Mastering Django requires overcoming various challenges, and one common stumbling block is the “init() missing 1 required positional argument: ‘on_delete'” error. This guide will provide you with insights into why this error surfaces and equip you with practical solutions to conquer it.
Understanding the Core Issue: “on_delete” Argument
The “init() missing 1 required positional argument: ‘on_delete'” error is rooted in Django’s foreign key declarations. In Django 2.0, the “init()” method demands the “on_delete” argument to dictate how related objects behave when their referenced counterpart is deleted. This error emerges when this pivotal argument is overlooked.
Exploring the Context: Error Causes and Scenarios
The “init()” error manifests due to non-compliance with Django’s stringent regulations. Neglecting to specify the “on_delete” argument in a foreign key declaration triggers this error. This applies to diverse relationships, including Many-to-One, Many-to-Many, and One-to-One.
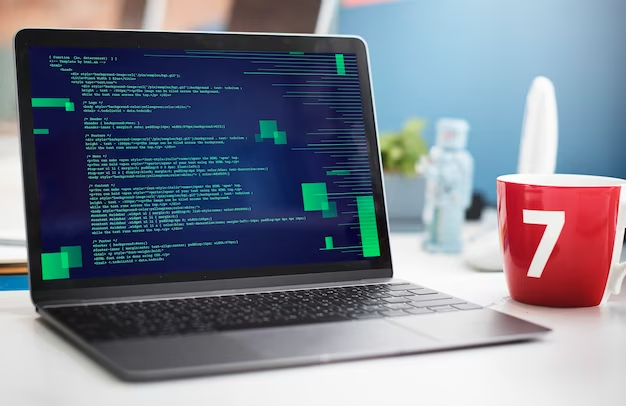
Solutions: Taming the “init() missing 1 required positional argument: ‘on_delete'” Beast
Overcoming this error demands meticulous steps to rectify the issue. Here’s a comprehensive approach to conquering the “init()” error:
- Identifying the Offender: Locate the Model and Field
The error message itself is a breadcrumb trail leading to the problematic model and field. For instance:
File "Path", line 11, in Album artist = models.ForeignKey(Artist) TypeError: ForeignKey.__init__() missing 1 required positional argument:
Here, the “artist” field within the “Album” model is at fault.
- Choosing the Right “on_delete” Option: Informed Decision-Making
Django presents seven distinct “on_delete” options: CASCADE, RESTRICT, PROTECT, SET_DEFAULT, SET_NULL, SET(), and DO_NOTHING. Your choice should align with your application’s dynamics. CASCADE, which deletes related objects when their referenced entity is deleted, is often a suitable pick.
- Applying the Solution: Embedding “on_delete” in Your Field
Embedding the chosen “on_delete” option into the problematic field’s declaration is the final step. Modify the field declaration in your model, as shown:
class Album(models.Model): name = models.CharField(max_length=30) artist = models.ForeignKey(Artist, on_delete=models.CASCADE)
By providing the requisite argument, you ensure Django’s expectations are met, quashing the error.
Table of Possible Methods
This table summarizes different methods for addressing the “init() missing 1 required positional argument: ‘on_delete'” issue in Django, providing an overview of each method’s purpose and impact.
Method | Description |
---|---|
Manually Adding on_delete | Explicitly provide the on_delete argument in the ForeignKey field declaration. |
Using CASCADE Option | Set the on_delete argument to CASCADE, automatically deleting related objects. |
Employing RESTRICT Option | Use the on_delete argument as RESTRICT to prevent deletion if related objects exist. |
Applying SET_NULL Option | Specify on_delete as SET_NULL to set foreign key references to NULL on deletion. |
Implementing SET_DEFAULT Option | Set on_delete to SET_DEFAULT to use a default value for foreign key references on deletion. |
Utilizing PROTECT Option | Use PROTECT for on_delete to raise a ProtectedError if related objects exist on deletion. |
Employing SET() Option | Specify on_delete as SET() to call a custom function when related objects are deleted. |
Implementing DO_NOTHING Option | Use DO_NOTHING for on_delete to leave the related objects untouched on deletion. |
Conclusion
Django’s “init() missing 1 required positional argument: ‘on_delete'” error might initially perplex you, but armed with this guide’s wisdom, you can confidently diagnose its source and apply remedies. Abiding by Django’s conventions is paramount for a seamless and error-free development journey.
FAQ
This error occurs in Django 2.0 when you fail to provide the required ‘on_delete’ argument while declaring a foreign key. It signals that referenced objects are left without a defined action when related objects are deleted.
The error message itself holds the key. It indicates the problematic model and field, guiding you directly to the source of the problem.
In Django 2.0, the ‘on_delete’ argument is mandatory for foreign keys. It specifies what should happen to an object referencing other objects when the referenced objects are deleted.
No, even if you don’t require a specific action, you must still provide the ‘on_delete’ argument with an appropriate option. Django doesn’t allow omitting it.