Python is a versatile programming language that offers a multitude of functionalities for developers. Among its most fundamental features is the ability to define and call functions.
A function is a block of code designed to perform a specific task. In Python, creating and invoking functions is straightforward and significantly enhances code readability and reusability.
How to Define a Function
Defining a function in Python involves two primary steps: declaring the function and specifying its arguments.
To define a function, use the def keyword followed by the function’s name and parentheses. If the function takes arguments, include them within the parentheses. The function’s code block is indented after the colon.
- Example:
def greet(name): print(“Hello, ” + name + “! How are you?”)
In the above example, a function named greet is defined, taking a single argument name. The function prints a greeting message containing the provided name.
How to Call a Function
Once a function is defined, it can be called multiple times within your code.
To call a function in Python, simply write the function’s name followed by parentheses. If the function requires arguments, they should be placed inside the parentheses.
- Example:
greet(“John”)
In this example, the greet function is called with the argument “John,” resulting in the output:
Hello, John! How are you?
Python Function Code Examples
Here’s a complete example demonstrating function definition and invocation:
def greet(name): print(“Hello, ” + name + “! How are you?”) greet(“John”)
Upon running this code, the output will be:
Hello, John! How are you?
Now, let’s explore a more advanced example involving the definition and call of functions in Python.
Suppose you want to create a function that takes a list of integers and returns a new list containing only the even numbers. Here’s how you can define and call this function:
def get_even_numbers(numbers): even_numbers = [] for number in numbers: if number % 2 == 0: even_numbers.append(number) return even_numbers numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = get_even_numbers(numbers) print(even_numbers)
In this example, the function get_even_numbers is defined to take a list of numbers. The function iterates through each number in the list, checking if it’s even. If it is, the number is added to the even_numbers list using the append method. Finally, the function returns the list of even numbers.
When calling the function, a list of numbers is provided, and the returned list of even numbers is printed to the console.
Benefits of Using Functions in Python
- Modularity: Functions allow you to break down complex tasks into smaller, manageable modules;
- Reusability: Once defined, functions can be called multiple times, eliminating code duplication;
- Readability: Functions enhance code readability by encapsulating specific functionality;
- Maintenance: Changes or improvements can be made to a function without affecting the rest of the codebase.
By leveraging Python’s ability to define and call functions, you can write more organized and efficient code, making your programming tasks more manageable and your codebase more maintainable.
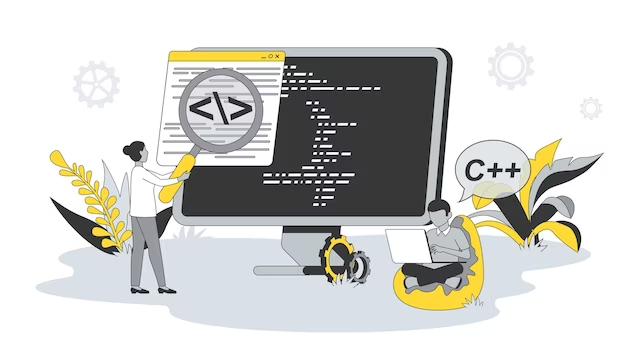
Table: Solutions to Common Function Problems
Mastering functions in Python empowers you to create modular, efficient, and maintainable code. Whether for simple scripts or complex applications, functions are a cornerstone of programming expertise. Start using functions to enhance your coding skills today!
Problem | Solution |
---|---|
No function output | Check function indentation, return statements, and arguments. |
Incorrect argument order | Verify the order of arguments when calling the function. |
Variable scope issues | Understand local and global variable scopes, and avoid naming conflicts. |
Infinite recursion | Ensure proper base case in recursive functions to prevent infinite loops. |
Mismatched parentheses | Check for balanced parentheses when defining and calling functions. |
Incorrect function name | Verify the spelling and case sensitivity of the function name. |
Conclusion
Defining and calling functions in Python is an essential skill for any developer. Functions provide a structured way to organize code, improve reusability, and enhance overall code readability.
With Python’s simple syntax and powerful capabilities, you can define functions with various arguments and execute a wide range of tasks within those functions. Whether you’re writing simple scripts or complex applications, mastering functions is crucial for writing clean, efficient, and maintainable code.
So, embrace the power of functions in Python, and take your coding skills to the next level by creating modular, reusable, and concise code.
FAQ
A function is a block of organized and reusable code designed to perform a specific task. It enhances code readability and reusability by breaking down complex operations into smaller, manageable components.
To define a function, use the def keyword followed by the function name and parentheses. Specify any arguments within the parentheses, and indent the code block after the colon.
To call a function, simply write the function’s name followed by parentheses. If the function requires arguments, provide them within the parentheses.
Yes, a function can return a value using the return statement. This allows the function to provide an output that can be used in further computations.