Welcome to a comprehensive guide on how to leverage the potential of if statements in Django templates. As a web developer, understanding the intricacies of conditional logic is paramount for creating dynamic and responsive web applications. In this article, we will delve deep into the world of if statements, exploring their syntax, use cases, and practical implementation. Whether you’re a novice or an experienced developer, this guide will equip you with the knowledge to wield if statements with finesse.
Exploring the Basics of if Statements
If Statements in Django Templates: Simplifying Dynamic Content Rendering
Conditional statements lie at the heart of programming. In Django templates, if statements act as decision-makers, enabling you to control the display of content based on certain conditions. Let’s begin by examining the fundamental syntax of an if statement:
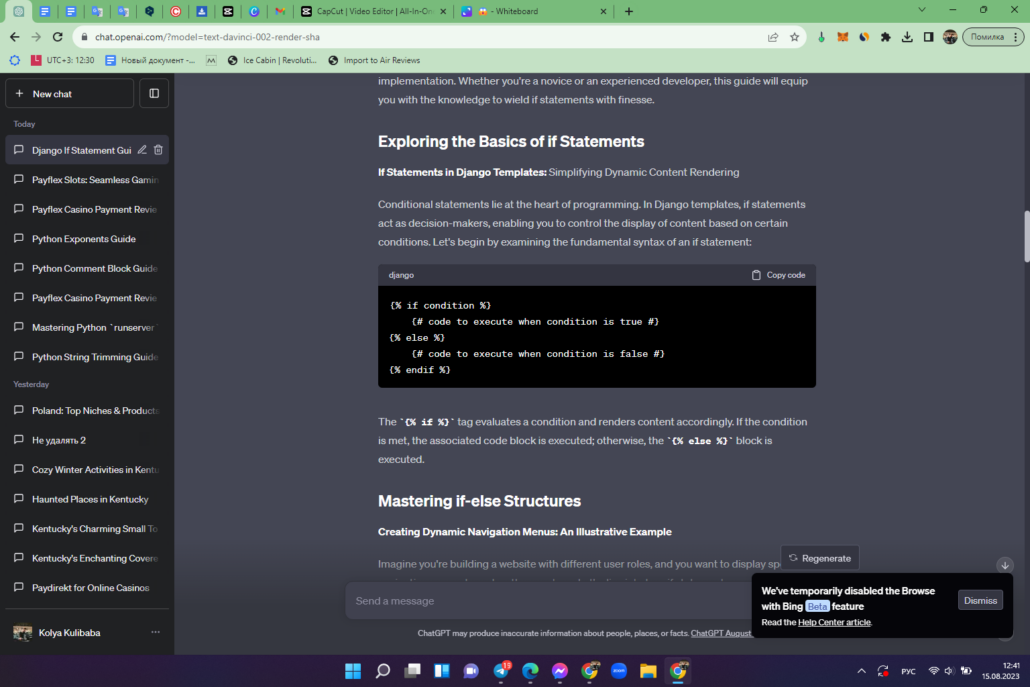
The {% if %} tag evaluates a condition and renders content accordingly. If the condition is met, the associated code block is executed; otherwise, the {% else %} block is executed.
Mastering if-else Structures
Creating Dynamic Navigation Menus: An Illustrative Example
Imagine you’re building a website with different user roles, and you want to display specific navigation menus based on the user type. Let’s dive into how if statements can help achieve this:
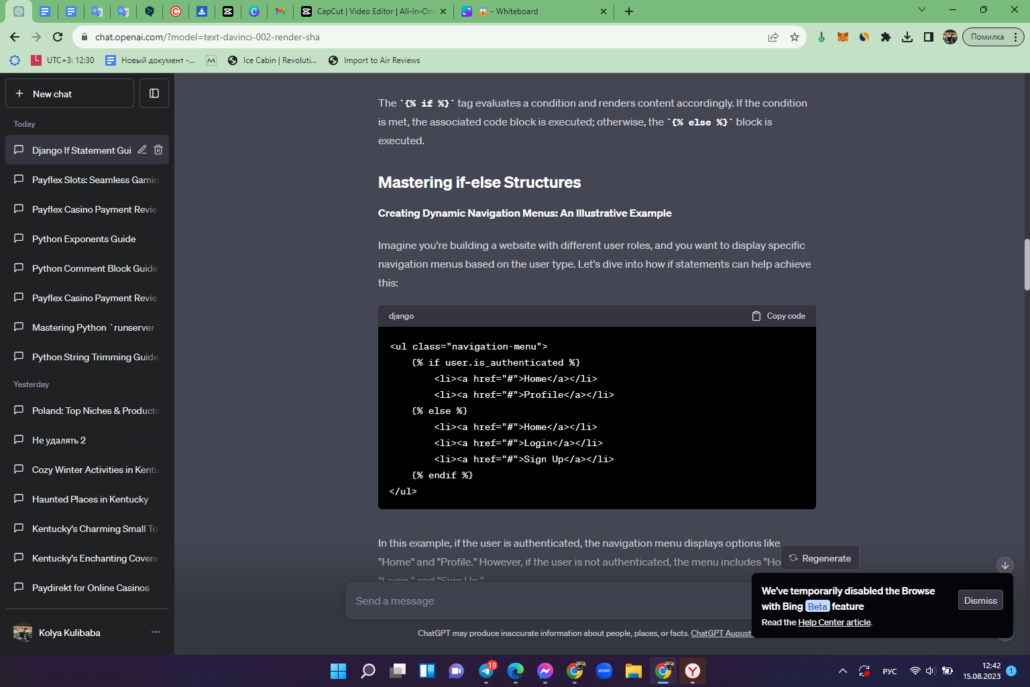
In this example, if the user is authenticated, the navigation menu displays options like “Home” and “Profile.” However, if the user is not authenticated, the menu includes “Home,” “Login,” and “Sign Up.”
Unlocking the Power of Filters with if Statements
Django templates offer a plethora of built-in filters that complement the functionality of if statements. Filters enable you to manipulate and format data before rendering it. Let’s consider an example involving date comparison:
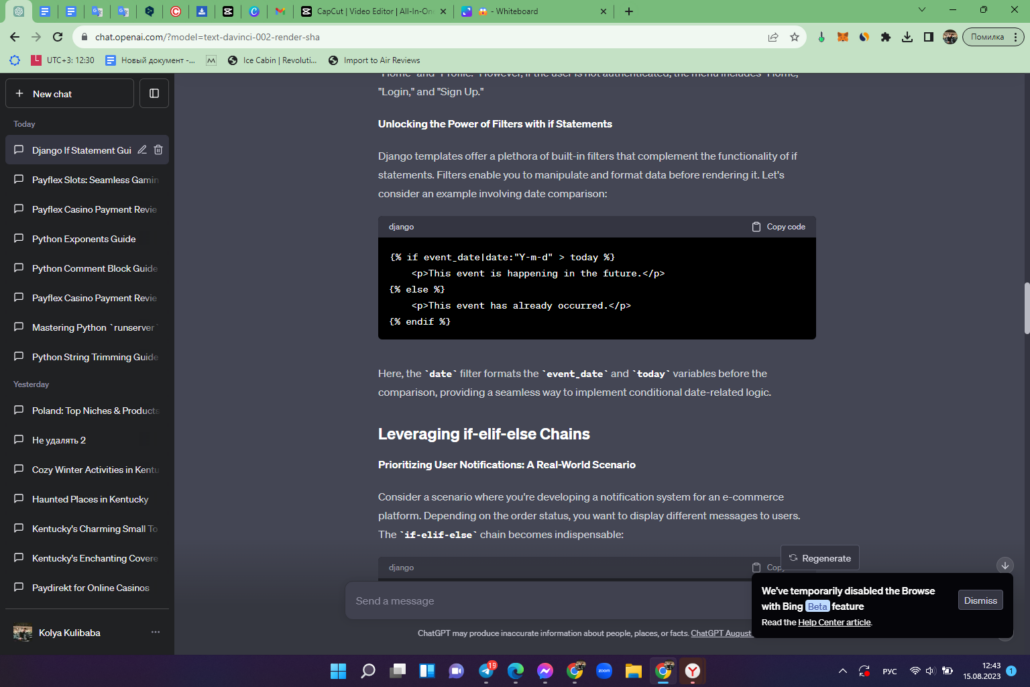
Here, the date filter formats the event_date and today variables before the comparison, providing a seamless way to implement conditional date-related logic.
Leveraging if-elif-else Chains
Prioritizing User Notifications: A Real-World Scenario
Consider a scenario where you’re developing a notification system for an e-commerce platform. Depending on the order status, you want to display different messages to users. The if-elif-else chain becomes indispensable:
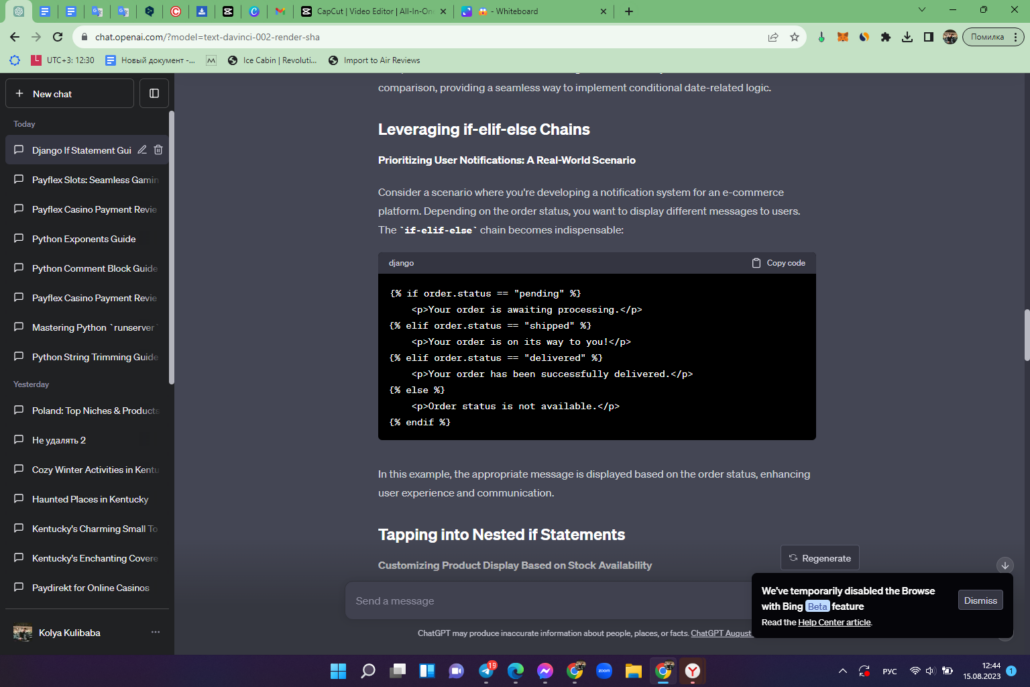
In this example, the appropriate message is displayed based on the order status, enhancing user experience and communication.
Tapping into Nested if Statements
Customizing Product Display Based on Stock Availability
When dealing with complex scenarios, nesting if statements can be remarkably effective. Let’s explore a case where you’re building an online store and need to display product availability based on stock levels:
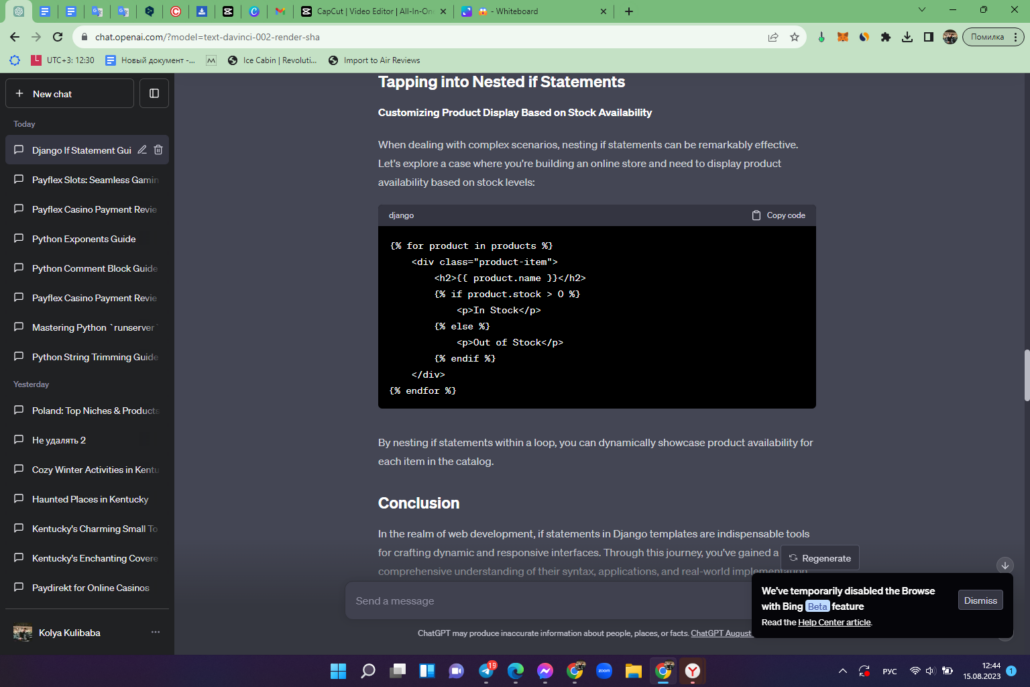
By nesting if statements within a loop, you can dynamically showcase product availability for each item in the catalog.
Diving into Advanced if Statement Techniques
Utilizing the and and or Operators for Complex Conditions
Sometimes, conditions require multiple factors to be evaluated. This is where the and and or operators come into play. Let’s explore an example where both operators are employed to create intricate conditions:
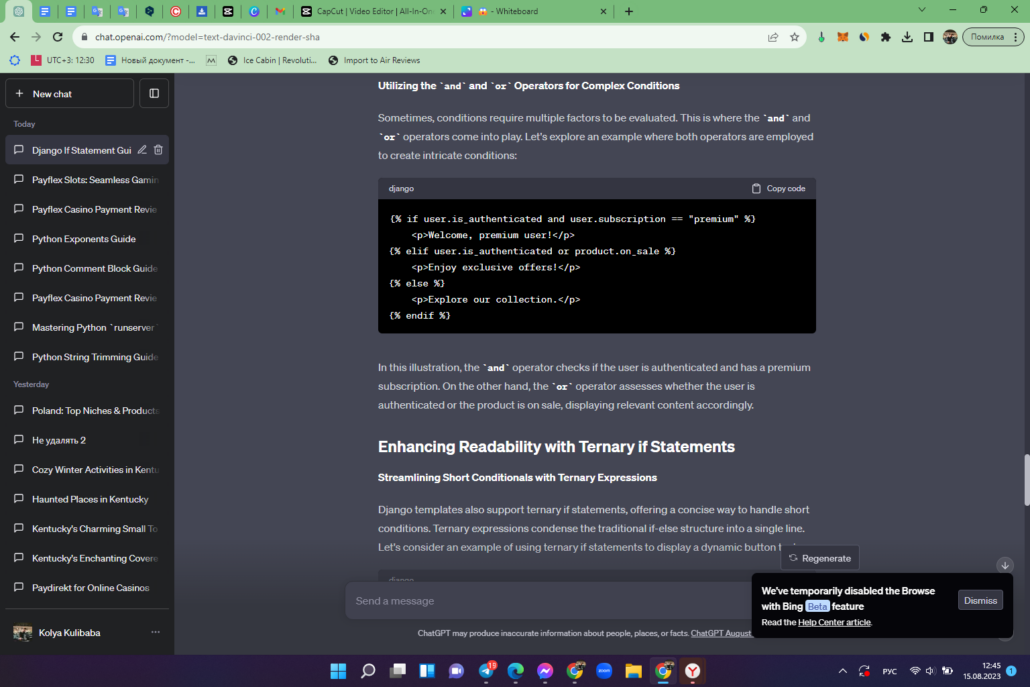
In this illustration, the and operator checks if the user is authenticated and has a premium subscription. On the other hand, the or operator assesses whether the user is authenticated or the product is on sale, displaying relevant content accordingly.
Enhancing Readability with Ternary if Statements
Streamlining Short Conditionals with Ternary Expressions
Django templates also support ternary if statements, offering a concise way to handle short conditions. Ternary expressions condense the traditional if-else structure into a single line. Let’s consider an example of using ternary if statements to display a dynamic button text:
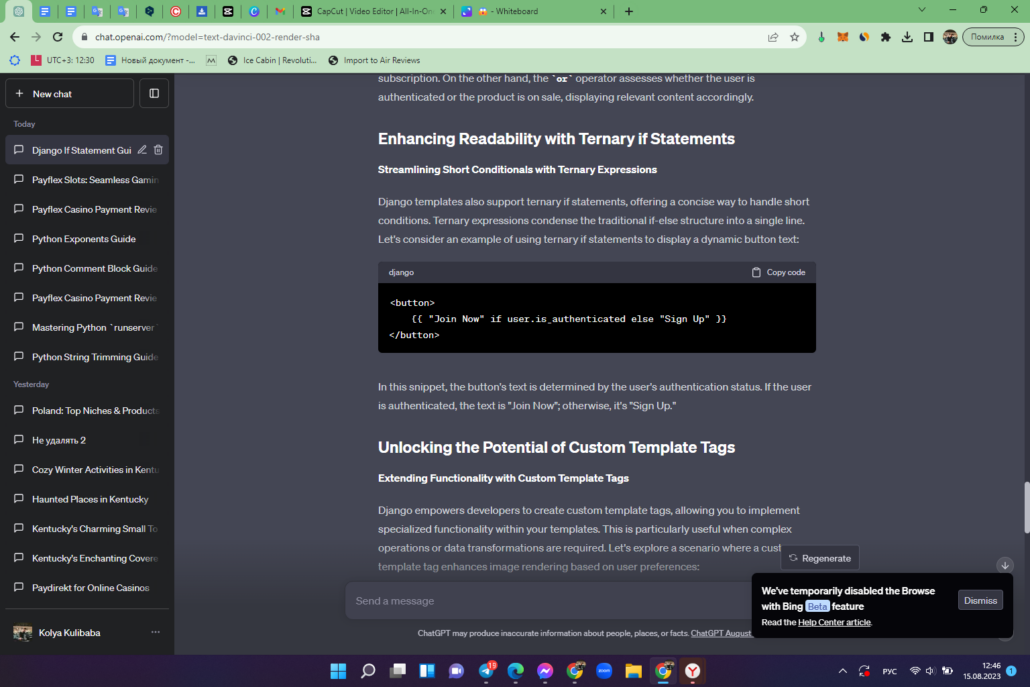
In this snippet, the button’s text is determined by the user’s authentication status. If the user is authenticated, the text is “Join Now”; otherwise, it’s “Sign Up.”
Unlocking the Potential of Custom Template Tags
Extending Functionality with Custom Template Tags
Django empowers developers to create custom template tags, allowing you to implement specialized functionality within your templates. This is particularly useful when complex operations or data transformations are required. Let’s explore a scenario where a custom template tag enhances image rendering based on user preferences:
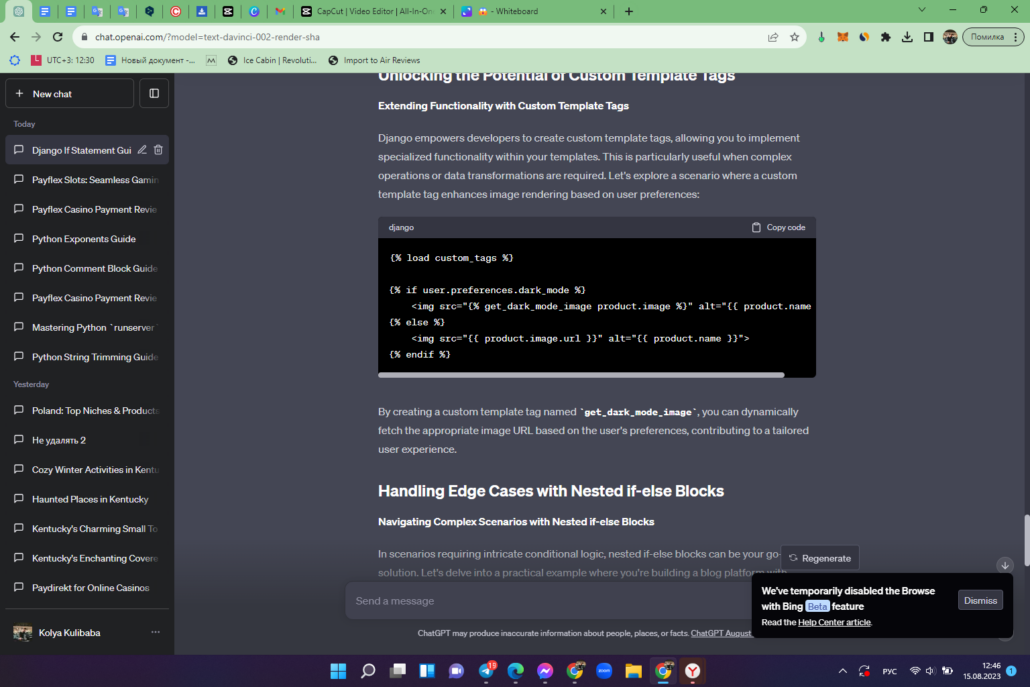
By creating a custom template tag named get_dark_mode_image, you can dynamically fetch the appropriate image URL based on the user’s preferences, contributing to a tailored user experience.
Handling Edge Cases with Nested if-else Blocks
Navigating Complex Scenarios with Nested if-else Blocks
In scenarios requiring intricate conditional logic, nested if-else blocks can be your go-to solution. Let’s delve into a practical example where you’re building a blog platform with varying levels of user access:
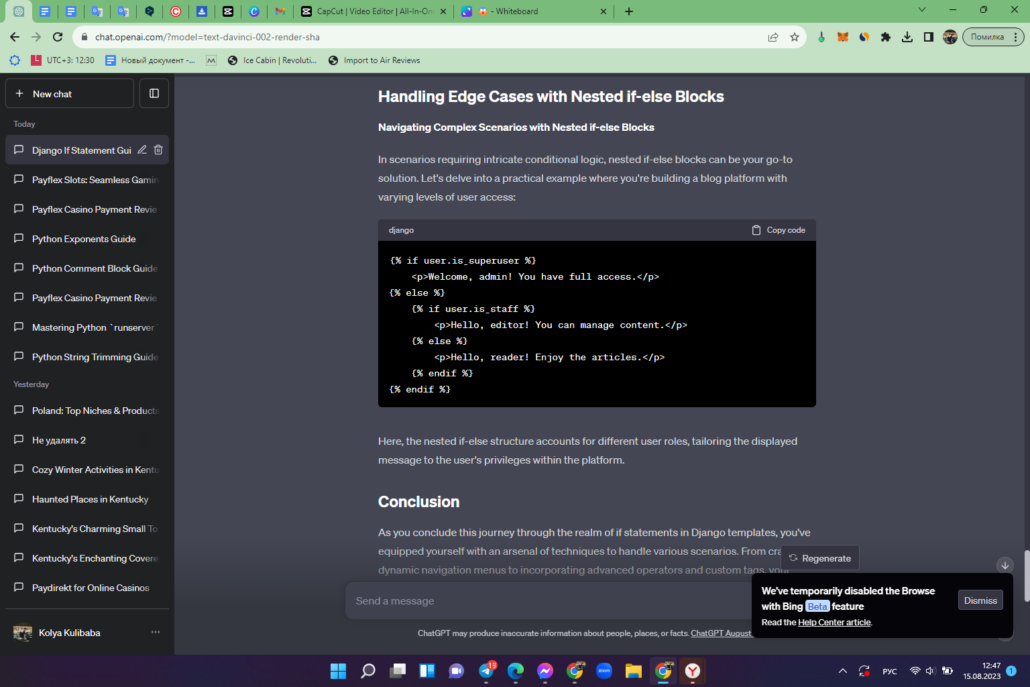
Here, the nested if-else structure accounts for different user roles, tailoring the displayed message to the user’s privileges within the platform.
Conclusion
In the realm of web development, if statements in Django templates are indispensable tools for crafting dynamic and responsive interfaces. Through this journey, you’ve gained a comprehensive understanding of their syntax, applications, and real-world implementation. As you harness the power of if statements, your ability to create tailored user experiences will reach new heights.
FAQs
Yes, you can nest if statements as needed to accommodate complex conditional logic. However, excessive nesting can lead to decreased readability and maintainability.
Yes, Django templates also offer the {% with %} tag and the {% ifchanged %} tag for specific scenarios requiring context management and change detection.
You can combine conditions using logical operators such as and, or, and not to create intricate if statements.
Certainly! Django supports custom template tags, allowing you to extend the functionality of your templates beyond built-in tags like {% if %}.
Ensure your conditions are correctly formulated and variables are properly defined. You can also print debugging information within template blocks for better insights into the evaluation process.