Within this discourse, we shall delve into diverse approaches to ascertain the residual outcome stemming from a division endeavor in the Python programming realm. Among the multitude of techniques to unveil a remainder value within Python, the eminent choice resides in the utilization of the modulo operator, denoted as ‘%’. This symbol stands as the endorsed residual operator in Python’s syntax, serving to yield the lingering value following the division of one numerical entity by another. To illustrate, consider the instance where the computation 10 % 3 is undertaken, culminating in the output of 1, as the integer 3 can be accommodated thrice within 10, thereby leaving behind a remainder of 1.
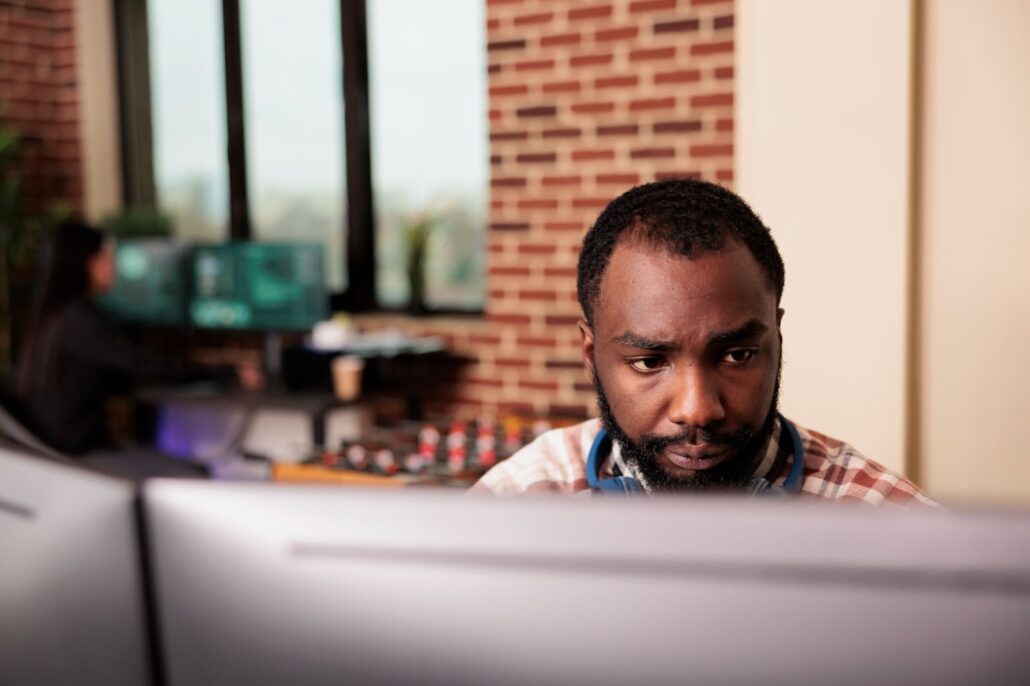
Method 1: Unveiling the Magic of the % Operator for Finding Remainders
Imagine a mathematical magician performing a trick that reveals the hidden world of remainders. This magician is none other than the ‘%’ operator, often called the modulo operator or remainder operator. Just like a skilled illusionist, this operator has the power to conjure up the remainder of a division operation, adding an element of intrigue and mystery to the realm of programming.
Unraveling the Concept: The Modulo Operator
In the realm of programming, the ‘%’ operator serves as a versatile tool, unlocking the enigma of remainders in division. While the traditional division operator (‘/’) effortlessly delivers the quotient of a division, the ‘%’ operator steps onto the stage to showcase the remainder. This operator dances with numbers, revealing the hidden gems that lie beyond the world of wholes.
Showcasing the % Operator in Action
Let’s immerse ourselves in the fascinating world of the ‘%’ operator with some captivating examples in Python. These snippets of code demonstrate the operator’s prowess in unveiling remainders:
>>> 7 % 3
1
>>> 9 % 5
4
>>> 12 % 2
0
Breaking Down the Elements: Dividend and Divisor
The essence of the ‘%’ operator revolves around two key players: the dividend and the divisor. The stage is set with the dividend, which represents the number under scrutiny, waiting to be divided. The divisor, the second protagonist, steps in to guide the division process.
Curtain Call: The Result
As the curtains draw back, the ‘%’ operator takes center stage. With elegance and precision, it returns the remainder of the division operation as its grand finale. In each example, the operator flawlessly calculates the remainder, painting a vivid picture of the numerical landscape.
In the first example, where the divisor is 3 and the dividend is 7, the division’s remainder is 1, and the ‘%’ operator masterfully showcases this result.
Practical Applications and Insights
The ‘%’ operator isn’t just a mere mathematical tool; it’s a guiding light in various programming scenarios. Here’s how you can harness its power:
- Fizz-Buzz Challenge: When solving the classic Fizz-Buzz problem, the ‘%’ operator can be your trusty ally. It helps identify numbers divisible by certain factors, enabling you to generate the desired output.
- Cycle Detection: In data structures like linked lists, the ‘%’ operator assists in detecting cycles. By calculating remainders, you can spot patterns and loops, enhancing the efficiency of your algorithms.
Method 2: Unveiling Division Insights with Python’s divmod() Function
In the realm of Python’s versatile toolkit, the divmod() function emerges as a powerful ally for unraveling the enigma of division. A true maestro of numbers, this built-in function elegantly accepts two numerical protagonists: the dividend and the divisor, and orchestrates a symphony that produces a tuple starring none other than the quotient and the remainder—both cast in harmonious unity.
Imagine wielding the divmod() function like a mathematical wand, conjuring the following captivating scenarios:
The Balanced Splitter:
divmod(8, 2) # Output: (4, 0)
In this act, the number 8 takes center stage as the dividend, and 2 plays the role of the divisor. As the divmod() spell unfolds, it conjures a tuple where the quotient is 4, and the remainder gracefully bows out at 0.
The Quirky Division Dance:
divmod(9, 4) # Output: (2, 1)
Behold, a whimsical dance of numbers unfolds—9 enters the stage as the dividend, while 4 embarks on the role of the divisor. The divmod() enchantment weaves a tale where the quotient takes a bow as 2, while the remainder pirouettes gently, landing as 1.
The Resilient Quotient and its Restive Companion:
divmod(17, 4) # Output: (4, 1)
As the curtains rise on this mathematical drama, 17 strides onto the platform as the resilient dividend, daringly paired with 4 in the role of the divisor. The divmod() symphony plays out with finesse, yielding a narrative where the quotient flourishes at 4, while the remainder valiantly asserts itself at 1.
Unlocking the Secrets of divmod() Insights:
- Diving Deeper into the Divisor-Dividend Duet: The divmod() function extends a hand to not only provide the remainder of a division but also to graciously offer the quotient, all in a single breathtaking sequence;
- Stepping into the Dividend’s Shoes:In the first act, the dividend takes the stage, donning the number 8. Dividing its attention between the poised divisor, 2, the divmod() function orchestrates an entrancing performance;
- Divisor Drama Unveiled:In the second act, the spotlight shifts, showcasing the resilient dividend of 17 alongside the daring divisor, 4. The divmod() function orchestrates a captivating display of division, revealing the nuanced interplay between quotient and remainder;
- The Art of the Tuple:Each divmod() incantation concludes with a dramatic flourish—a tuple composed of the quotient and the remainder. A tuple, in Python, is an immutable sequence that elegantly captures the essence of division’s dualities.
Sage Advice for the Aspiring Divmod Magician:
- Taming Complex Divisions:Harness the divmod() function’s prowess to dissect intricate divisions, unveiling both the quotient and remainder in a single, elegant swoop;
- Numerical Choreography:Experiment with diverse dividend-divisor pairs to uncover the subtle dance of numbers orchestrated by the divmod() function. Witness firsthand the harmonious partnership between quotient and remainder;
- Tuple Mastery:Embrace the world of tuples, the enchanting vessels that encapsulate divmod() revelations. Delve into tuple manipulation and exploration to deepen your understanding of this mystical duo;
- Expanding Horizons:Extend your exploration beyond the realms of simple arithmetic. Embrace divmod() as a tool for crafting efficient algorithms, optimizing code, and unlocking new perspectives in numerical problem-solving.
Method 3: Employing the math.fmod() Function for Determining Remainders in Python
In the fascinating realm of Python programming, uncovering remainders takes on a new dimension with the math.fmod() function. Nestled within the toolkit of the math module, this function presents an alternative avenue to the traditional % operator. However, what sets it apart is its remarkable adherence to the principles laid down by the venerable C programming language.
Eager to embark on this mathematical voyage? The first step involves ushering in the math module. Don’t worry; it’s a seamless process. Just import the math module into your code, and you’re ready to unveil the magic of math.fmod().
Let’s now dive into captivating examples that showcase the sheer versatility of math.fmod():
Rhythmic Ripples:
import math
remainder = math.fmod(5, 2)
# The remainder of 5 divided by 2 is 1.0
Harmonious Imbalance:
import math
remainder = math.fmod(11, 7)
# When 11 dances with 7, the remainder of their partnership is 4.0
Symphonic Division:
import math
remainder = math.fmod(13, 5)
# The harmony of 13 divided by 5 yields a captivating remainder of 3.0
As we delve into the intricacies of these examples, we begin to see the mathematical narrative unfold. In the first scenario, the stage is set with 5 as the dividend and 2 as the divisor. The enchanting math.fmod() function steps in to reveal a remainder of 1.0, adding a touch of elegance to this numerical dance.
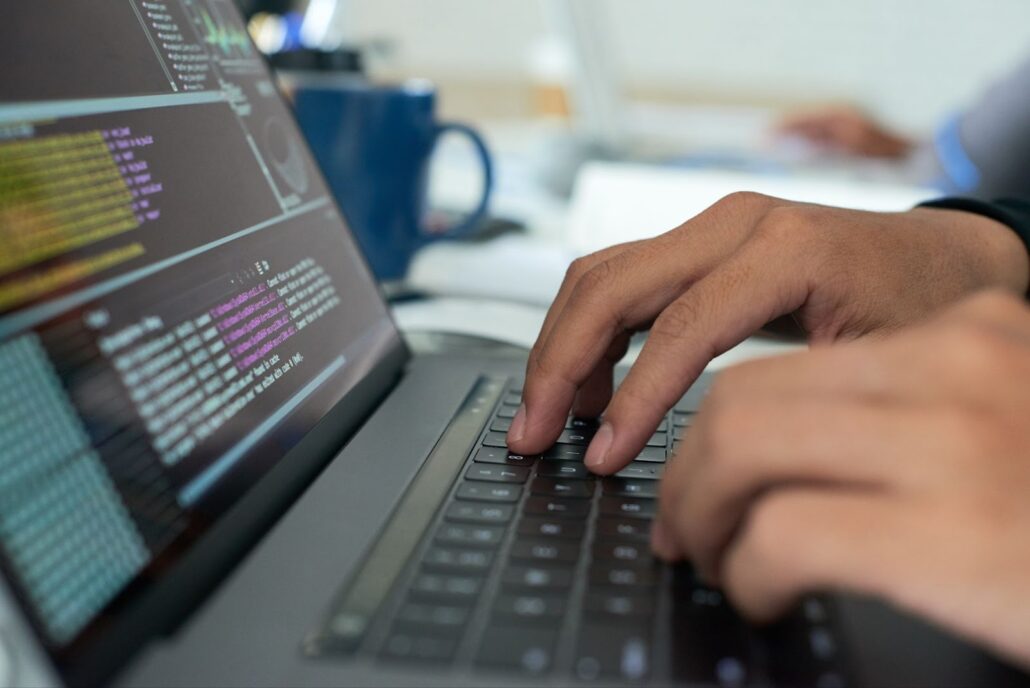
But what makes math.fmod() truly remarkable is its allegiance to the C programming legacy. Let’s not just scratch the surface; instead, let’s peel back the layers and explore why math.fmod() is the symphony conductor of remainder calculation.
Why Choose math.fmod() Over Traditional Operators?
- C-Style Resonance: Inspired by the principles of the C programming language, math.fmod() ensures compatibility and consistency with its time-honored roots;
- Floating-Point Flourish: Unlike its operator counterpart, math.fmod() embraces floating-point numbers with open arms, offering a broader range of application in your Pythonic orchestrations;
- Negative Serenade: When negative numbers make an appearance, math.fmod() deftly manages the melody, retaining their inherent negativity in the remainder calculation.
Conducting Your Own Symphony with math.fmod(): Tips and Tricks
- Diverse Data Duets: Experiment with various data types, ranging from integers to floating-point numbers, to witness the harmonious resonance that math.fmod() brings to each performance;
- Float into Precision: If precision is your muse, math.fmod() excels in handling decimal-heavy calculations, painting accurate pictures of remainders that transcend the ordinary;
- Modular Composition: Combine math.fmod() with other mathematical functions from the math module to compose intricate pieces of code that perform remarkable mathematical acrobatics.
Method 4: Unveiling Remainders with Python’s Remarkable remainder() Function
Introducing the captivating world of Python’s mathematical prowess! Among its arsenal of division-wrangling tools lies the exceptional remainder() method, a true marvel within the math module. Ever find yourself seeking the elusive remainder of a division operation? Look no further; the remainder() function is here to fulfill your mathematical yearnings. But hold on, this function isn’t just any old remainder calculator; it adheres to the prestigious IEEE 754 standards, ensuring precision and reliability in every numerical dance.
Unleashing the Power of remainder()
Before we dive into the mesmerizing world of remainders, a quick import is in order. Just like its sibling fmod() function, the remainder() method requires the math module to be summoned before its magical abilities can be harnessed. Once the math module has been beckoned, brace yourself for a symphony of mathematical excellence. Let’s illuminate the path with a few enlightening examples:
import math
# Calculating the remainder of 8 divided by 3
result_1 = math.remainder(8, 3) # Output: 2.0
# Discovering the remainder of 13 divided by 5
result_2 = math.remainder(13, 5) # Output: 4.0
# Unraveling the mystery behind 11 divided by 3
result_3 = math.remainder(11, 3) # Output: 2.0
In the third example, where 11 is the brave dividend and 3 is the noble divisor, the remainder() function elegantly unveils the answer: the remainder is 2, a tidbit of mathematical magic that’s crucial to various problem-solving scenarios.
Unmasking the IEEE 754 Standard
But what sets the remainder() function apart from its counterparts? A splendid question! While both remainder() and fmod() might appear akin, our champion adheres to the regal IEEE 754 standards with unwavering loyalty. Imagine a world where numerical precision is paramount, where consistency reigns supreme, and calculations are executed with impeccable accuracy. That’s the realm where the remainder() function thrives.
Gems of Wisdom: Practical Insights and Recommendations
Embracing the remainder() function opens doors to a realm of possibilities. Here’s a treasure trove of insights and recommendations to make the most of this mathematical gem:
- Harmony with IEEE 754: When precision is paramount, and adherence to the IEEE 754 standard is a must, remainder() shines brightest. Its commitment to exactness ensures results that can be trusted with unwavering confidence;
- Numerical Elegance: From calculating periodic cycles in data analysis to deciphering time intervals in your code, the remainder() function becomes your partner in unraveling numerical elegance;
- Divide and Conquer: When dividing the world into smaller, manageable pieces, the remainder() function lends itself to tasks like evenly distributing resources, balancing workloads, or partitioning data;
- The Gateway to Creativity: Think beyond arithmetic! Combine the remainder() function with other Python tools to create artful algorithms, intriguing puzzles, or simulations that push the boundaries of imagination;
- Smooth Sailing: By importing the math module and welcoming the remainder() function into your codebase, you unlock a world of mathematical possibilities. Harness its power to streamline calculations and make your code more efficient.
Conclusion
To sum up, the process of determining the remainder becomes effortlessly clear and direct through the utilization of the % operator, rendering it a widely embraced choice for this particular undertaking. A tuple yielded by the divmod() function encapsulates both the quotient and remainder resulting from a division operation. Within the math module, we encounter both the fmod() and remainder() functions; nonetheless, the former abides by the regulations of the C programming language, whereas the latter conforms to the standards set by IEEE 754.
The previously mentioned techniques stand out as excellent methodologies for deducing the remainder in the context of a divisional calculation. The specific approach you opt for hinges upon your distinct requisites and predilections for the project you are embarking upon.