When working with Python programming, it’s common to encounter situations where you need to check if a list is empty before proceeding with further operations. Whether you’re a beginner or an experienced developer, knowing multiple ways to determine the emptiness of a list can improve your code’s efficiency and readability. In this article, we’ll delve into three distinct approaches to achieve this task effortlessly.
Using the len() Function
One of the simplest ways to determine if a list is empty is by using the built-in len() function. This function returns the length of the list, and an empty list will have a length of 0. By comparing the length to 0, you can easily ascertain whether the list is empty or not.
Example:
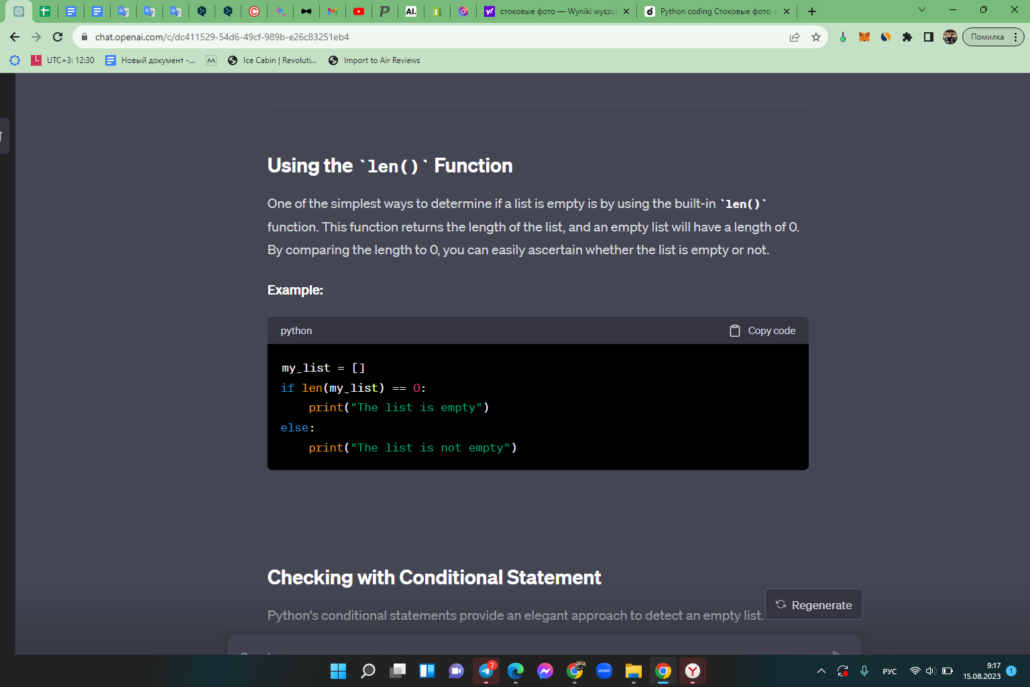
Checking with Conditional Statement
Python’s conditional statements provide an elegant approach to detect an empty list. You can use the truthiness of lists to your advantage. In Python, an empty list evaluates to False in a boolean context, while a non-empty list evaluates to True.
Example:
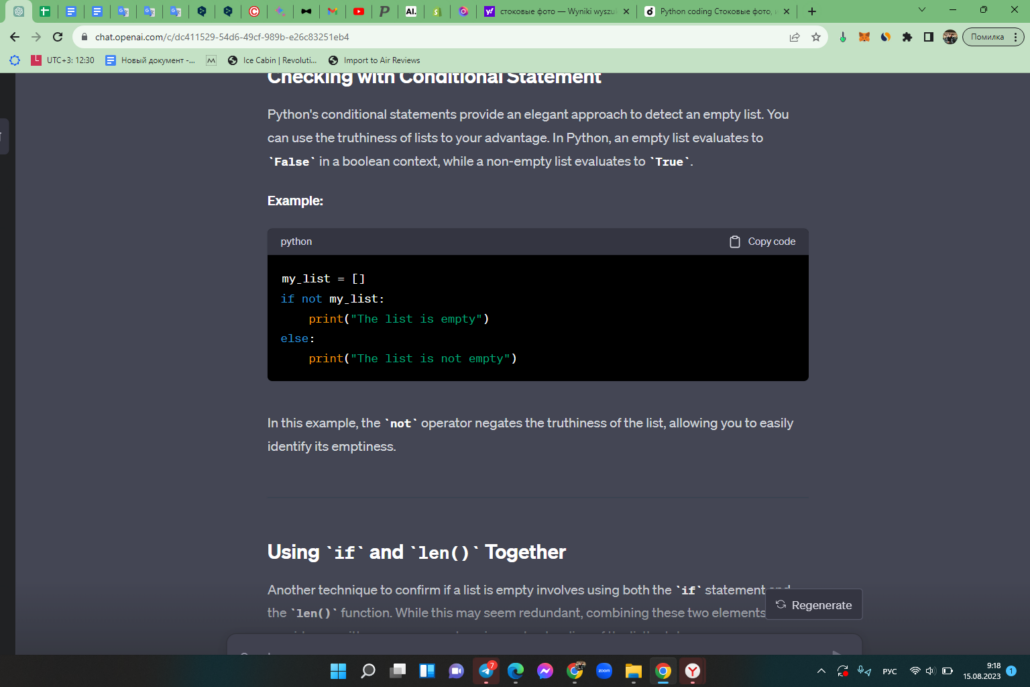
In this example, the not operator negates the truthiness of the list, allowing you to easily identify its emptiness.
Using if and len() Together
Another technique to confirm if a list is empty involves using both the if statement and the len() function. While this may seem redundant, combining these two elements can provide you with a more comprehensive understanding of the list’s state.
Example:
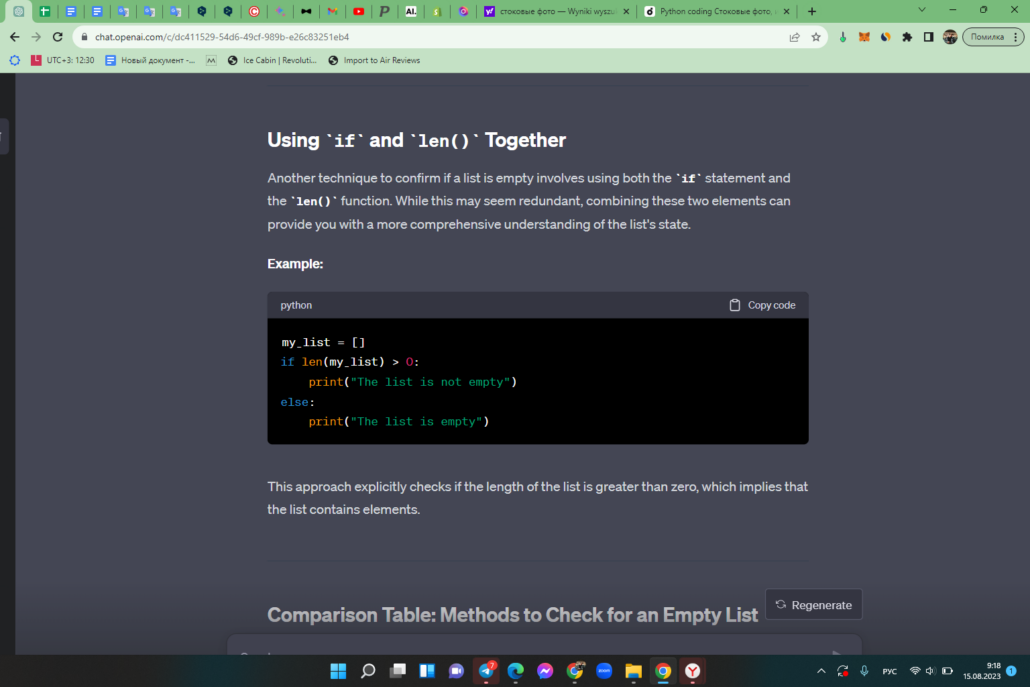
This approach explicitly checks if the length of the list is greater than zero, which implies that the list contains elements.
Using any() and all() Functions
Python provides the versatile any() and all() functions that can be employed to examine the contents of a list and determine its emptiness. The any() function returns True if at least one element in the iterable is True, and the all() function returns True if all elements are True.
Example:
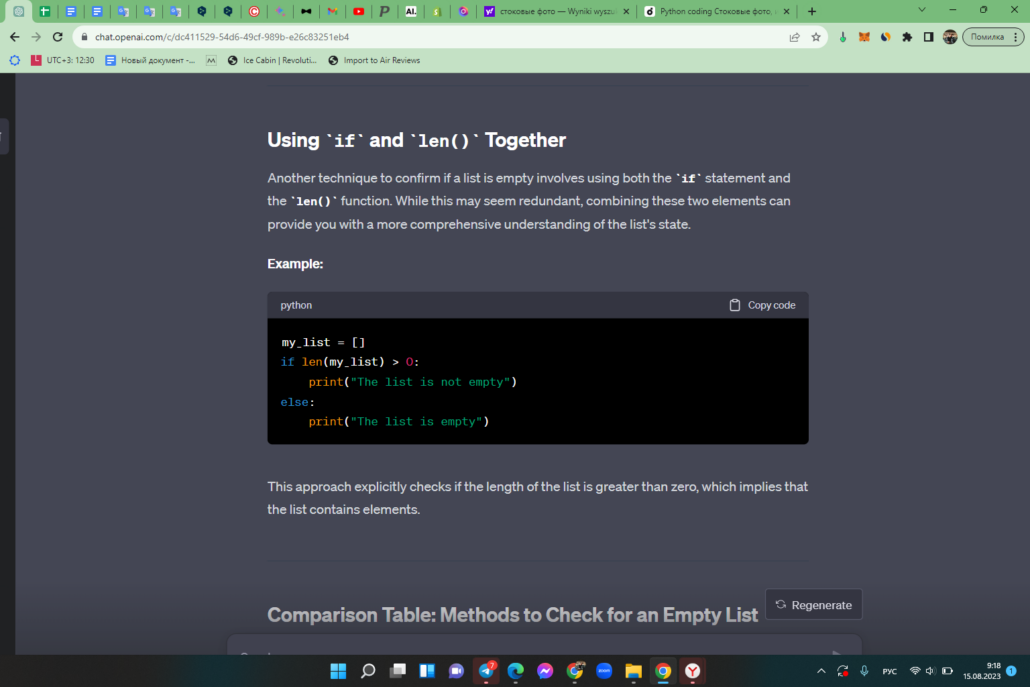
In this example, the any() function evaluates to False for an empty list, effectively detecting its emptiness.
Using Exception Handling
Exception handling is another intriguing way to identify an empty list. By attempting to access the first element of the list within a try block and catching the IndexError exception, you can deduce whether the list is empty or not.
Example:
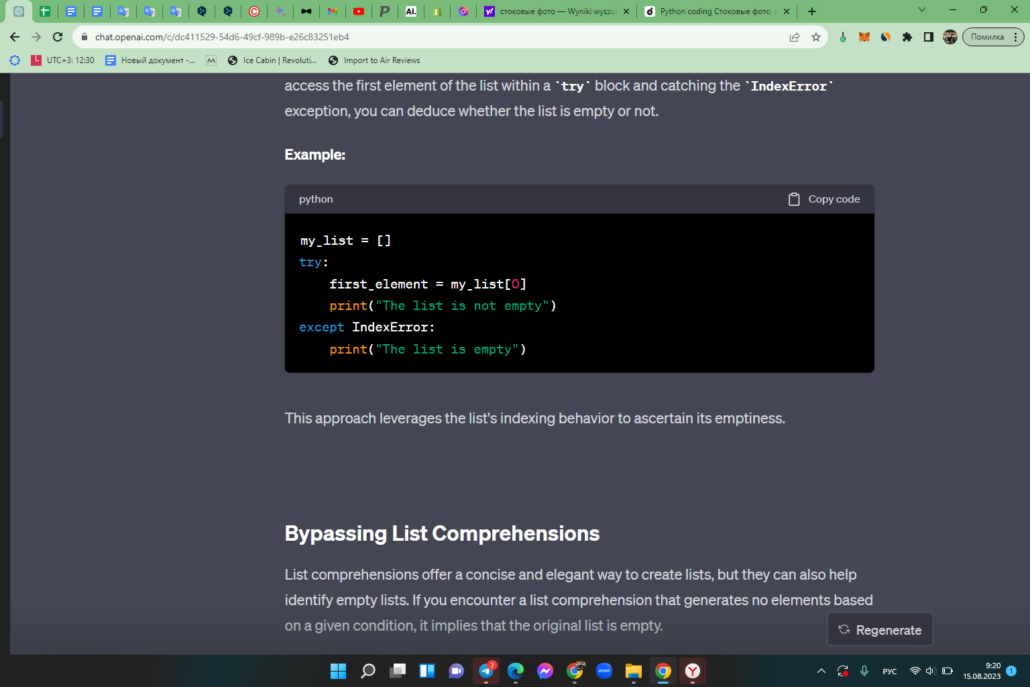
This approach leverages the list’s indexing behavior to ascertain its emptiness.
Bypassing List Comprehensions
List comprehensions offer a concise and elegant way to create lists, but they can also help identify empty lists. If you encounter a list comprehension that generates no elements based on a given condition, it implies that the original list is empty.
Example:
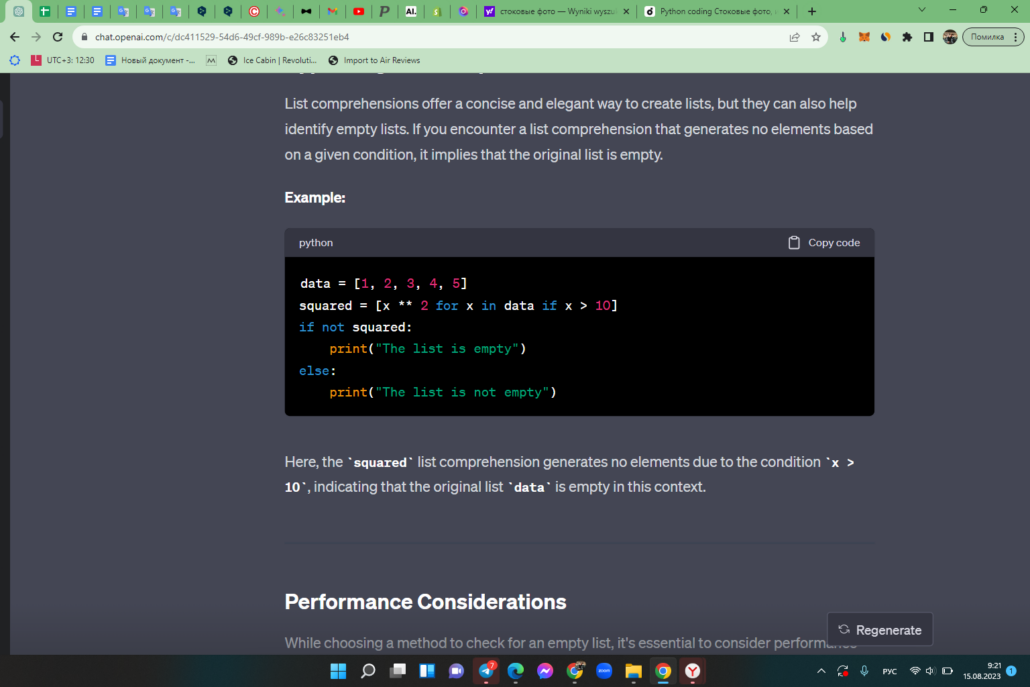
Here, the squared list comprehension generates no elements due to the condition x > 10, indicating that the original list data is empty in this context.
Methods to Check for an Empty List
To provide a quick overview, let’s compare the three methods discussed above in a comparison table:
Method | Description | Pros | Cons |
---|---|---|---|
len() Function | Uses the len() function to determine list length. | Simple and easy to understand. | Involves an explicit comparison. |
Conditional Statement | Utilizes the truthiness of lists in a boolean context. | Elegant and concise syntax. | May not be as intuitive for beginners. |
if and len() Combination | Combines if statement with the len() function. | Offers a clear insight into list state. | Slightly more verbose than other methods. |
Practical Use Cases
Understanding how to check for an empty list is crucial in various scenarios. Here are some practical situations where you might need to apply these techniques:
- Data Validation: Before processing user inputs, check if a list is empty to ensure that valid data is present;
- Iteration Control: When iterating through a list, verifying its emptiness prevents unnecessary iterations;
- Error Handling: Empty lists can trigger errors in some operations, so it’s essential to handle them appropriately.
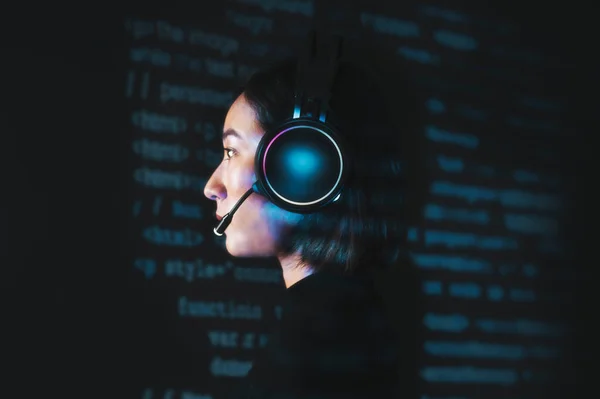
Conclusion
In Python programming, efficiently detecting an empty list is essential for writing robust and error-free code. By mastering the three methods outlined in this article, you’ll be well-equipped to handle various situations where checking for an empty list is necessary. Whether you prefer the simplicity of the len() function or the elegance of conditional statements, these techniques will undoubtedly enhance your coding skills.
FAQs
Yes, you can. The if statement, in combination with the truthiness of lists, provides a concise way to determine if a list is empty.
The len() function is straightforward and familiar to most programmers, making it a preferred choice for readability.
Yes, these methods are applicable to other data structures as well. You can use them to check the emptiness of tuples, dictionaries, and other iterable objects.
Generally, the performance differences are negligible for most use cases. Choose the method that aligns with your coding style and project requirements.
Absolutely! You can use logical operators (such as and or or) to create more complex conditions that involve checking for an empty list along with other criteria.