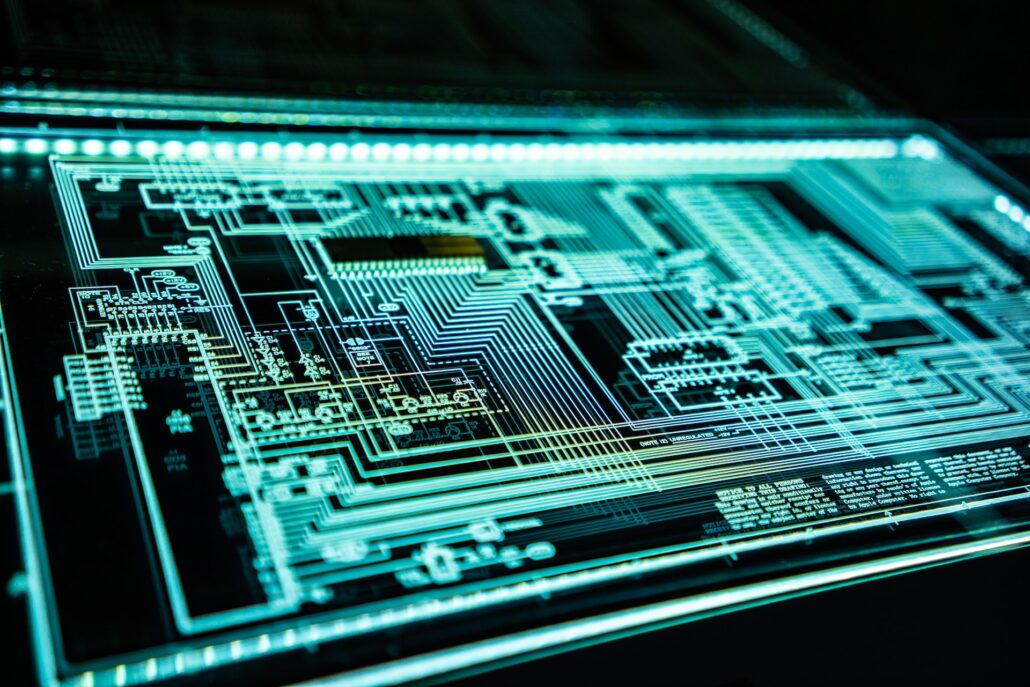
To wrap up
In the realm of Python programming, the enigmatic “list indices must be integers or slices, not list” error stands as a formidable challenge, particularly for those traversing the early stages of their coding journey. This predicament arises when an attempt is made to employ a list as an index for another list, deviating from the expected integer or slice. However, unraveling this conundrum becomes feasible with a deeper understanding of the underlying causes and effective remedial strategies.
As elucidated, the error’s complexity is not limited to straightforward examples. Nested lists and loops can complicate matters, leading to a cascade of confusion and a frustration that plagues developers of varying skill levels. Nevertheless, the solutions presented above offer a beacon of hope. By replacing the incorrect usage of list indices with appropriate integers or slices, programmers can circumvent this common pitfall.
In conclusion, the journey to mastery in Python programming involves grappling with challenges like the “list indices must be integers or slices, not list” error. Embracing these hurdles fosters growth and a more comprehensive understanding of the language’s intricacies. Armed with the insights provided in this article, programmers can navigate through this specific obstacle, advancing not only their coding abilities but also their problem-solving skills. Through perseverance and continuous learning, Python enthusiasts can conquer such roadblocks, emerging as more adept and confident developers in the ever-evolving landscape of programming.