In the intricate realm of web development, ensuring that the structure of your database evolves coherently with your application’s needs is paramount. This is where Django, one of the most popular web frameworks for Python, offers a powerful toolset through its migrations feature. Whether you’re just starting out or are seeking to enhance your proficiency, understanding migrations in Django can be the linchpin for smooth database operations and hassle-free deployments. Welcome to our “Django Migrations Tutorial”, where we will demystify the process of making migrations, walk you through the steps, and shed light on best practices. Dive in, and watch your expertise in Django database management soar!
Understanding Migrations in Django
In the vast ecosystem of Django, a popular web framework written in Python, migrations play a vital role. These are mechanisms that allow developers to alter their project’s database schema so that it mirrors the evolving structure of Django models. But that’s not all. With Django’s robust framework, developers also have the flexibility to revert these alterations if the situation demands it. The fundamental objective of migrations is to ensure that there’s a consistent alignment between the database and the Django models.
Why Migrations Matter: Delving Deeper into their Significance
Safe and Structured Alterations: One of the hallmarks of Django migrations is the provision of a safe haven for making changes. Whether tweaking models or adjusting the database schema, these migrations ensure that changes occur in a structured and controlled environment.
- Comprehensive Change Tracking: In any development project, tracking alterations can be as crucial as making them. With migrations, not only can developers keep tabs on shifts in the model and database schema, but they can also gain insights into the historical evolution of their database. This clarity provides a strategic advantage when the need arises to reconstruct or revert the database to a former state;
- Collaborative Development Sans Conflicts: In the world of collaborative development, avoiding conflicts, especially those related to databases, can be challenging. Enter Django’s migration framework. It’s ingeniously designed to allow multiple developers to simultaneously craft their own sets of migrations. This way, each contributor can progress without inadvertently stepping on their peers’ toes, ensuring a smoother development flow;
- Efficient Deployment Across Environments: Deploying a Django project across varied environments often comes with its set of challenges. With migrations, there’s no need to build the database from scratch every time. Instead, developers can seamlessly deploy their projects, ensuring that the underlying database adjusts appropriately to different environments. This approach not only simplifies the deployment process but also amplifies its reliability.
In conclusion, migrations in Django aren’t just a feature; they’re an indispensable asset. They not only streamline the development and deployment processes but also bolster the robustness and resilience of Django projects. Whether it’s about making iterative changes, tracking them, collaborating without conflicts, or deploying across diverse environments, migrations ensure that developers stay in the driver’s seat, steering their Django projects towards success.
Constructing a Migration
To establish a migration through Django’s “makemigrations” function, adhere to the subsequent procedures:
Initiation: Update Your Django Models
Firstly, ensure you’ve thoroughly updated your Django models within the models.py document. These modifications might encompass the addition of a fresh model or field, altering an existing field’s type, or eliminating a field.
Proceed to the Second Phase: Activate the makemigrations Function
Within your Django project’s terminal, execute the “makemigrations” function. While this command can be utilized without any specifications to generate migrations for the entire project, it’s advisable to tailor migrations to individual apps. Consequently, indicate the specific app you’re targeting for the migration. As an illustration:
$ python manage.py makemigrations myapp
Upon successful execution, a fresh migration document will emerge within the “migrations” folder of the “myapp” app, encompassing the essential directives for both the application and reversal of your model modifications.
Digging Deeper: Supplementary Commands
For a more tailored migration procedure, consider these additional command enhancements:
1. Selective Migration:
Though one might introduce several alterations to the models.py (like model addition, field modifications, or removals), there might be times you’d want to isolate a migration to a specific modification. The optimal method? Initiate an unpopulated migration document, combining the –empty and –name options. Once this file is produced, you can manually embed the requisite migration code for that specific alteration.
For instance:
$ python manage.py makemigrations myapp –name delete_title_model –empty
This will birth a blank migration file named “delete_title_model”. You can then manually input the necessary adjustments. For a clearer perspective, peruse other migration documents to familiarize yourself with the code structure.
2. Aggregating Multiple App Changes:
Desire to merge alterations from all apps into a singular migration? Employ the –merge command. For instance:
$ python manage.py makemigrations –merge
This will spawn a consolidated migration document, outlining instructions for applying and retracting modifications spanning all apps.
Congratulations! You’ve now crafted a migration via Django’s “makemigrations” command. The next logical step? Implement these migrations onto the database.
Executing Migrations in Django
To execute a migration using Django’s migrate tool, consider the following guidelines:
Initiating the Migration:
Launch the migration by indicating the desired application name. For instance:
$ python manage.py migrate myapp
This action will process any outstanding migrations specific to the ‘myapp’ application. An outstanding migration refers to any migration that hasn’t been integrated into the database.
Tailored Commands:
For Specific Migrations:
If there’s a need to execute a distinct migration, simply identify it by its filename. As an illustration:
$ python manage.py migrate myapp 0001_initial
This will execute the ‘0001_initial’ migration for the ‘myapp’ application.
For Universal Migration:
To integrate all migrations across every application, utilize the –all argument, as shown:
$ python manage.py migrate –all
This encompasses all outstanding migrations from all applications.
Reversing a Migration:
The –backward argument serves to reverse migrations. Here’s how:
$ python manage.py migrate –backward
This reverses the most recent migration made to the ‘myapp’ application.
Dealing with Multiple Databases:
If your Django setup involves several databases, there might be occasions when specific migrations need to be directed to a particular database. The –database flag comes in handy here:
$ python manage.py migrate myapp –database=mydatabase
This processes the outstanding migrations for ‘myapp’ in the ‘mydatabase’ database. It’s worth noting that the –database flag can be combined with others, such as:
$ python manage.py migrate myapp 0001_initial –database=mydatabase
This transfers the initial migration file’s changes to the mentioned database.
For All Applications:
$ python manage.py migrate –database=mydatabase –all
This ensures migrations from all applications are directed to the given database.
To Revert a Specific Migration:
$ python manage.py migrate myapp –database=mydatabase –backward
This reverts the most recently processed migration for ‘myapp’ in ‘mydatabase’.
If your Django setup involves a singular database, there’s no need to specify the –database flag as Django defaults to that.
You’ve now adeptly navigated the nuances of Django’s migrate tool. As a best practice, always commit your database modifications to your version control system, ensuring consistency across different setups.
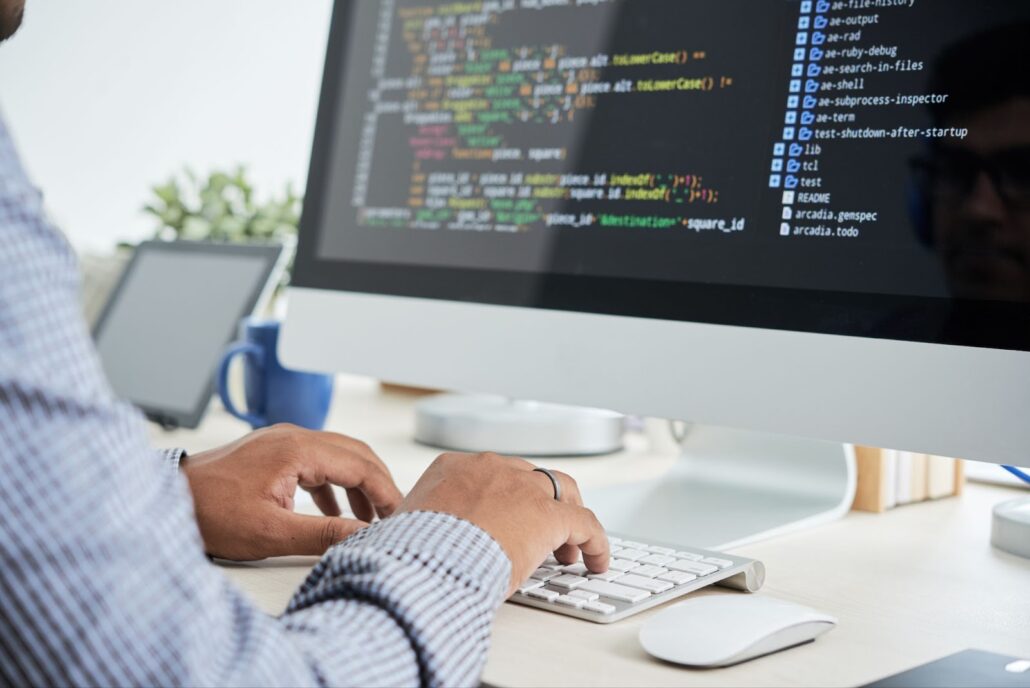
FAQ
In Django, migrations are essential components for handling changes in your database schema. Migrations allow you to manage the changes to your models over time, and they enable you to evolve your database schema in a controlled and consistent manner. Here are some reasons why saving Django migrations is crucial:
1. Version Control of Database Schema: When working with a team, it’s essential to know which state the database is in. Migrations serve as a version control for your database schema, allowing different team members to apply the same changes to their local databases.
2. Data Integrity and Transformation: Sometimes, model changes can’t just adjust the schema but also require data transformations. Migrations allow you to script both schema alterations and data migrations to maintain consistency and data integrity.
3. Rollbacks: If a particular migration introduces an issue, you can rollback to a previous migration. Without the migration files, this becomes difficult or even impossible.
4. Deployment: When you’re deploying your Django project, migrations ensure that the production database schema matches what your application expects. Without migrations, you would need some other method to ensure the production database is correctly structured.
5. Clarity: Having a history of migrations provides a clear path of how your database schema has evolved over time. This can be helpful when onboarding new developers or for future reference.
6. Dependencies between migrations: Sometimes migrations in one app might depend on migrations in another app. Without saving and managing these migration files, applying migrations can become chaotic.
However, there are some caveats:
1. Don’t edit migrations after they’ve been applied: After a migration has been applied and especially if it’s been distributed to other team members or deployed, you shouldn’t modify it. If you need to make a change, create a new migration.
2. Source Control: Always include migrations in your version control system (e.g., Git). This ensures that everyone working on the project has access to the necessary migrations.
Executing Django migrations is an essential part of maintaining the structure and integrity of your database as your application evolves. However, the timing and environment in which you execute migrations is crucial to ensure smooth transitions and minimize disruptions.
Here are some general guidelines about when and how to execute Django migrations:
1. Development Phase: Run migrations frequently to ensure that your database schema is always in sync with your models. After creating a new migration file (using python manage.py makemigrations), immediately apply it using python manage.py migrate.
2. Version Control: Always commit migrations to your version control system (like Git). This ensures that all developers on the team have the same migration history. When pulling changes from a shared repository, always check for new migrations and apply them to your local database.
3. Staging Environment: Before deploying to production, always test migrations in a staging or a pre-production environment that mirrors your production environment as closely as possible. This will help you catch potential issues.
4. Production Environment: Schedule the deployment for a time when the application has the least traffic or during maintenance windows. This minimizes potential disruptions to users. Always backup your production database before applying migrations. Ensure that you have a rollback strategy in case something goes wrong. This might involve reverting the migration or restoring from a backup. Run migrations in a sequential and consistent order. Avoid skipping migrations. Monitor the application after applying migrations to ensure everything works as expected.
5. Data Migrations: If a migration involves large data changes or complex operations, consider splitting it into multiple smaller migrations. Test data migrations thoroughly in a staging environment to ensure data integrity.
6. Collaboration: When working in a team, coordinate with team members to ensure migrations are applied in the right order and that there are no conflicting migrations.
7. Long Running Migrations: For very large databases, some migrations (like adding a new column with a default value) might take a significant amount of time. Plan these migrations carefully and consider techniques like zero-downtime deployments.
Conclusion
In summary, mastering Django migrations stands out as a paramount tool vital for managing and sustaining a Django project’s database. This exceptional utility grants you the ability to modify model designs and database configurations coherently and with the option of reversal. Moreover, it ensures a synchronized relationship between your database and the active Django models.
By diligently following the detailed guidelines presented in this discourse, you can effortlessly create and implement migrations using Django’s “makemigrations” and “migrate” functions. Whether navigating the complexities of a small endeavor or partnering in a comprehensive joint project, Django migrations consistently assist in safeguarding your database’s current status, all under the umbrella of version management.
write a meta title. for an article “MAIN KEYWORD”character limit is 60 characters including spaces. use keyword “MAIN KEYWORD” only once.
write a H1 for an article “MAIN KEYWORD”. character limit is 60 characters including spaces. use keyword “MAIN KEYWORD” only once. you can rephrase this keyword.
Your task is to rewrite the entire text in better words and make it unique with natural language. Rewrite the text using a unique and highly varied sentence structure The text to rewrite it is this:
Rewrite and rephrase the text sections below in a unique and creative way and expand each of them. Add more information in each section. Write in a more informative and engaging way. Make each section detailed, comprehensive, and valuable as much as possible for readers. Include useful bullet lists, recommendations, tips, or insights where possible. Do not write from the first-person perspective. Do not copy any words or texts from the text below or from any other texts, articles, or sites. Use only your own words.
write a creative and catchy meta Description for an article “MAIN KEYWORD”. character limit is 150 characters including spaces. use keyword “MAIN KEYWORD” only once.