Python web development has taken the digital world by storm, and at the heart of this process lies the mighty manage.py runserver command. If you’re a developer venturing into the world of Python web applications, you’re in for a treat. In this comprehensive guide, we’ll unravel the mysteries surrounding manage.py runserver and equip you with the knowledge you need to embark on your coding journey.
Getting Started with manage.py runserver
Python web development often revolves around the Django framework, and manage.py runserver is a command-line tool that comes bundled with Django. It’s your gateway to the local development server – the key to testing your web applications before deploying them to a live environment.
Setting Up Your Environment
Before diving into the command itself, ensure you have Django installed. You can create a virtual environment and install Django using:
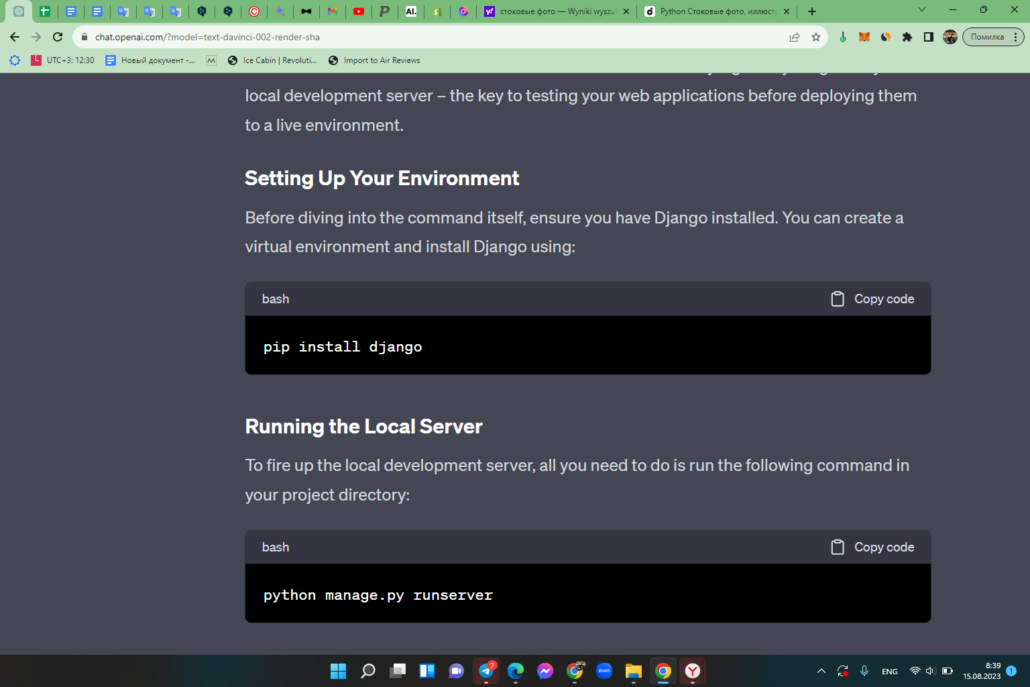
Running the Local Server
To fire up the local development server, all you need to do is run the following command in your project directory:
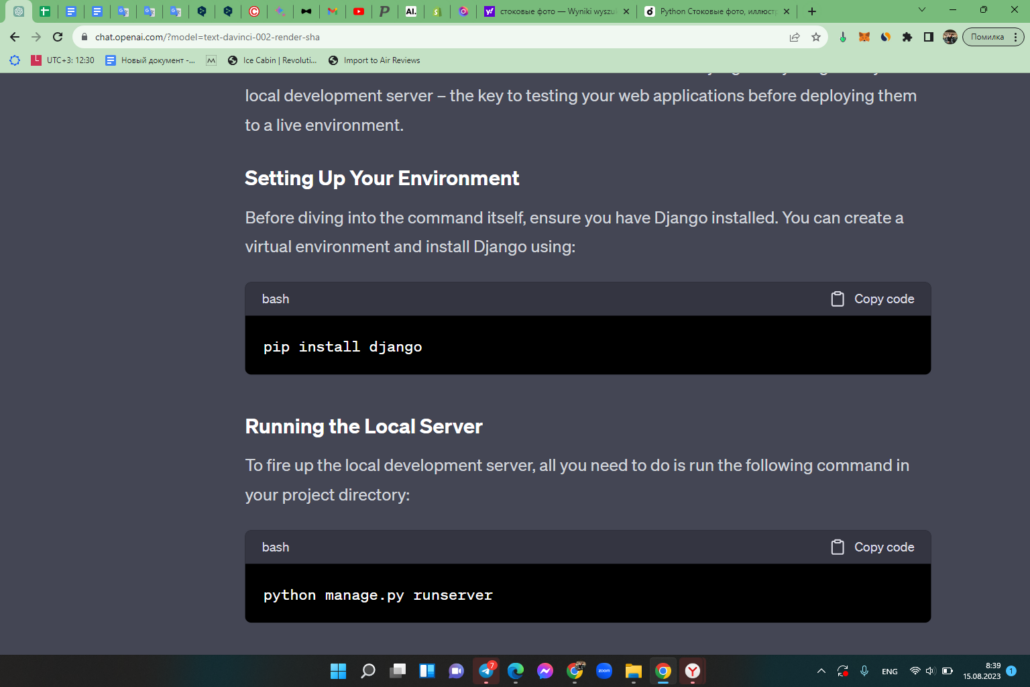
Voilà! Your development server is now up and running, and you can access your web app at http://127.0.0.1:8000/.
Understanding the Command
Let’s break down the components of the manage.py runserver command:
- manage.py: This script acts as a command-line tool for various Django management tasks;
- runserver: This is the specific command we’re using to start the local development server.
The beauty of runserver lies in its simplicity; it automates much of the server setup, allowing you to focus on coding.
Navigating manage.py runserver Features
Automatic Reload
One of the standout features of the development server is its automatic reload functionality. This means that whenever you make changes to your code, the server will automatically restart, reflecting those changes instantly. No more manual restarts!
Specifying Ports
By default, the development server runs on port 8000. But what if you want to use a different port? Simply append the desired port number to the command:
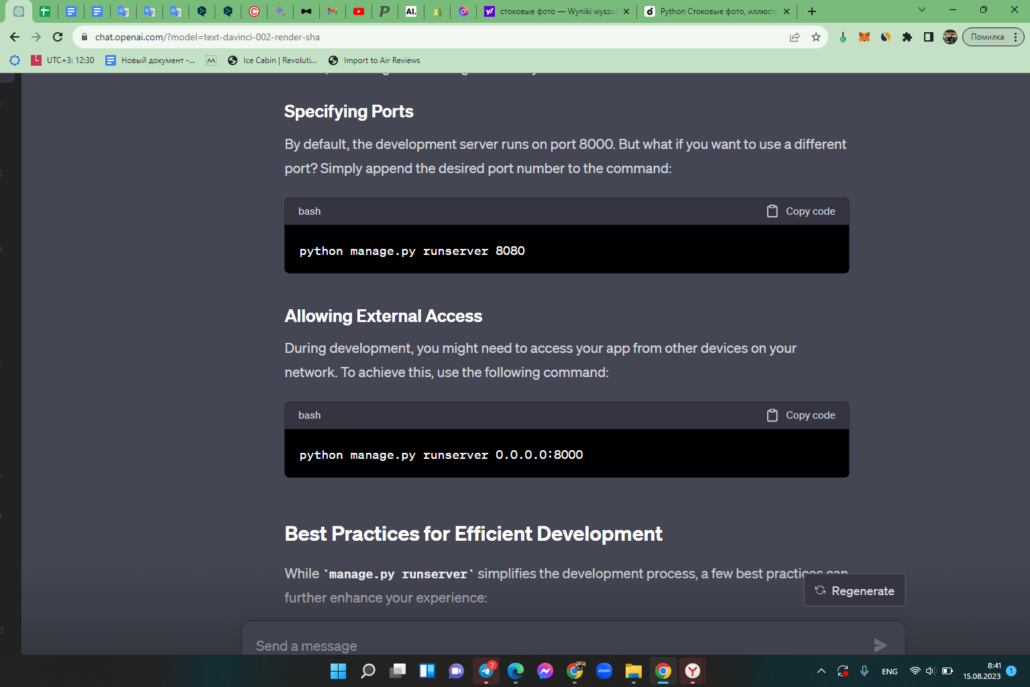
Allowing External Access
During development, you might need to access your app from other devices on your network. To achieve this, use the following command:
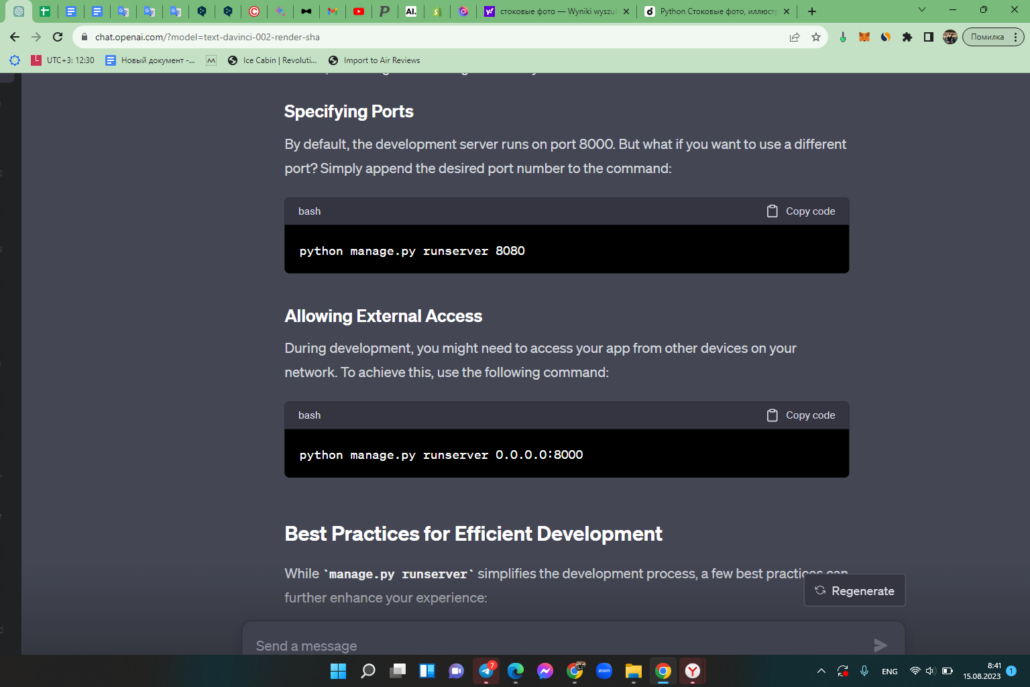
Best Practices for Efficient Development
While manage.py runserver simplifies the development process, a few best practices can further enhance your experience:
- Keep Your Code Modular: Divide your code into reusable modules for easy maintenance;
- Utilize Django’s Features: Leverage Django’s built-in functionalities for faster development;
- Implement Version Control: Use Git or other version control systems to track changes effectively.
Troubleshooting and Tips
Developers often encounter a few hiccups while using manage.py runserver. Here are some common issues and how to overcome them:
Problem | Solution |
---|---|
Port Already in Use | Change the port number: python manage.py runserver 8080 |
Database Migration Errors | Run python manage.py makemigrations followed by python manage.py migrate to update changes. |
Comparison: runserver vs Production Servers
Aspect | runserver | Production Server |
---|---|---|
Use Case | Development and testing | Hosting live applications |
Performance | Single-threaded and not optimized for speed | Optimized for high traffic and speed |
Security | Minimal security measures | Robust security features |
Static Files | Slower handling of static files | Efficient handling of static files |
Enhancing Development Workflow with runserver Extensions
Django’s extensibility allows developers to enhance the capabilities of the runserver command through various extensions. These extensions provide additional features and functionalities that can streamline your development workflow.
Django Extensions
The django-extensions package offers a collection of custom management commands, including ones that complement runserver. For example, you can use the runserver_plus command, which integrates the Werkzeug development server, providing advanced debugging and code reloading capabilities.
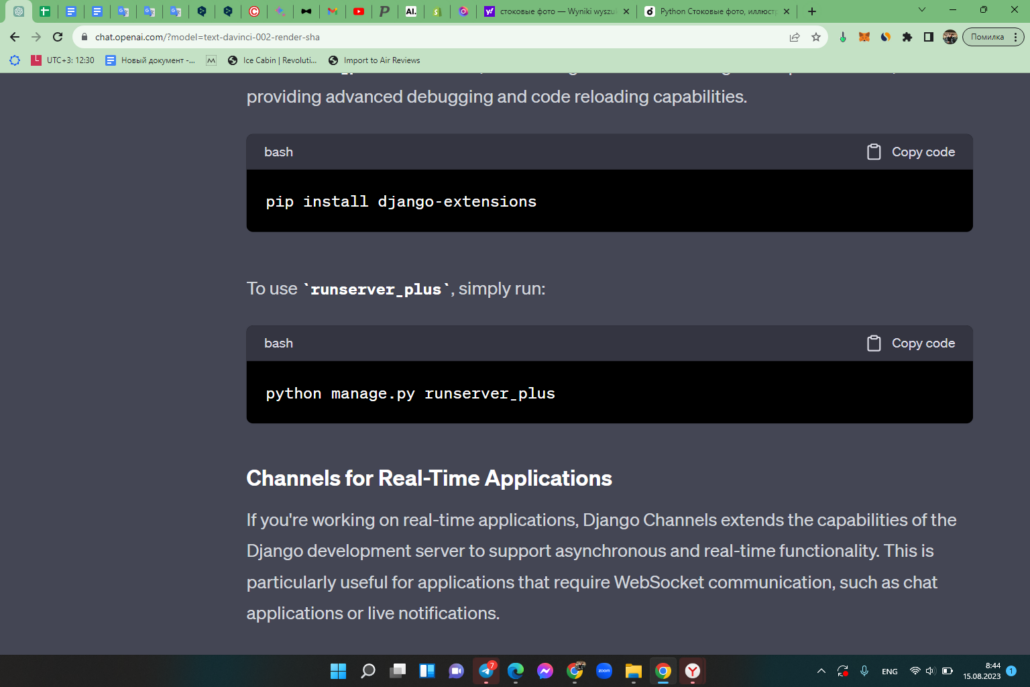
To use runserver_plus, simply run:
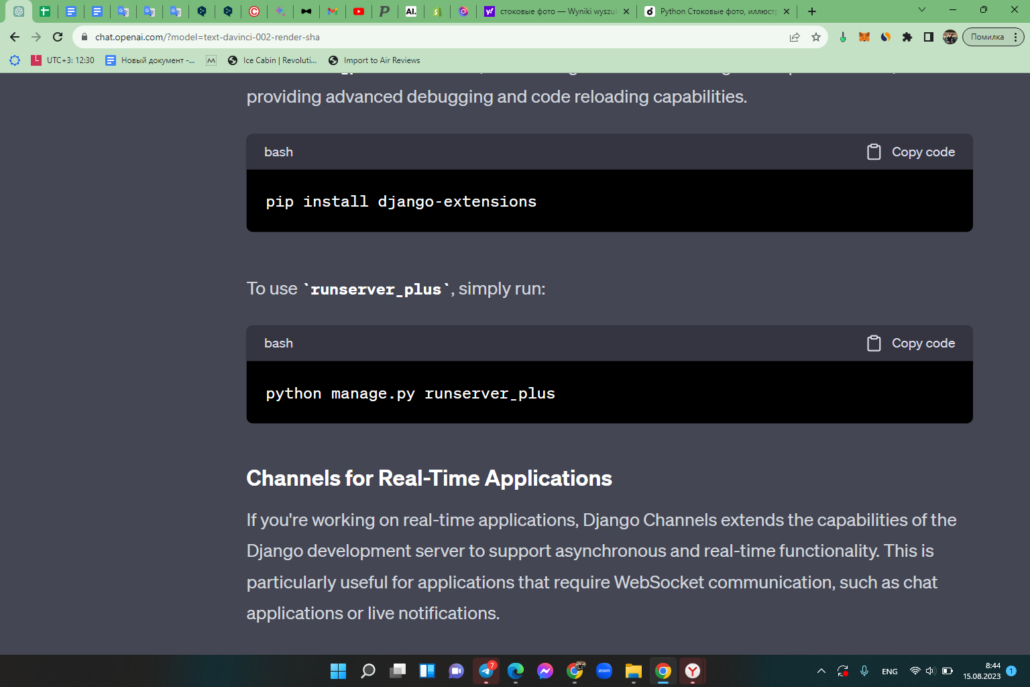
Channels for Real-Time Applications
If you’re working on real-time applications, Django Channels extends the capabilities of the Django development server to support asynchronous and real-time functionality. This is particularly useful for applications that require WebSocket communication, such as chat applications or live notifications.
Advanced Configuration and Customization
While the default behavior of manage.py runserver is sufficient for most projects, you can further customize its behavior by passing various optional arguments.
Changing the Host and Port
You can specify a different host and port using the –host and –port options:
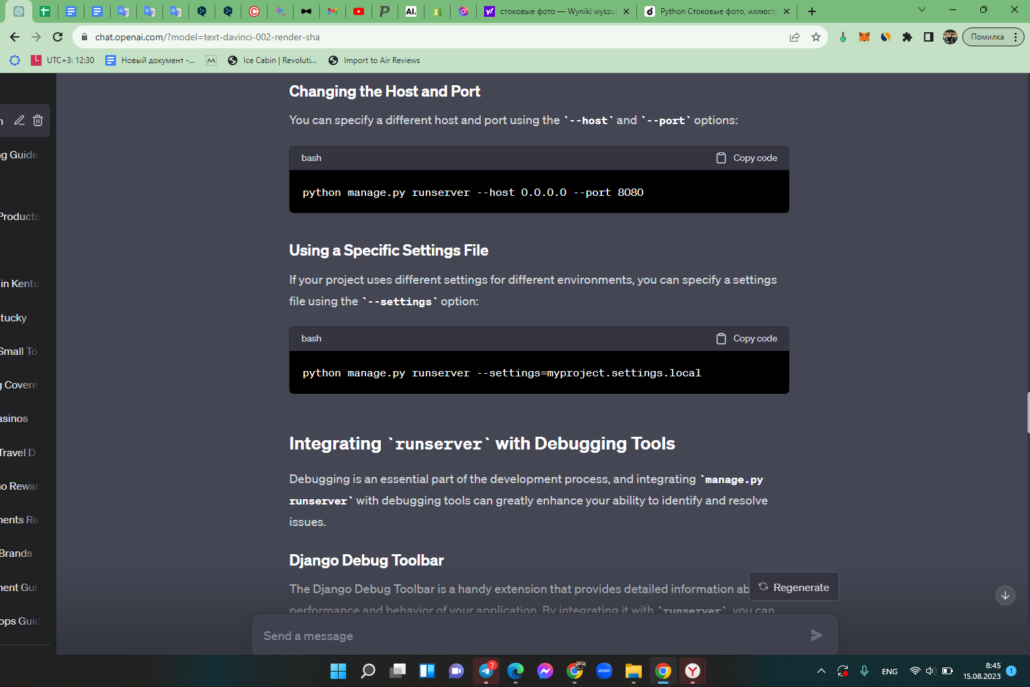
Using a Specific Settings File
If your project uses different settings for different environments, you can specify a settings file using the –settings option:
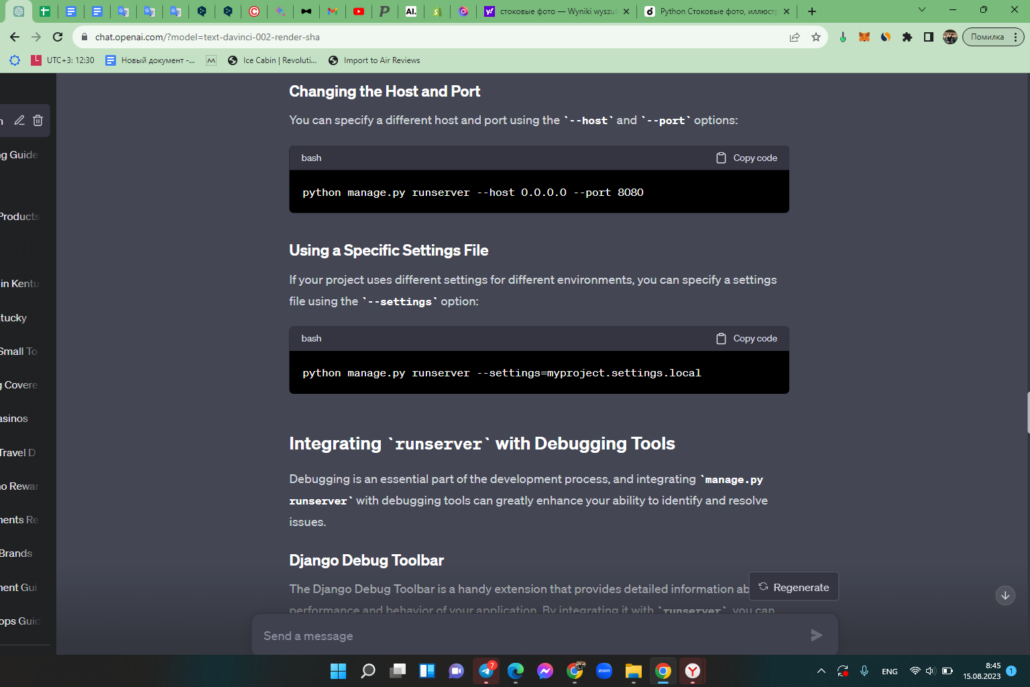
Integrating runserver with Debugging Tools
Debugging is an essential part of the development process, and integrating manage.py runserver with debugging tools can greatly enhance your ability to identify and resolve issues.
Django Debug Toolbar
The Django Debug Toolbar is a handy extension that provides detailed information about the performance and behavior of your application. By integrating it with runserver, you can monitor database queries, template rendering times, and more.
To install and use the Debug Toolbar, follow these steps:
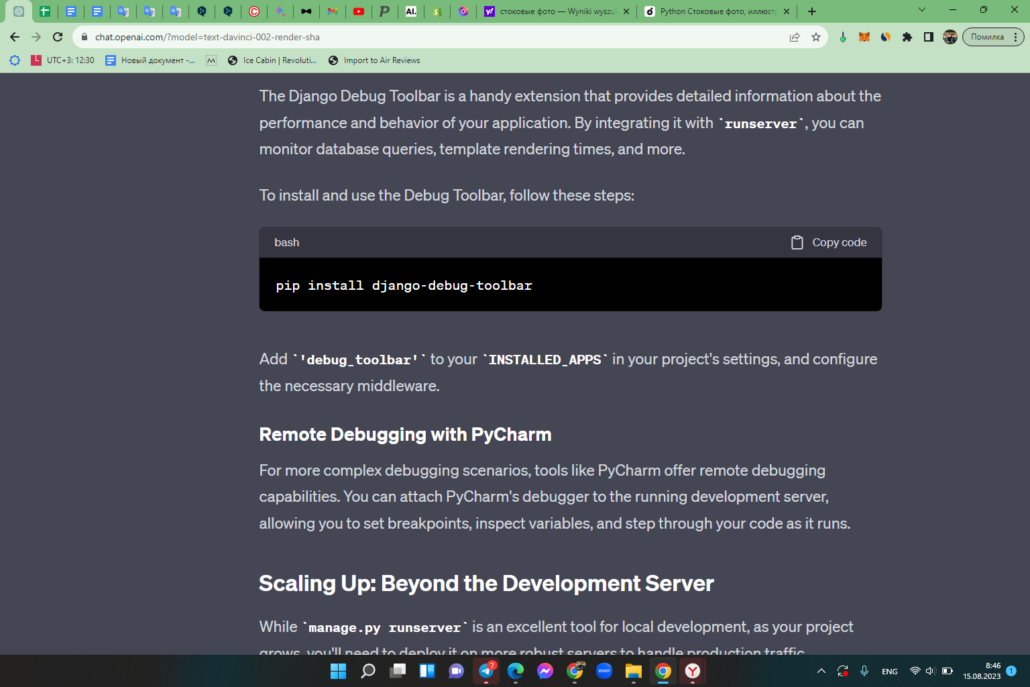
Add ‘debug_toolbar’ to your INSTALLED_APPS in your project’s settings, and configure the necessary middleware.
Remote Debugging with PyCharm
For more complex debugging scenarios, tools like PyCharm offer remote debugging capabilities. You can attach PyCharm’s debugger to the running development server, allowing you to set breakpoints, inspect variables, and step through your code as it runs.
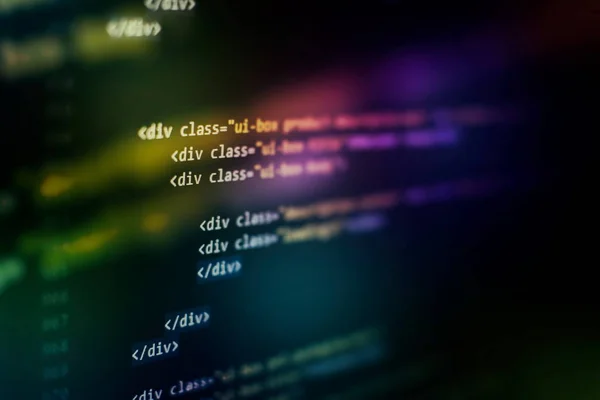
Scaling Up: Beyond the Development Server
While manage.py runserver is an excellent tool for local development, as your project grows, you’ll need to deploy it on more robust servers to handle production traffic.
Deploying to Production
For production deployments, consider using web servers like Nginx or Apache in conjunction with application servers like Gunicorn or uWSGI. These setups provide the performance, security, and scalability required for serving applications to a larger audience.
Containerization with Docker
Containerization has gained immense popularity for its ability to package applications along with their dependencies. Docker, along with tools like Docker Compose, allows you to create consistent and portable environments for your application to run smoothly across different environments.
Optimizing Performance for Development
Efficiency is paramount in the development process. While manage.py runserver offers convenience, there are ways to optimize its performance for a smoother coding experience.
Enabling Threading for Faster Loading
By default, Django’s development server doesn’t handle multiple requests concurrently. To enable threading for faster loading, use the –noreload option:
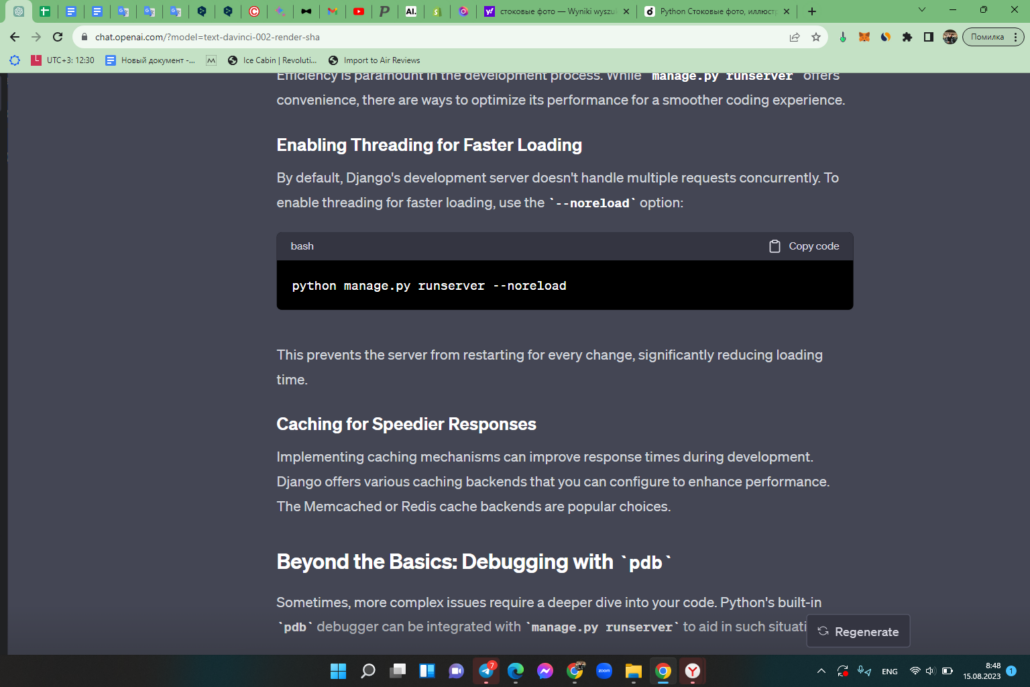
This prevents the server from restarting for every change, significantly reducing loading time.
Caching for Speedier Responses
Implementing caching mechanisms can improve response times during development. Django offers various caching backends that you can configure to enhance performance. The Memcached or Redis cache backends are popular choices.
Beyond the Basics: Debugging with pdb
Sometimes, more complex issues require a deeper dive into your code. Python’s built-in pdb debugger can be integrated with manage.py runserver to aid in such situations.
Setting Breakpoints
Inserting breakpoints using the pdb debugger allows you to pause the execution of your code at a specific line. To set a breakpoint, add the following code at the desired location:
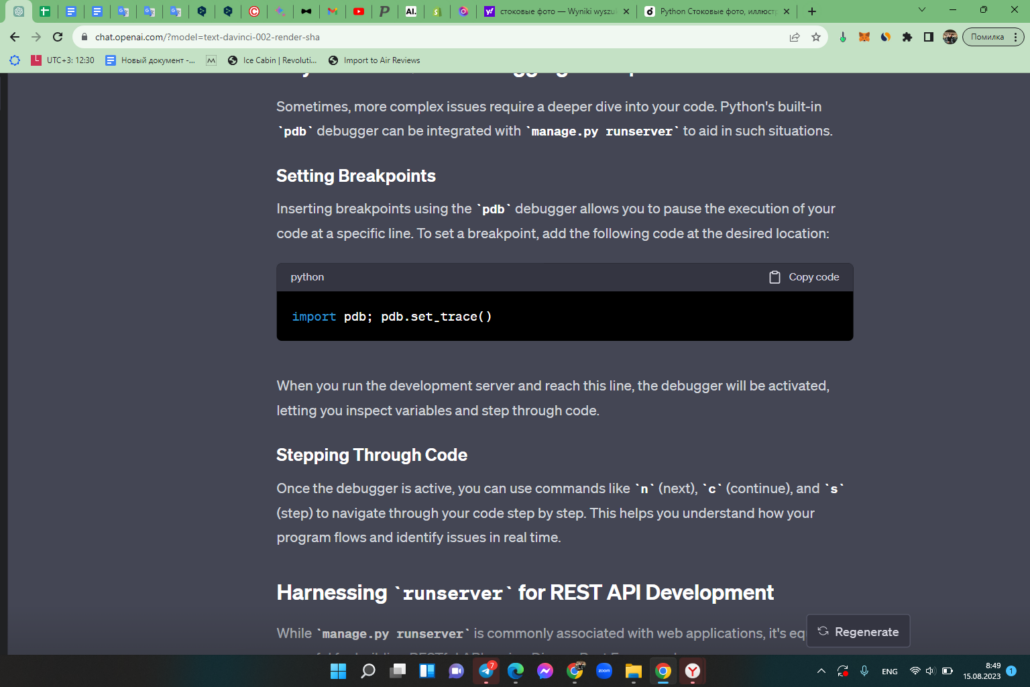
When you run the development server and reach this line, the debugger will be activated, letting you inspect variables and step through code.
Stepping Through Code
Once the debugger is active, you can use commands like n (next), c (continue), and s (step) to navigate through your code step by step. This helps you understand how your program flows and identify issues in real time.
Harnessing runserver for REST API Development
While manage.py runserver is commonly associated with web applications, it’s equally powerful for building RESTful APIs using Django Rest Framework.
Building APIs with Django Rest Framework
Django Rest Framework (DRF) is a popular package for creating APIs in Django projects. With runserver, you can instantly preview your API’s behavior during development.
Install DRF using:
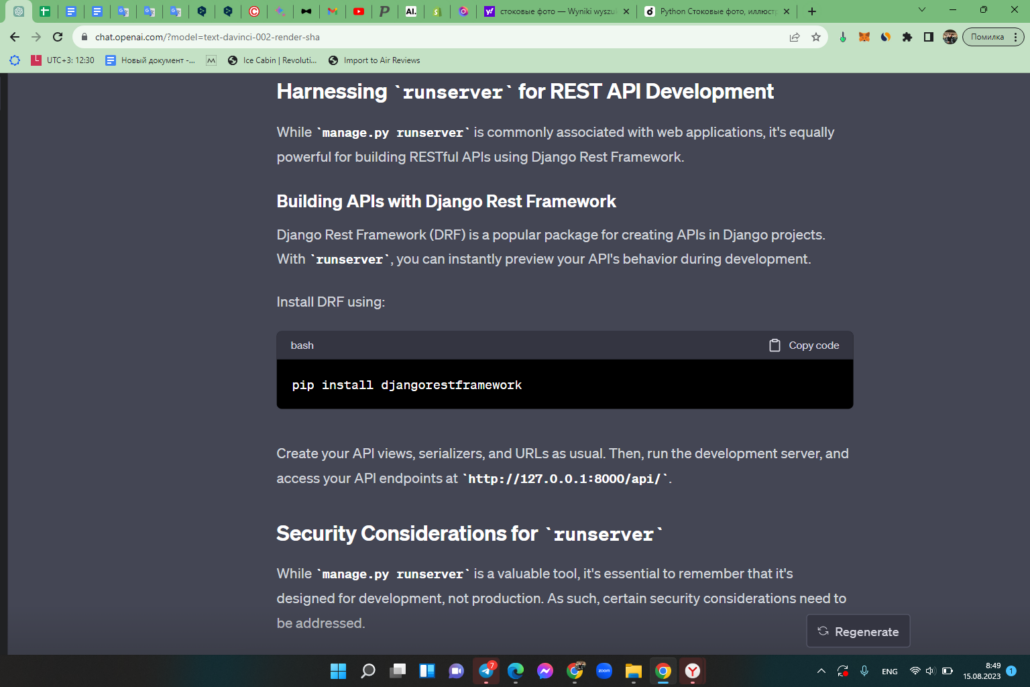
Create your API views, serializers, and URLs as usual. Then, run the development server, and access your API endpoints at http://127.0.0.1:8000/api/.
Security Considerations for runserver
While manage.py runserver is a valuable tool, it’s essential to remember that it’s designed for development, not production. As such, certain security considerations need to be addressed.
Disabling Debug Mode
By default, runserver runs in debug mode, which provides detailed error messages. In a production environment, you should always disable debug mode to prevent exposing sensitive information to potential attackers.
To run runserver without debug mode:
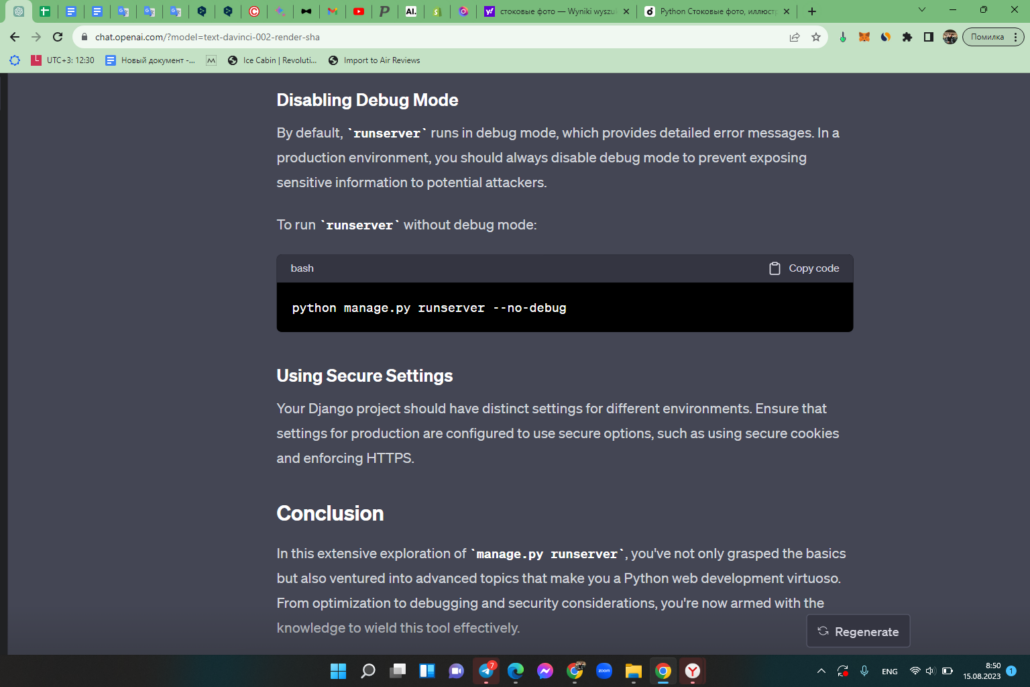
Using Secure Settings
Your Django project should have distinct settings for different environments. Ensure that settings for production are configured to use secure options, such as using secure cookies and enforcing HTTPS.
Conclusion
Python’s manage.py runserver is a developer’s best friend when it comes to building and testing web applications. Its simplicity, automatic reloading, and flexibility make it an essential tool in your development arsenal.
Remember, mastering manage.py runserver is just the beginning of your Python web development journey. Embrace the learning process, explore more Django functionalities, and keep building amazing web experiences!
FAQs
manage.py is a command-line utility that helps you perform various administrative tasks in a Django project, such as starting the development server, creating database tables, and more.
While runserver is perfect for development and testing, it’s not recommended for production due to its limited capabilities and security features.
In a production environment, consider using a web server like Nginx or Apache to serve static files efficiently, unlike the runserver which is slower in handling them.
Yes, creating a virtual environment for each project is a best practice. It helps isolate project-specific dependencies and prevents conflicts between packages.
To contribute to Django, you can check out their official documentation on how to get involved with the development process.