Whether you’re a seasoned Python developer or just starting your coding journey, understanding how to use comment blocks is crucial. Comment blocks not only make your code more readable but also help you and your team grasp the logic behind it.
Introduction to Python Comment Blocks
Commenting your code is like leaving breadcrumbs for fellow programmers who might work on your project in the future. It provides context, explanations, and even warnings about specific code segments. Python comment blocks are essential tools for clear communication within your codebase.
Why Are Comment Blocks Essential?
Comment blocks serve as a lifeline for developers when they revisit their own or others’ code. They offer clarity and understanding, especially for complex algorithms or logic that might not be immediately apparent. Comment blocks act as annotations, helping you remember the purpose of a certain function, variable, or class.
Types of Comment Blocks
Python supports two types of comment blocks: single-line comments and multi-line comments. The syntax for single-line comments is straightforward:
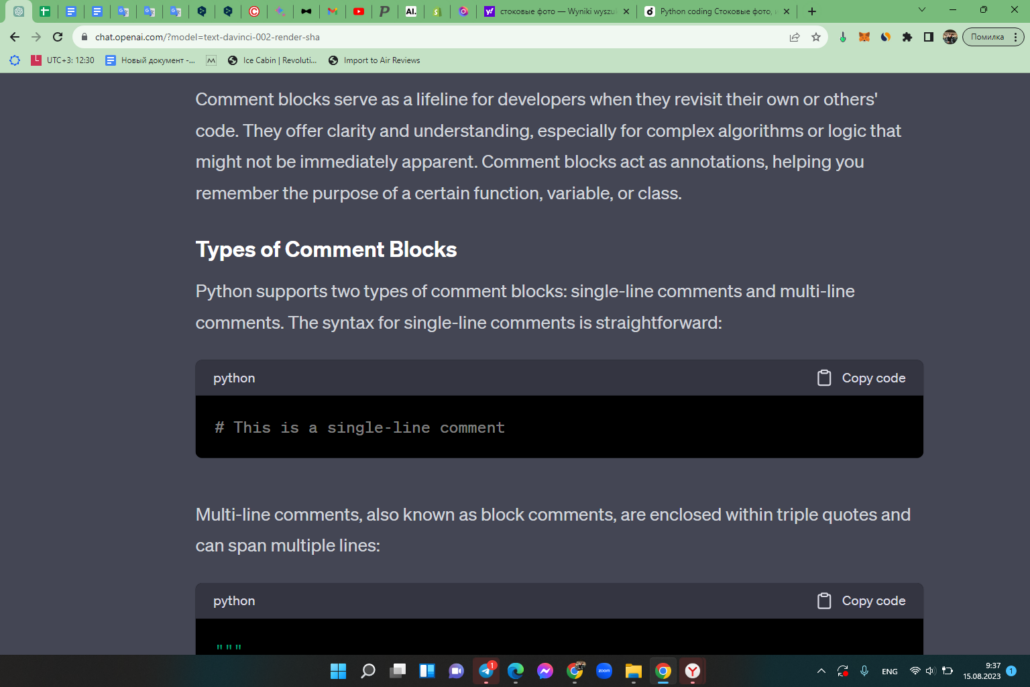
Multi-line comments, also known as block comments, are enclosed within triple quotes and can span multiple lines:
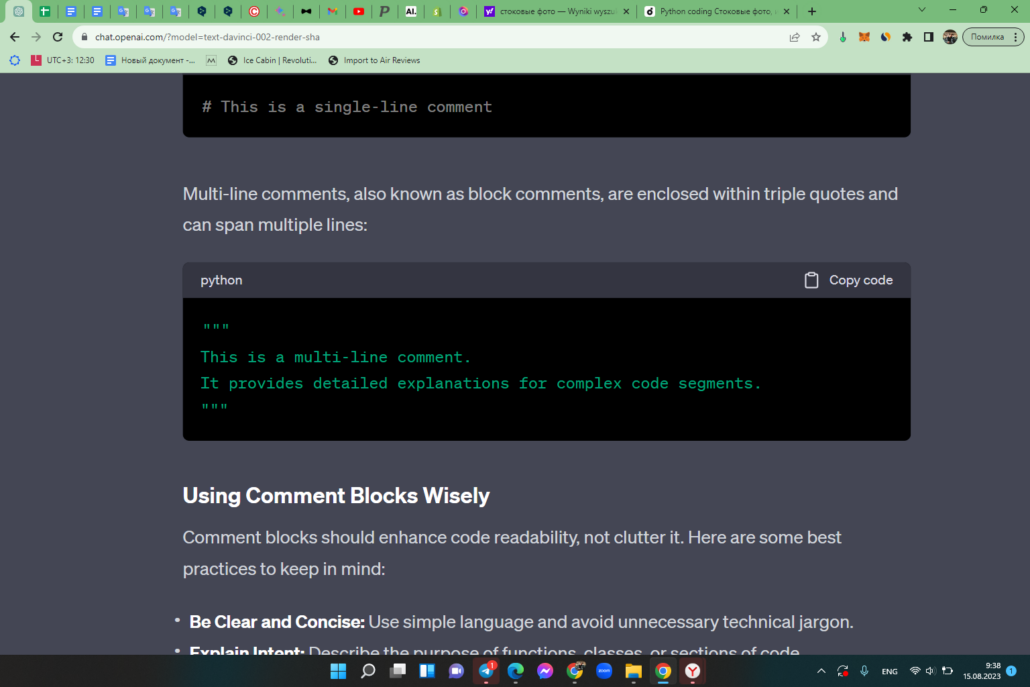
Using Comment Blocks Wisely
Comment blocks should enhance code readability, not clutter it. Here are some best practices to keep in mind:
- Be Clear and Concise: Use simple language and avoid unnecessary technical jargon;
- Explain Intent: Describe the purpose of functions, classes, or sections of code;
- Document Assumptions: If your code relies on certain conditions, mention them in the comment block;
- Update Regularly: As your code evolves, make sure to update the comment blocks accordingly.
Adding Comments to Specific Code Elements
Different code elements require different comment approaches:
- Function Comments: Explain the function’s inputs, outputs, and behavior;
- Class Comments: Describe the role and responsibilities of the class;
- Method Comments: Clarify the purpose of methods and how they contribute to the class’s functionality.
Commenting for Collaborative Projects
In team projects, comment blocks become even more critical. They facilitate collaboration and allow team members to understand each other’s code quickly. When working on a collaborative project:
- Use a Consistent Style: Establish coding and commenting conventions for uniformity;
- Comment Before Writing: Draft comment blocks before writing the code to clarify your approach;
- Review and Edit: Regularly review and update comment blocks during code reviews.
Examples of Effective Comment Blocks
Let’s take a look at a few examples of well-structured comment blocks:
Example 1: Function Comment
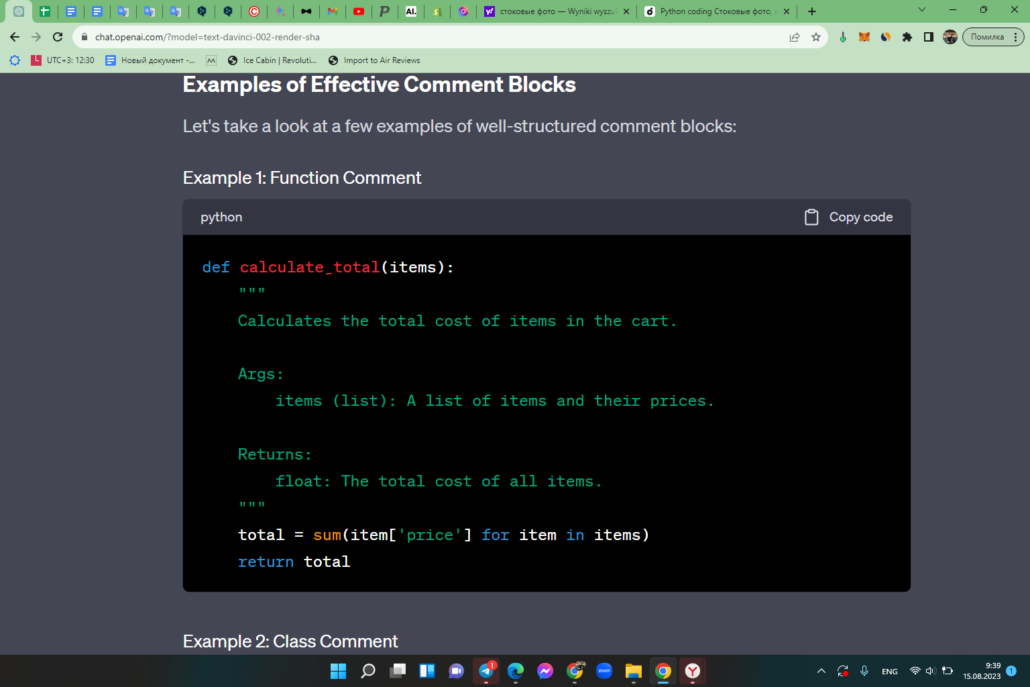
Example 2: Class Comment
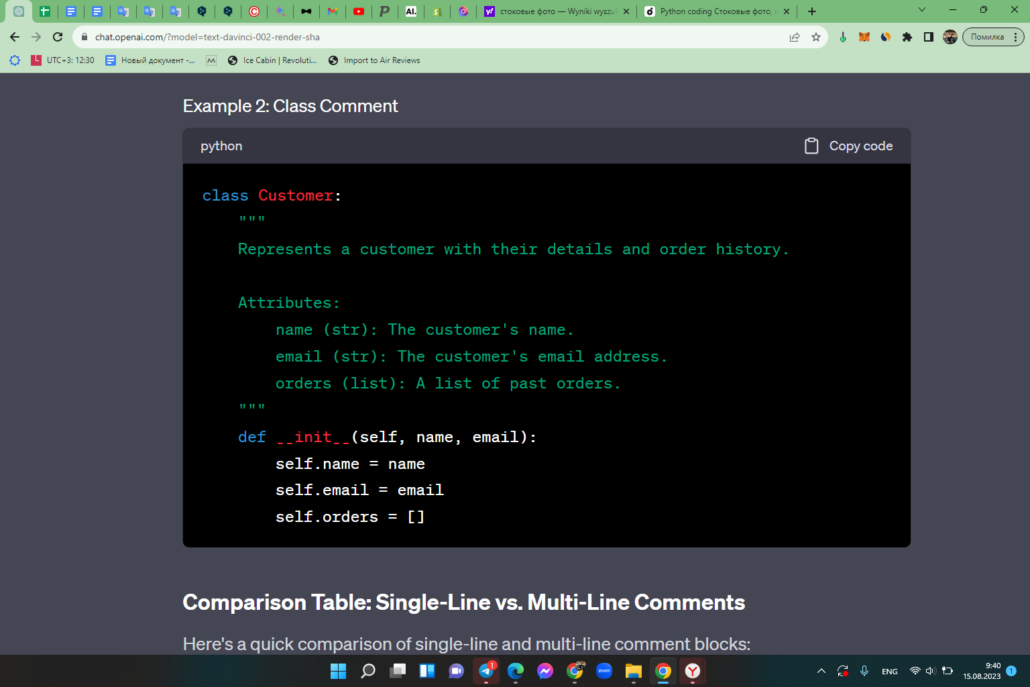
Single-Line vs. Multi-Line Comments
Here’s a quick comparison of single-line and multi-line comment blocks:
Aspect | Single-Line Comment | Multi-Line Comment |
---|---|---|
Syntax | # This is a comment | “””This is a multi-line comment””” |
Applicability | Short explanations | Detailed explanations and context |
Ideal Usage | End of lines | Complex code segments |
Advanced Techniques for Comment Blocks
As you become more proficient in Python coding, you’ll encounter scenarios that demand more advanced comment block techniques. Let’s explore some of these techniques to enhance your coding prowess further.
Inline Comments for Complex Logic
Inline comments are succinct explanations placed directly within a line of code. They’re particularly useful when dealing with intricate logic that might be tough to grasp at first glance. Here’s an example:
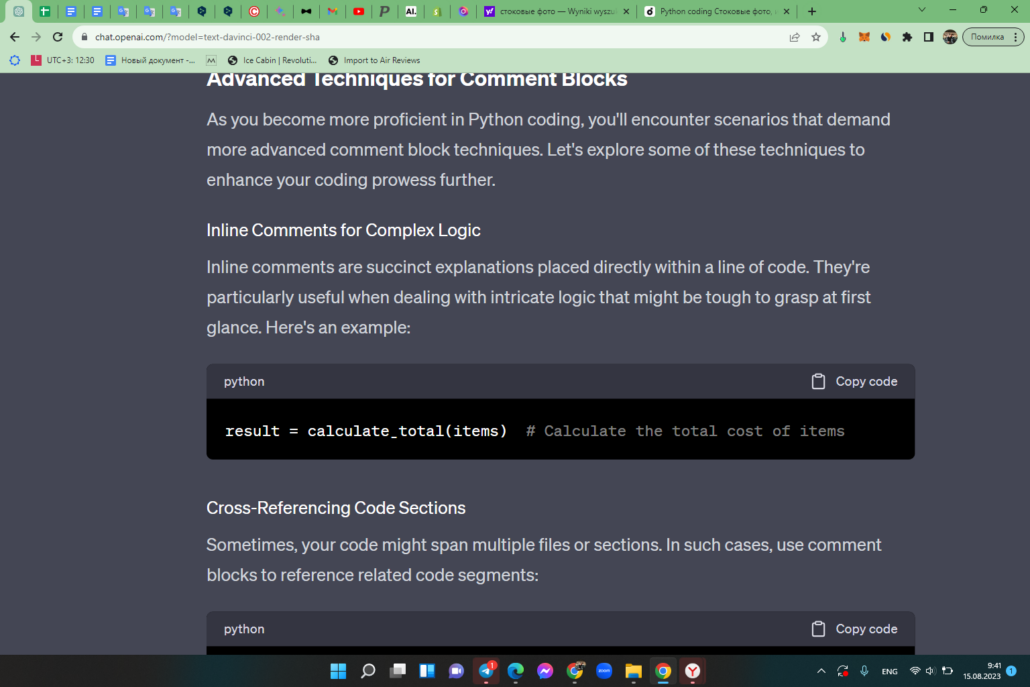
Cross-Referencing Code Sections
Sometimes, your code might span multiple files or sections. In such cases, use comment blocks to reference related code segments:
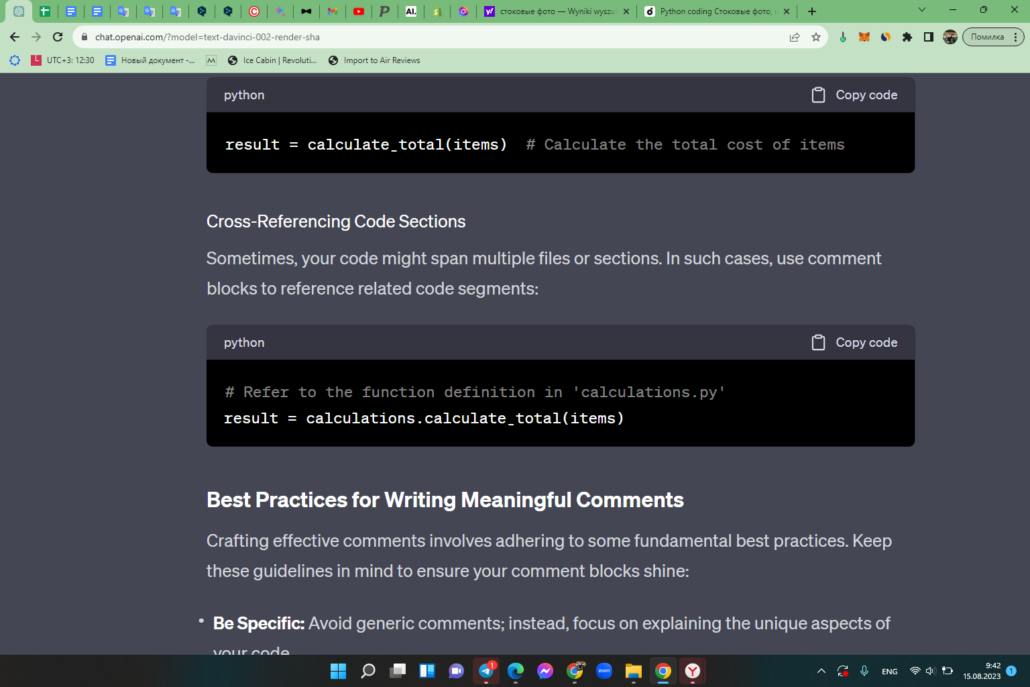
Best Practices for Writing Meaningful Comments
Crafting effective comments involves adhering to some fundamental best practices. Keep these guidelines in mind to ensure your comment blocks shine:
- Be Specific: Avoid generic comments; instead, focus on explaining the unique aspects of your code;
- Avoid Obvious Comments: Don’t state the obvious; your comments should add value by providing insights;
- Update During Refactoring: When you refactor code, don’t forget to update the associated comments;
- Use Clear Formatting: Well-structured comments are easier to read. Use proper indentation and spacing;
- Be Polite and Professional: Your comments reflect your professionalism, even in casual projects.
Leveraging Comment Blocks for Debugging
Comment blocks can play a significant role in the debugging process. They help you track the flow of execution, isolate problematic sections, and document your debugging steps. Here’s how you can employ comment blocks for effective debugging:
1. Flagging Debug Points: Mark specific lines with comments to indicate potential problem areas:
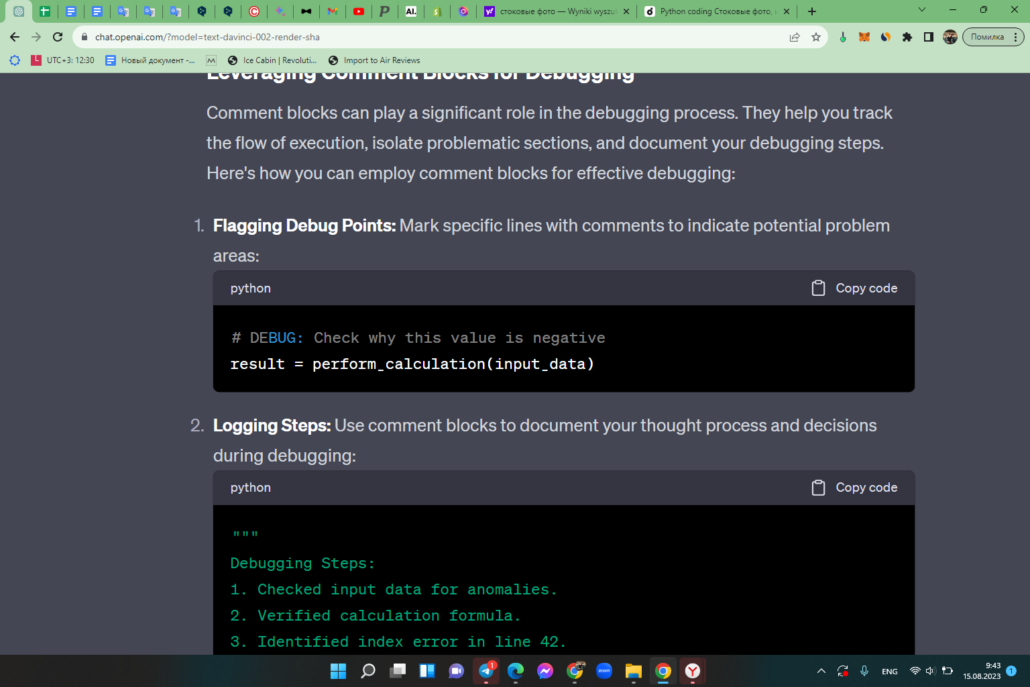
2. Logging Steps: Use comment blocks to document your thought process and decisions during debugging:
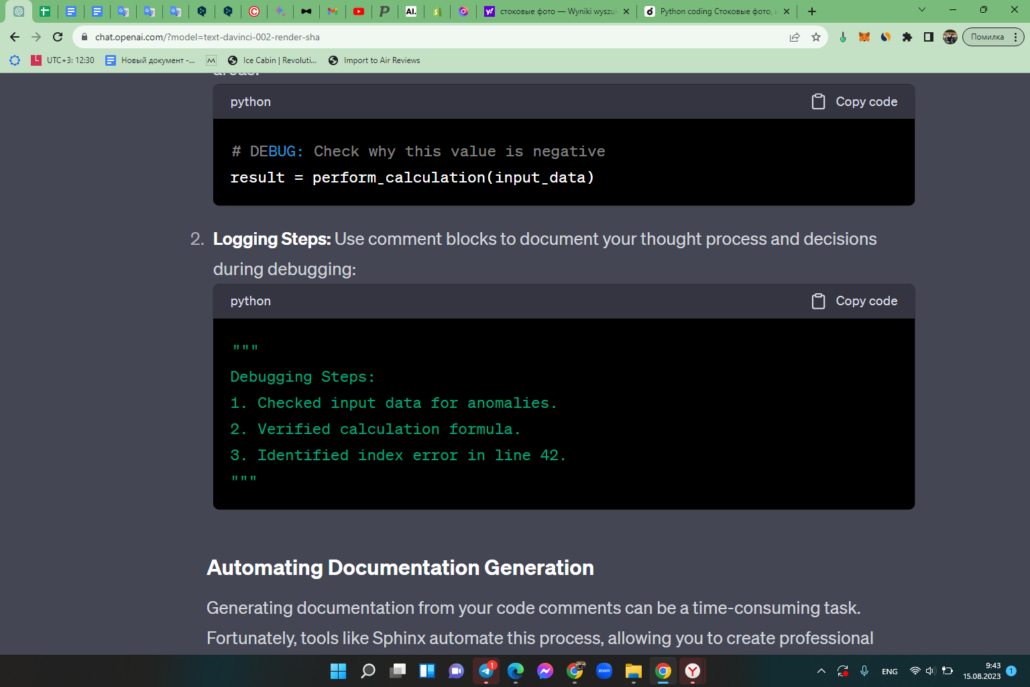
Automating Documentation Generation
Generating documentation from your code comments can be a time-consuming task. Fortunately, tools like Sphinx automate this process, allowing you to create professional documentation effortlessly. Follow these steps to generate documentation using Sphinx:
1. Install Sphinx using pip:
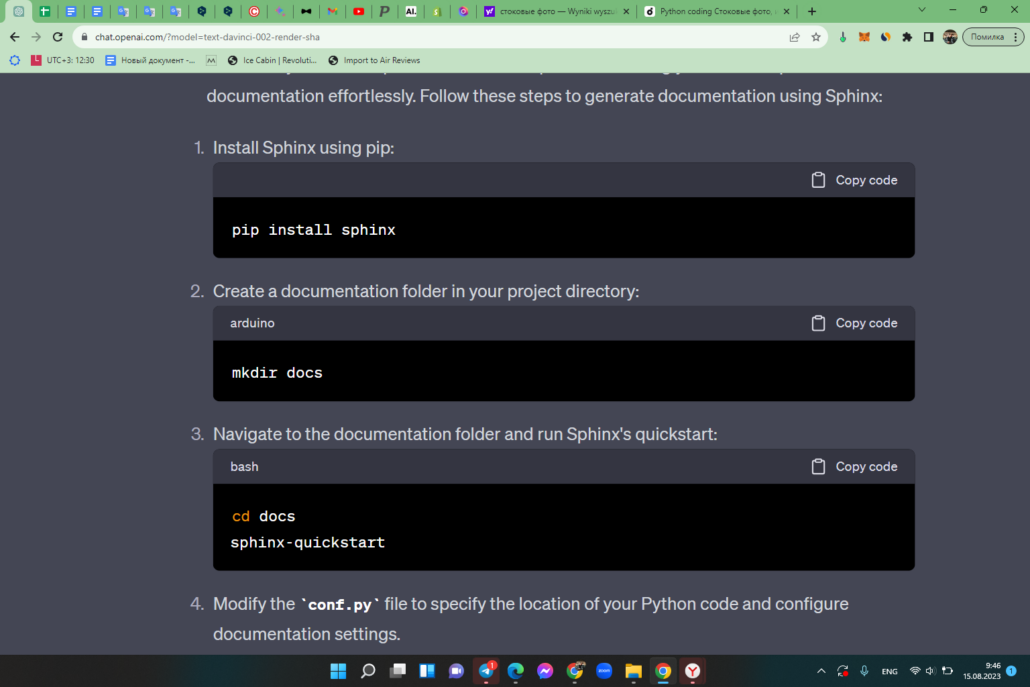
2. Create a documentation folder in your project directory:
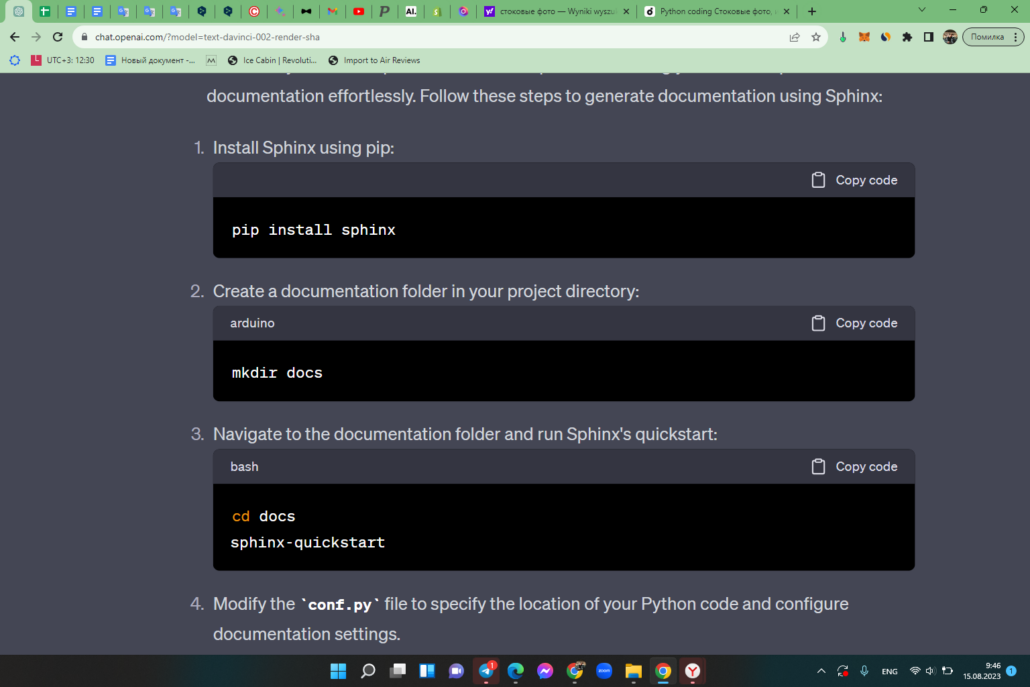
3. Navigate to the documentation folder and run Sphinx’s quickstart:
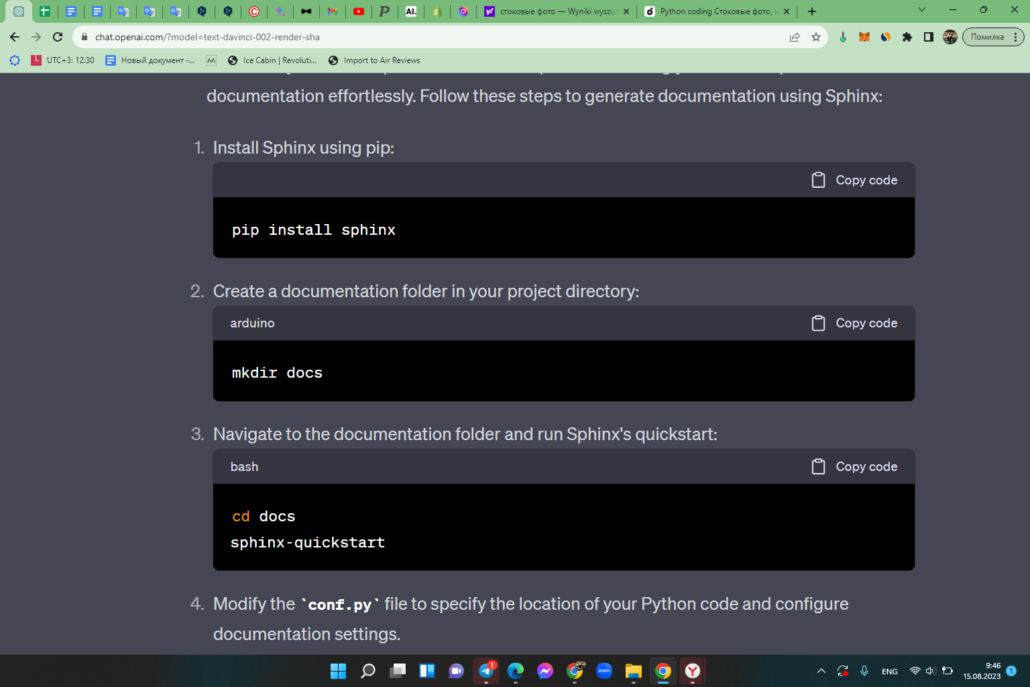
4. Modify the conf.py file to specify the location of your Python code and configure documentation settings.
5. Run the documentation generator:
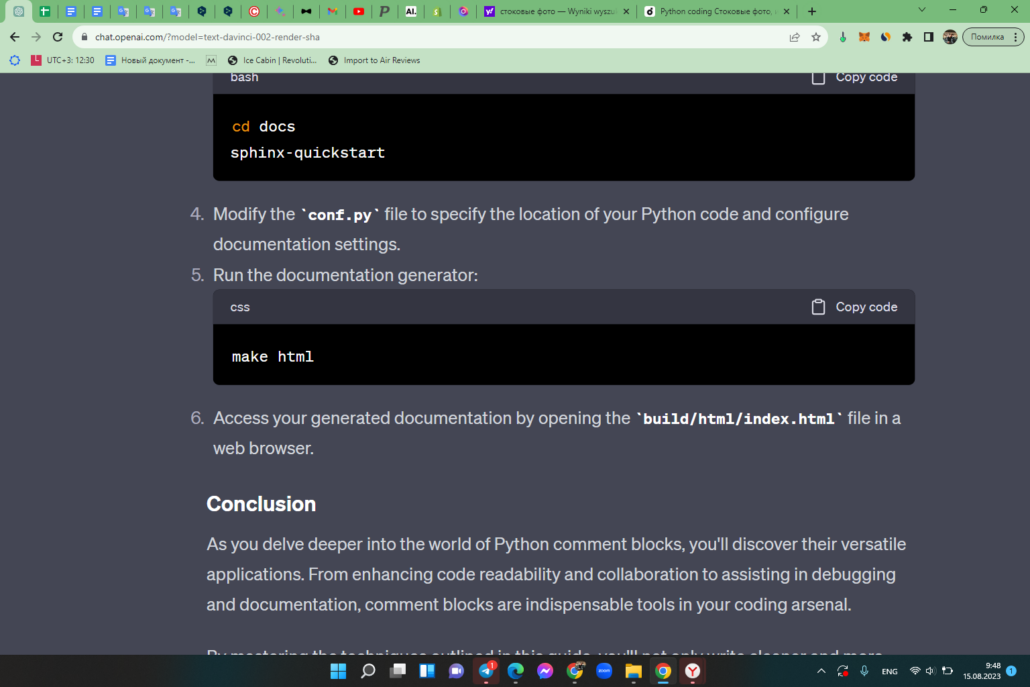
6. Access your generated documentation by opening the build/html/index.html file in a web browser.
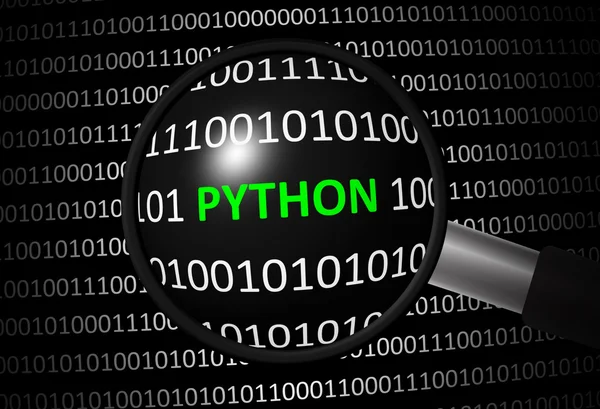
Frequently Asked Questions
No, comments are ignored by the Python interpreter. They’re purely for human readers.
Yes, you can use the block comment syntax (”’ or “””) to comment out multiple lines at once.
Outdated comments can mislead developers. Always update comments when you modify code.
Yes, tools like Sphinx can generate documentation from well-structured comments.
While comments are valuable, excessive commenting can clutter your code. Aim for balance.
Conclusion
Mastering the art of Python comment blocks empowers you to create more understandable and maintainable code. By following the best practices outlined in this guide, you can effectively communicate your code’s intent, even to those who come across it in the distant future.