In the world of Python programming, effectively locating the index of a specific element within a List is an essential skill. This guide will walk you through various methods to achieve this, employing Python’s built-in functions and techniques.
Python List Index -1: Obtaining the Last Element’s Index
Finding the last element’s index in a Python List is a common task that can be conveniently achieved using index -1. This negates the need for manual index calculations when dealing with the final element.
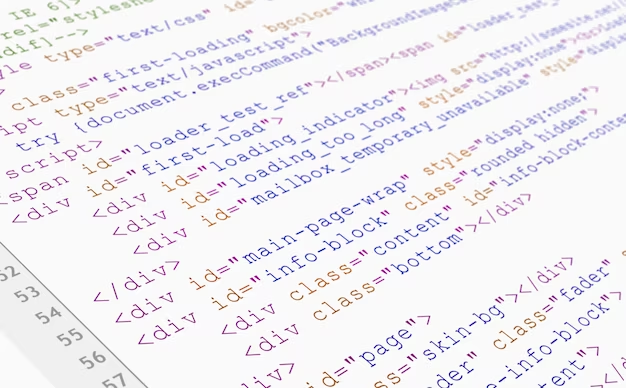
Using List Comprehension
Using list comprehension, you can elegantly obtain the indices of occurrences of a specific element in a Python List.
new_list = [62, 84, 29, 82, 29, 29, 11, 29] element_to_find = 29 indices = [i for i in range(len(new_list)) if new_list[i] == element_to_find] print(“Indices where”, element_to_find, “is present:”, indices)
Using the index() Function
Python provides the index() function, a built-in method that returns the index of the first occurrence of an element in a List.
fruits = [“apple”, “banana”, “cherry”] element_to_find = “banana” index = fruits.index(element_to_find) print(“Index of”, element_to_find, “is:”, index)
Utilizing enumerate() for Index Retrieval
The enumerate() function is a versatile tool for obtaining both index and value pairs, making it valuable for extracting indices based on specific criteria.
new_list = [98, 178, 178, 145, 913] element_to_find = 178 indices = [i for i, value in enumerate(new_list) if value == element_to_find] print(“Indices where”, element_to_find, “is present:”, indices)
Applying the filter() Method
The filter() method is handy for filtering a list based on a given condition. It is also useful for obtaining indices that meet specific criteria.
new_values = [78, 23, 17, 17, 89, 56, 17] element_to_find = 17 indices = list(filter(lambda i: new_values[i] == element_to_find, range(len(new_values)))) print(“Indices for”, element_to_find, “:”, indices)
Comparative Analysis of Methods
Each method has its own strengths and uses cases. Consider your project’s requirements to choose the most suitable approach:
Method | Pros | Cons |
---|---|---|
List Comprehension | Concise, obtains indices meeting specific criteria | Limited to specific criteria |
index() Function | Simple, returns index of first occurrence | Limited to first occurrence |
enumerate() | Provides index and value pairs, versatile | Extracts all occurrences of element |
filter() Method | Filters based on condition, obtains indices meeting criteria | Requires lambda function for filtering |
- List Comprehension: Obtain indices meeting specific criteria;
- index() Function: Find the index of the first occurrence;
- enumerate(): Extract indices alongside element values;
- filter() Method: Get indices satisfying a condition.
Conclusion
Obtaining the index of an element within a Python List is a foundational skill for any programmer. Armed with various methods, you can confidently navigate tasks involving list manipulation and searching. Tailor your choice of method to your project’s needs, enhancing both efficiency and readability in your Python programming.
Remember, versatility in handling different scenarios is key to becoming a proficient Python programmer.
FAQ
Index -1 is used to access the last element of a list without the need for manual calculations. It simplifies retrieving the last element’s index.
Yes, list comprehension can be employed to obtain indices of all occurrences of a specific element in a list.
You can avoid ValueError by checking if the element is present in the list using a conditional statement, or by using try-except blocks for exception handling.
Yes, you can modify the list comprehension or other methods to find the index of the second occurrence of an element by skipping the first occurrence.