Strings are fundamental in Python programming, but dealing with unwanted whitespace can be a hassle. Fear not, for we’re about to dive into the world of string trimming using Python’s powerful functions: strip(), lstrip(), and rstrip(). Whether you’re a beginner or an experienced coder, this comprehensive guide will help you wield these tools with confidence.
Understanding String Trimming
Whitespace, those pesky spaces before, after, or within a string, can disrupt your code’s flow and lead to unexpected bugs. Let’s explore the techniques to remove these spaces.
The Basics of strip():
The strip() method is a versatile tool that eliminates leading and trailing whitespace from a string. It’s like trimming the edges of a photograph to focus on the subject. Here’s how you use it:
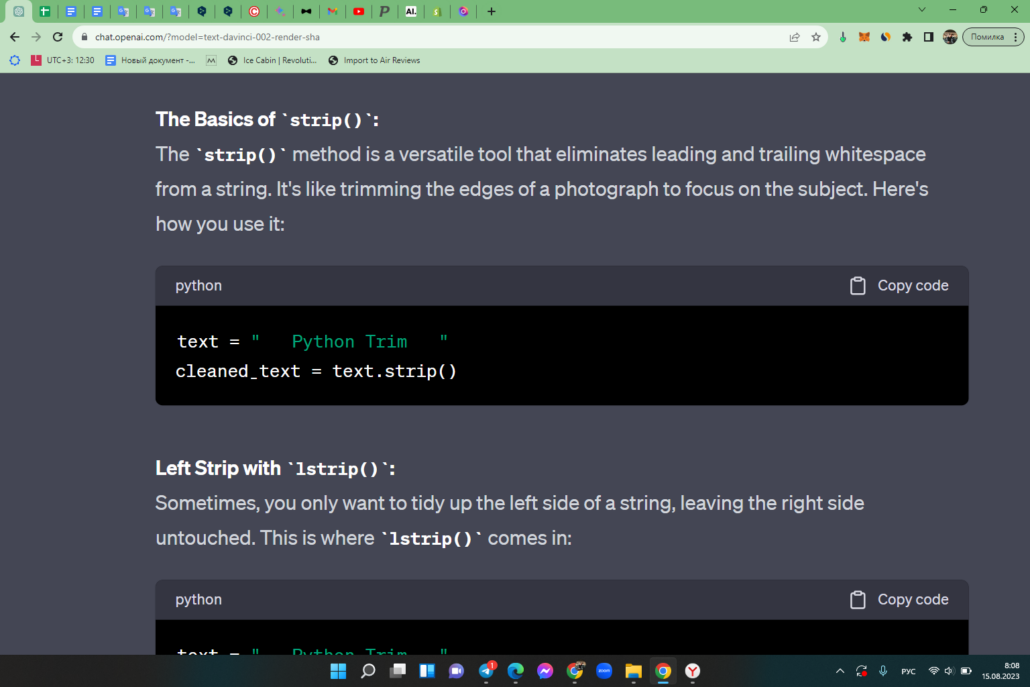
Left Strip with lstrip():
Sometimes, you only want to tidy up the left side of a string, leaving the right side untouched. This is where lstrip() comes in:
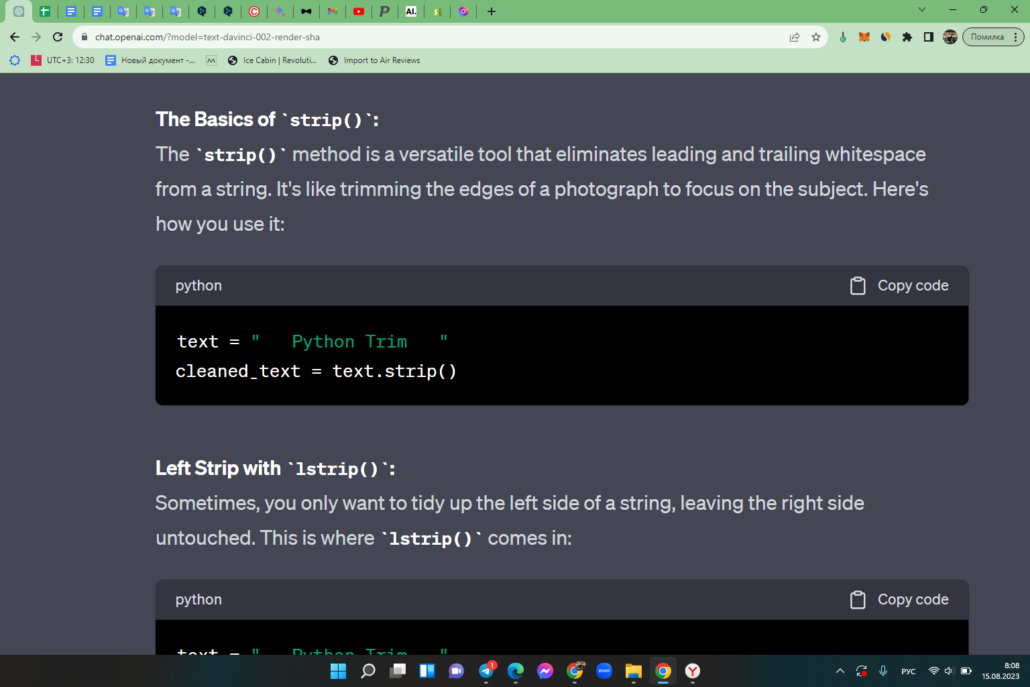
Right Strip with rstrip():
Conversely, rstrip() is perfect for removing spaces from the right side of a string:
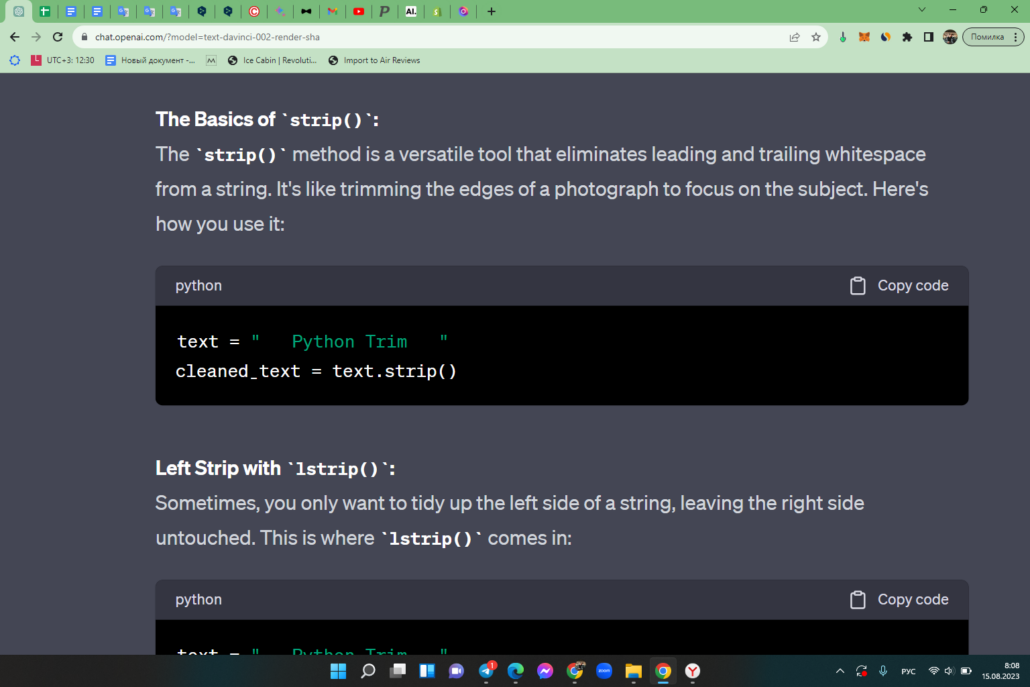
Mastering Whitespace Removal Techniques
Each of these functions has its place in different scenarios. Let’s explore when and where to apply them.
Comparing strip() vs. lstrip() vs. rstrip() (Chart):Use Cases and Examples
Function | Removes Leading Whitespace | Removes Trailing Whitespace | Removes Both |
---|---|---|---|
strip() | ✓ | ✓ | |
lstrip() | ✓ | ||
rstrip() | ✓ |
Use Cases and Examples
Let’s see these functions in action with real-world examples.
Scenario 1: User Input Cleaning:
When handling user inputs, trimming whitespace ensures that unintended spaces don’t affect your logic:
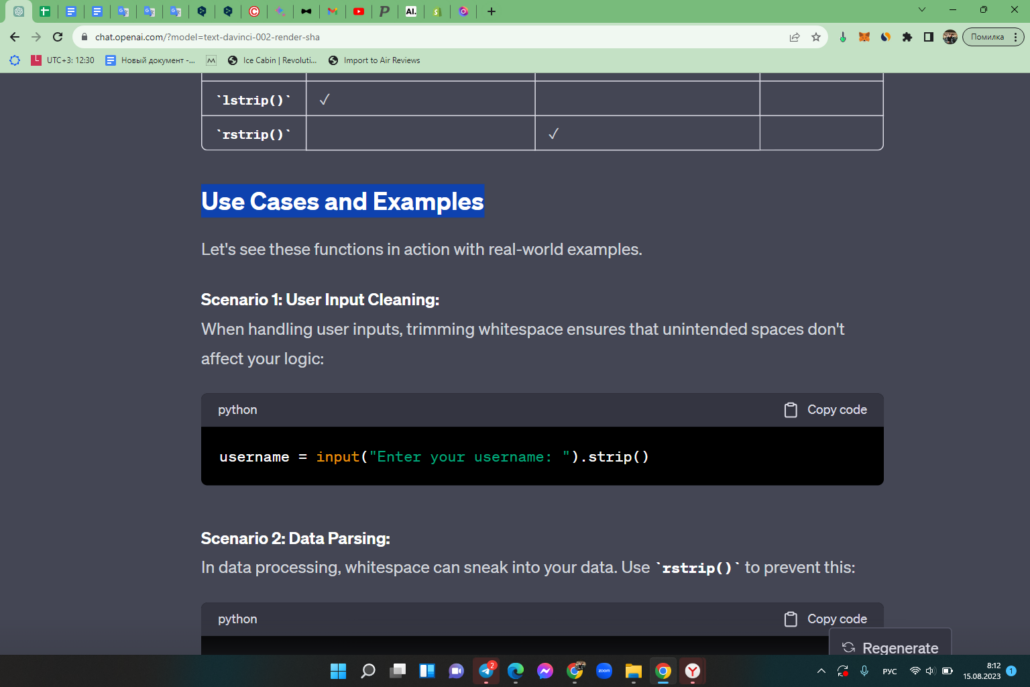
Scenario 2: Data Parsing:
In data processing, whitespace can sneak into your data. Use rstrip() to prevent this:
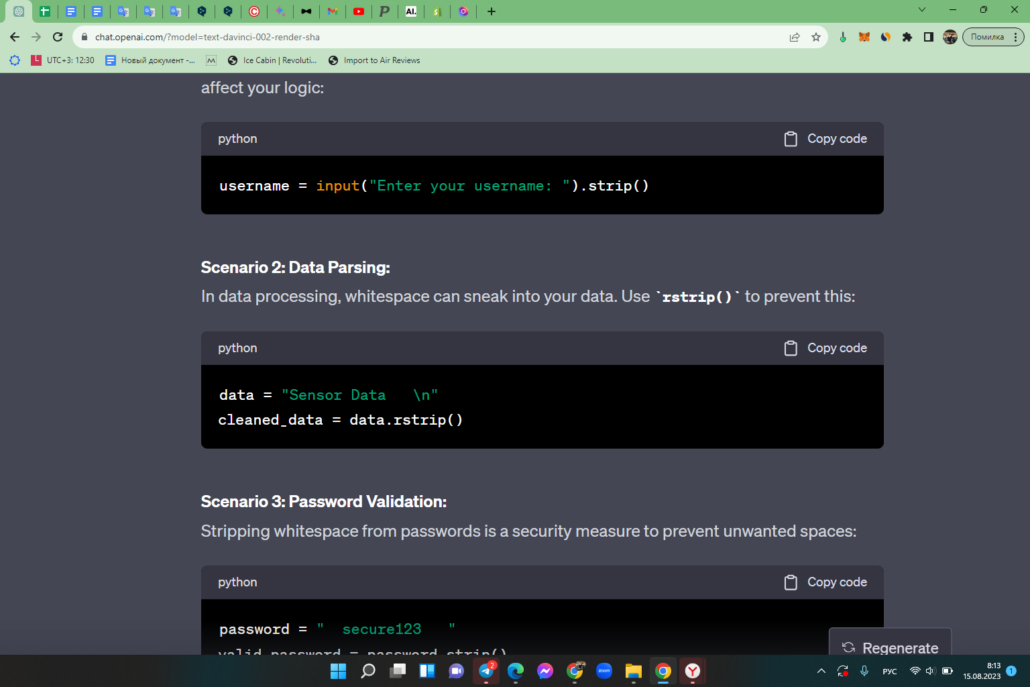
Scenario 3: Password Validation:
Stripping whitespace from passwords is a security measure to prevent unwanted spaces:
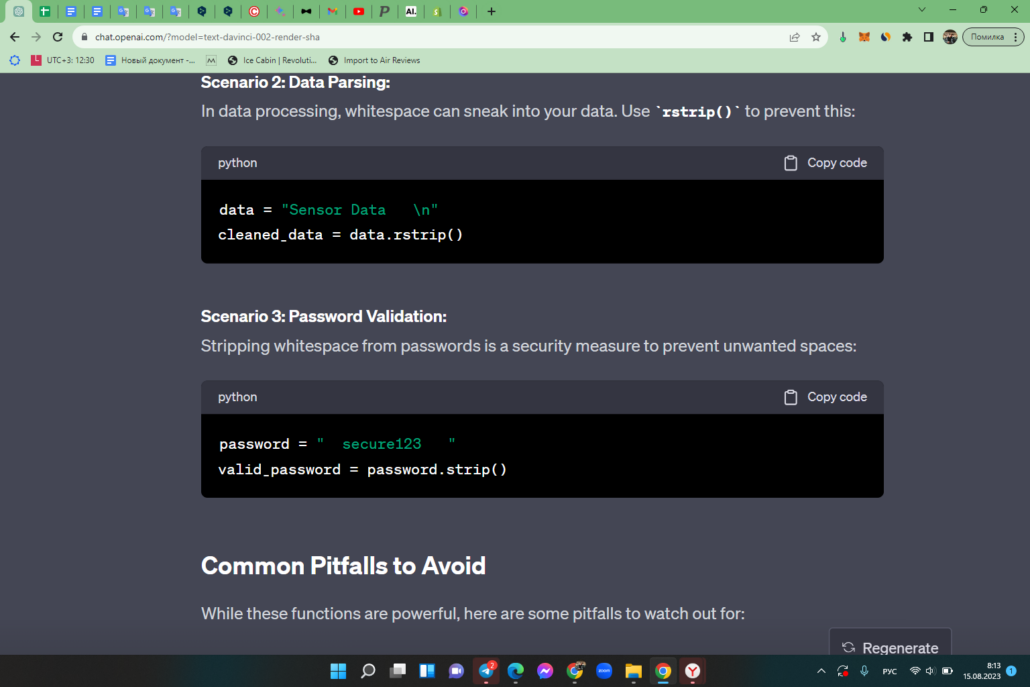
Common Pitfalls to Avoid
While these functions are powerful, here are some pitfalls to watch out for:
1. Over-Trimming:
Be cautious not to strip away characters you actually need, like spaces within the string;
2. Not Assigning Results:
Remember to assign the result of the trimming back to the variable for further use;
3. Using Wrong Functions:
Choose the appropriate function based on the type of whitespace you want to remove.
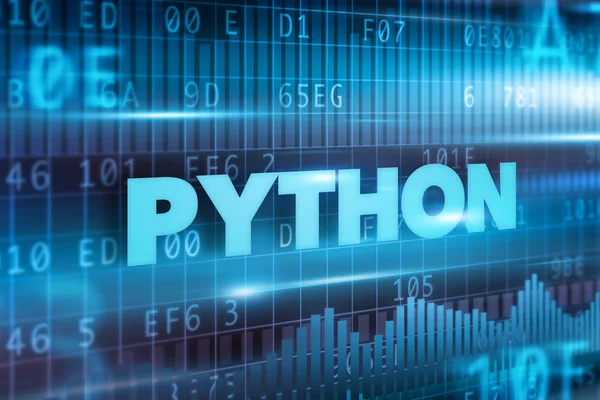
Advanced Techniques for String Trimming
When dealing with more complex scenarios, Python offers additional techniques to enhance your string trimming capabilities.
Custom Character Removal with strip():
The strip() function can go beyond removing whitespace. By providing a string argument, you can specify which characters to remove:
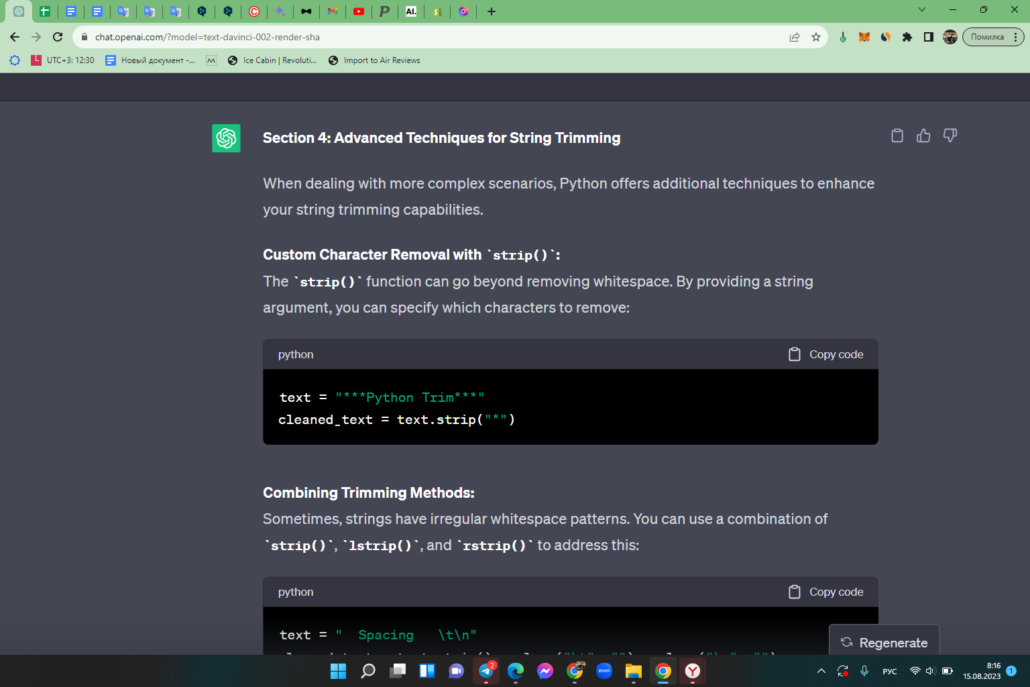
Combining Trimming Methods:
Sometimes, strings have irregular whitespace patterns. You can use a combination of strip(), lstrip(), and rstrip() to address this:
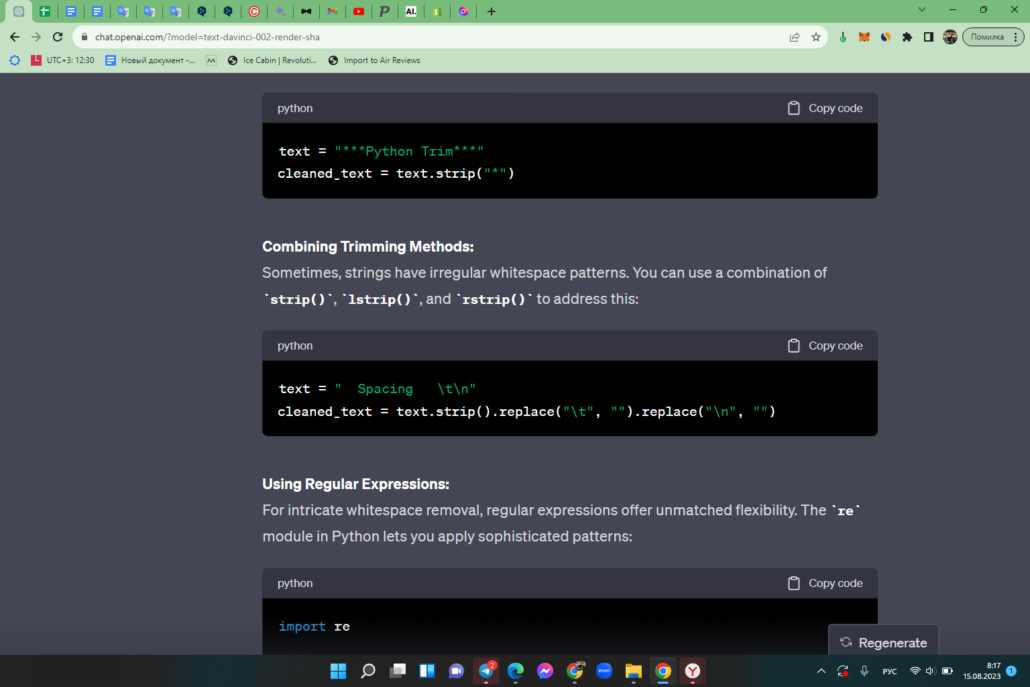
Using Regular Expressions:
For intricate whitespace removal, regular expressions offer unmatched flexibility. The re module in Python lets you apply sophisticated patterns:
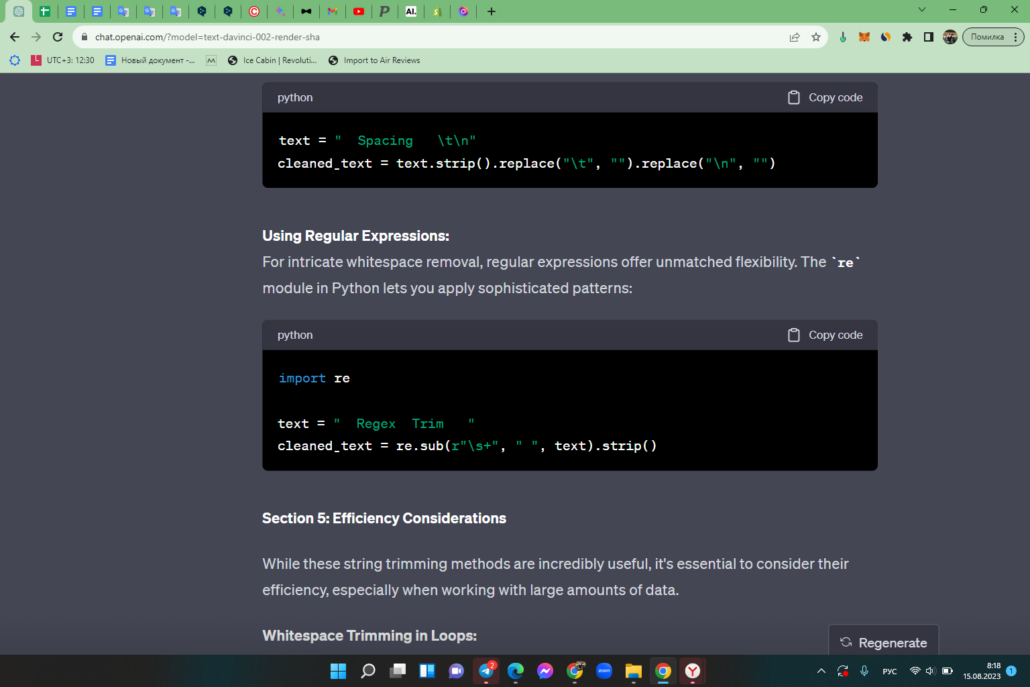
Efficiency Considerations
While these string trimming methods are incredibly useful, it’s essential to consider their efficiency, especially when working with large amounts of data.
Whitespace Trimming in Loops:
If you’re processing strings in loops, be mindful of calling trimming functions excessively, as this can impact performance. Trim the strings before the loop if possible.
Using str.join() for Lists:
When working with lists of strings, consider using str.join() along with a list comprehension for efficient whitespace removal:
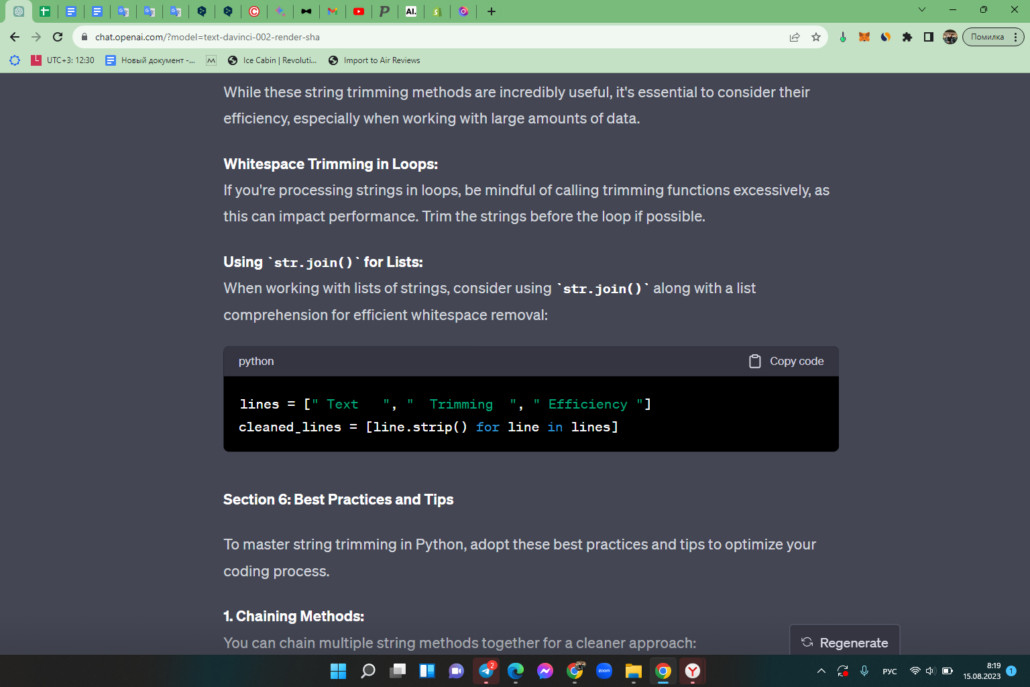
Best Practices and Tips
To master string trimming in Python, adopt these best practices and tips to optimize your coding process.
1. Chaining Methods:
You can chain multiple string methods together for a cleaner approach:
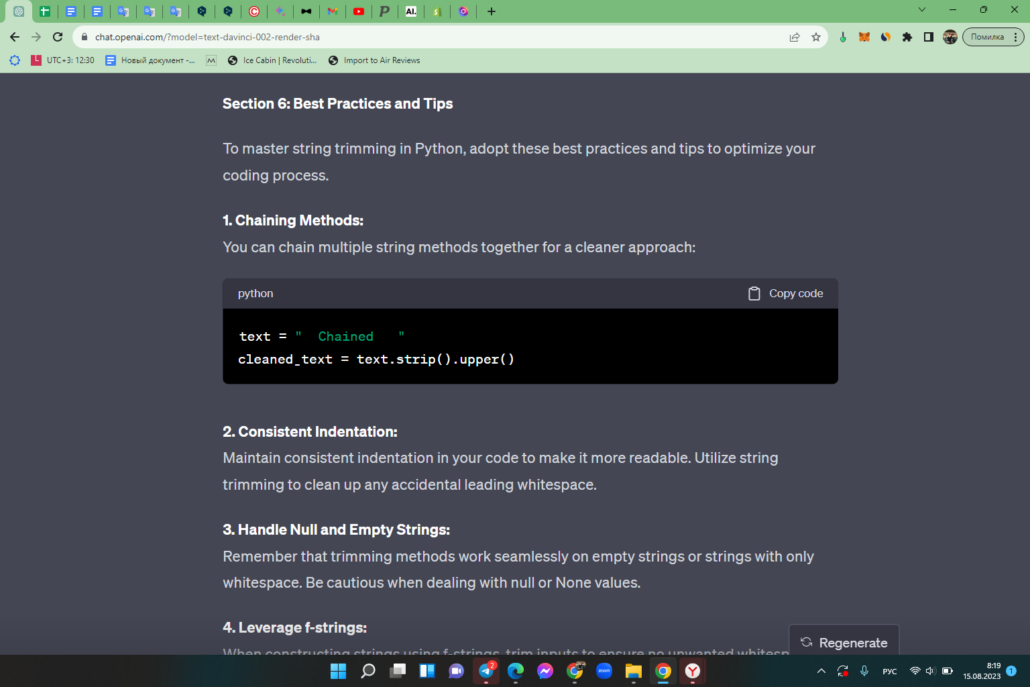
2. Consistent Indentation:
Maintain consistent indentation in your code to make it more readable. Utilize string trimming to clean up any accidental leading whitespace.
3. Handle Null and Empty Strings:
Remember that trimming methods work seamlessly on empty strings or strings with only whitespace. Be cautious when dealing with null or None values.
4. Leverage f-strings:
When constructing strings using f-strings, trim inputs to ensure no unwanted whitespace is included:
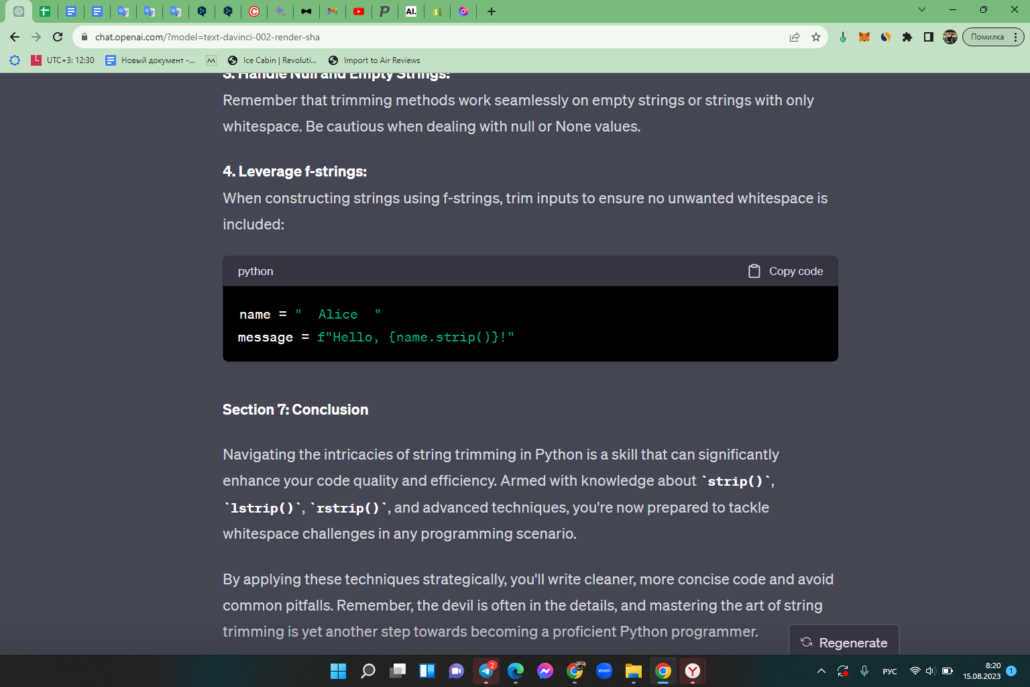
Conclusion
Python’s string trimming functions—strip(), lstrip(), and rstrip()—are invaluable tools to keep your code clean and precise. By now, you’re equipped to elegantly eliminate whitespace from your strings, enhancing the readability and functionality of your programs. Keep coding efficiently!
FAQs:
Absolutely! strip() works on individual strings within a list, helping you clean up lists efficiently.
Indeed, strip() focuses on leading and trailing spaces, while replace() replaces all occurrences of a specific substring.
Certainly! strip() can remove any characters specified in its argument, not just whitespace.
No, strip() creates a new string with the whitespace removed, leaving the original string unchanged.
When used together, strip() will remove both leading and trailing whitespace, while rstrip() only targets trailing whitespace.